3.js原型和原型链
class Student {
constructor(name, number) {
this.name = name
this.number = number
this.gender = 'nale'
}
sayHi() {
console.log(`姓名${this.name}, 学号${this.number}`)
}
}
const xiaoluo = new Student('夏洛', 100)
console.log(xiaoluo.name)
console.log(xiaoluo.number)
xiaoluo.sayHi()
const madongmei = new Student('马冬梅', 101)
console.log(madongmei.name)
console.log(madongmei.number)
madongmei.sayHi()
class People {
constructor(name) {
this.name = name
}
eat() {
console.log(`${this.name} eat something`)
}
}
class Student extends People {
constructor(name, number) {
super(name)
this.number = number
}
sayHi() {
console.log(`姓名${this.name}, 学号${this.number}`)
}
}
class Teacher extends People {
constructor(name, major) {
super(name)
this.major = major
}
teach() {
console.log(`姓名${this.name}, 学号${this.major}`)
}
}
const xiaoluo = new Student('夏洛', 100)
console.log(xiaoluo.name)
console.log(xiaoluo.number)
xiaoluo.sayHi()
xiaoluo.eat()
const wanglaoshi = new Teacher('王老师', '语文')
console.log(wanglaoshi.name)
console.log(wanglaoshi.major)
wanglaoshi.teach()
wanglaoshi.eat()
xiaoluo instanceof Student
xiaoluo instanceof People
