有UI 的版本
import { useState, useEffect } from 'react';
function CountdownTimer({ targetTime }) {
const [timeDiff, setTimeDiff] = useState(targetTime - Date.now());
useEffect(() => {
const timerId = setInterval(() => {
const newTimeDiff = targetTime - Date.now();
setTimeDiff(newTimeDiff);
if (newTimeDiff <= 0) {
clearInterval(timerId);
}
}, 10);
return () => clearInterval(timerId);
}, [targetTime]);
const hours = Math.floor(timeDiff / (1000 * 60 * 60));
const minutes = Math.floor((timeDiff % (1000 * 60 * 60)) / (1000 * 60));
const seconds = Math.floor((timeDiff % (1000 * 60)) / 1000);
const milliseconds = timeDiff % 1000;
return (
<div>
<span>{hours.toString().padStart(2, '0')}</span>:
<span>{minutes.toString().padStart(2, '0')}</span>:
<span>{seconds.toString().padStart(2, '0')}</span>.
<span>{milliseconds.toString().padStart(3, '0')}</span>
</div>
);
}
export default CountdownTimer;
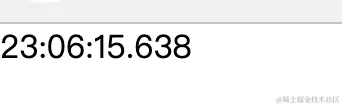
再增加一个hook 版本:
import { useState, useEffect } from 'react';
const useCountdownTimer = (initialSeconds) => {
const [time, setTime] = useState(initialSeconds * 1000);
useEffect(() => {
const countdown = setInterval(() => {
setTime((prevTime) => (prevTime > 0 ? prevTime - 10 : 0));
}, 10);
return () => clearInterval(countdown);
}, []);
return {
milliseconds: time,
seconds: Math.floor(time / 1000),
minutes: Math.floor(time / (1000 * 60)),
formatted: `${Math.floor(time / (1000 * 60))}:${((time % 60000) / 1000).toFixed(3)}`,
};
};
export default useCountdownTimer;
使用就方便了
const { milliseconds, seconds, minutes, formatted } = useCountdownTimer(600);