xiaoluo instanceof Object
[] instanceof Array
[] instanceof Object
{} instanceof Object
typeof People
typeof Student
console.log(xiaoluo.__proto__)
console.log(Student.prototype)
console.log(xiaoluo.__proto__ === Student.prototype)
每个class都有显示原型prototype
每个实例都有隐式原型__proto__
实例的__proto__指向对应class的prototype
获取属性xiaoluo.name或执行方法xialuo.sayHi()时
先在自身属性和方法寻找
如果找不到则自动去__proto__中查找
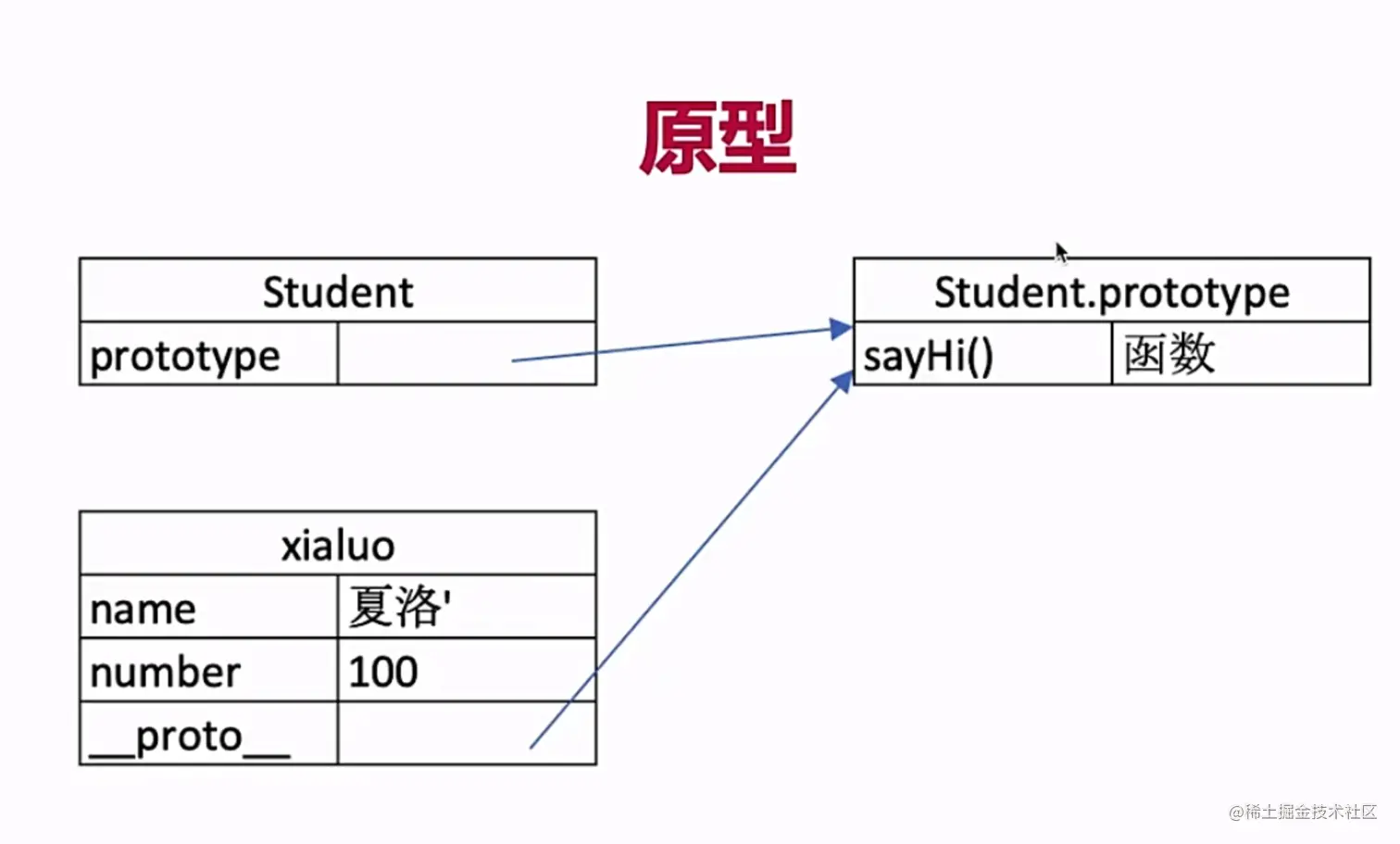
console.log(Student.prototype.__proto__)
console.log(People.prototype)
console.log(People.prototype === Student.prototype.__proto__)
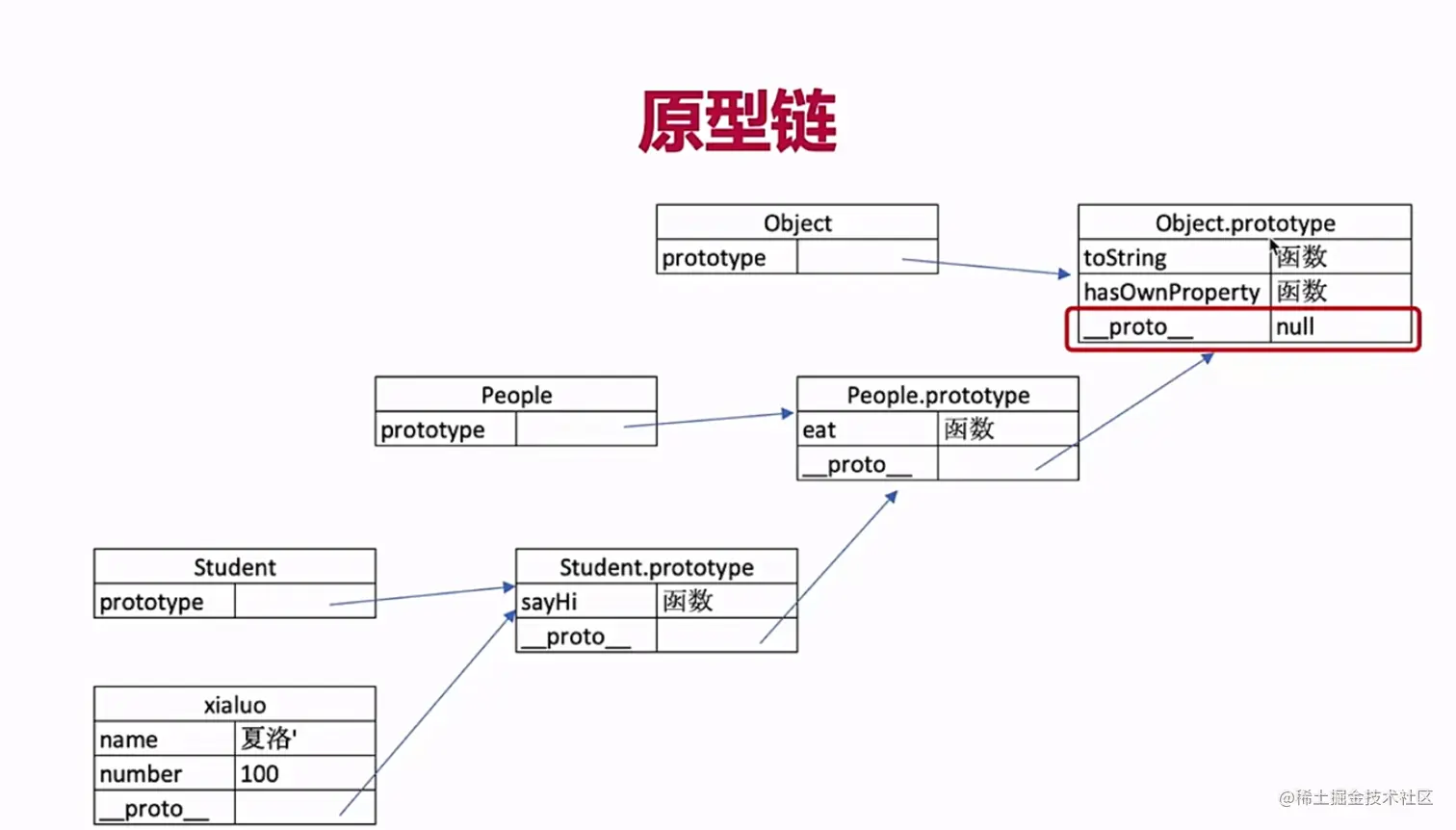
instanceof原理
instanceof原理
1.获取实例对象的隐式原型
2.获取构造函数的prototype属性
3.while 循环 -> 在原型链上不断向上查找
4.在原型链上不断查找 构造函数的显式原型
5.直到implicitPrototype = null 都没有找到,返回false
6.构造函数的prototype属性出现在实例对象的原型链上返回true
定义:instanceof运算符用于检测构造函数的prototype属性是否出现某个实例对象的原型链上
function instance_of(Obj, Constructor) {
let implicitPrototype = Obj.__proto__
let displayPrototype = Constructor.prototype
while(true) {
if (implicitPrototype === null) {
return false
} else if (implicitPrototype === displayPrototype) {
return true
}
implicitPrototype = implicitPrototype.__proto__
}
}