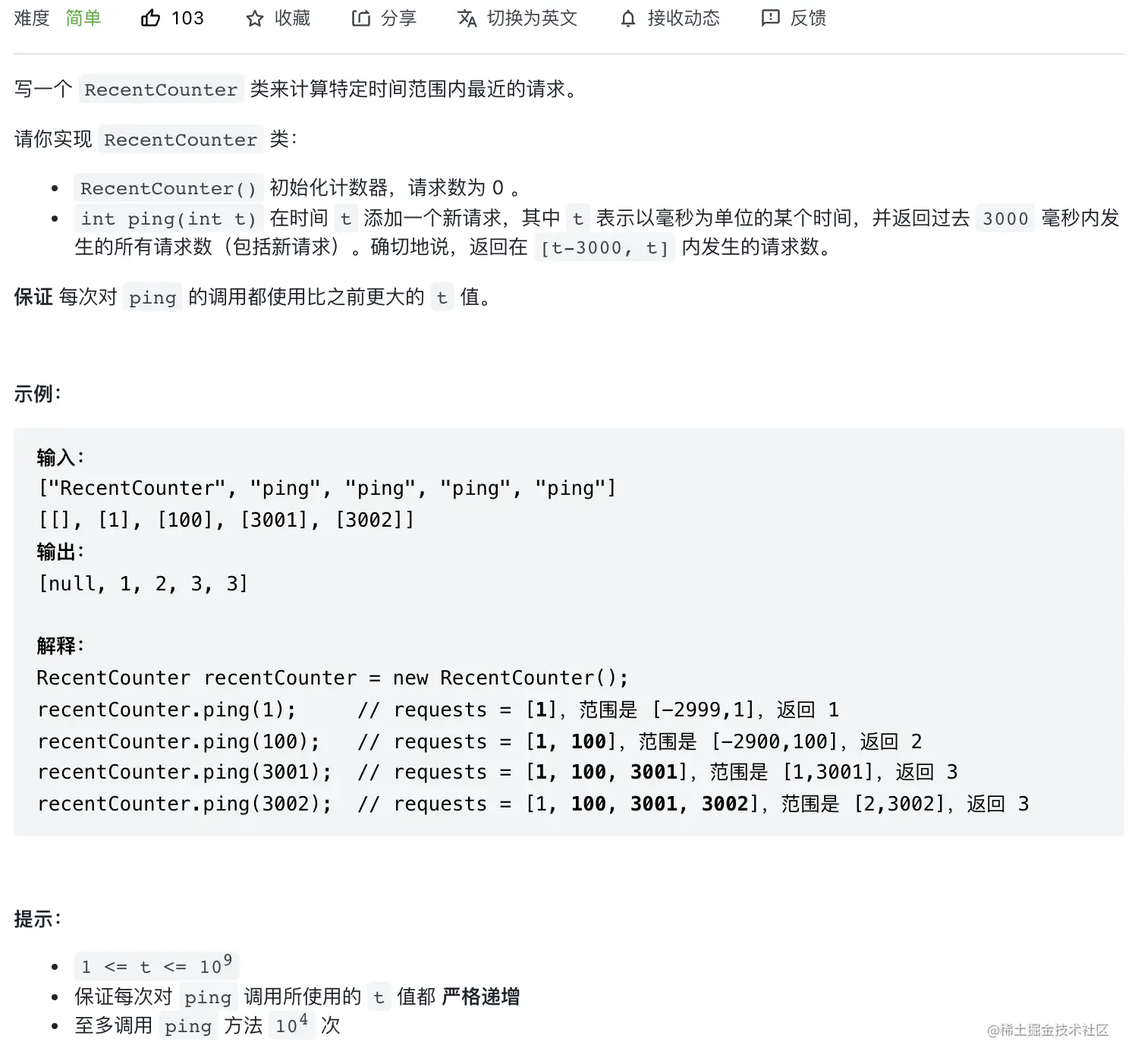
解题思路
- 小于 t-3000 的请求 都是不符合要求的请求
- 在循环中,都需要shift掉
var RecentCounter = function() {
this.queue =[];
};
RecentCounter.prototype.ping = function(t) {
this.queue.push(t);
while(this.queue[0] < t - 3000) {
this.queue.shift();
}
return this.queue.length;
};
解法二
var RecentCounter = function() {
this.queue = [];
this.cur = 0;
};
RecentCounter.prototype.ping = function(t) {
this.queue.push(t);
while(this.queue[this.cur] < t - 3000) {
this.cur++
}
return this.queue.length - this.cur;
};
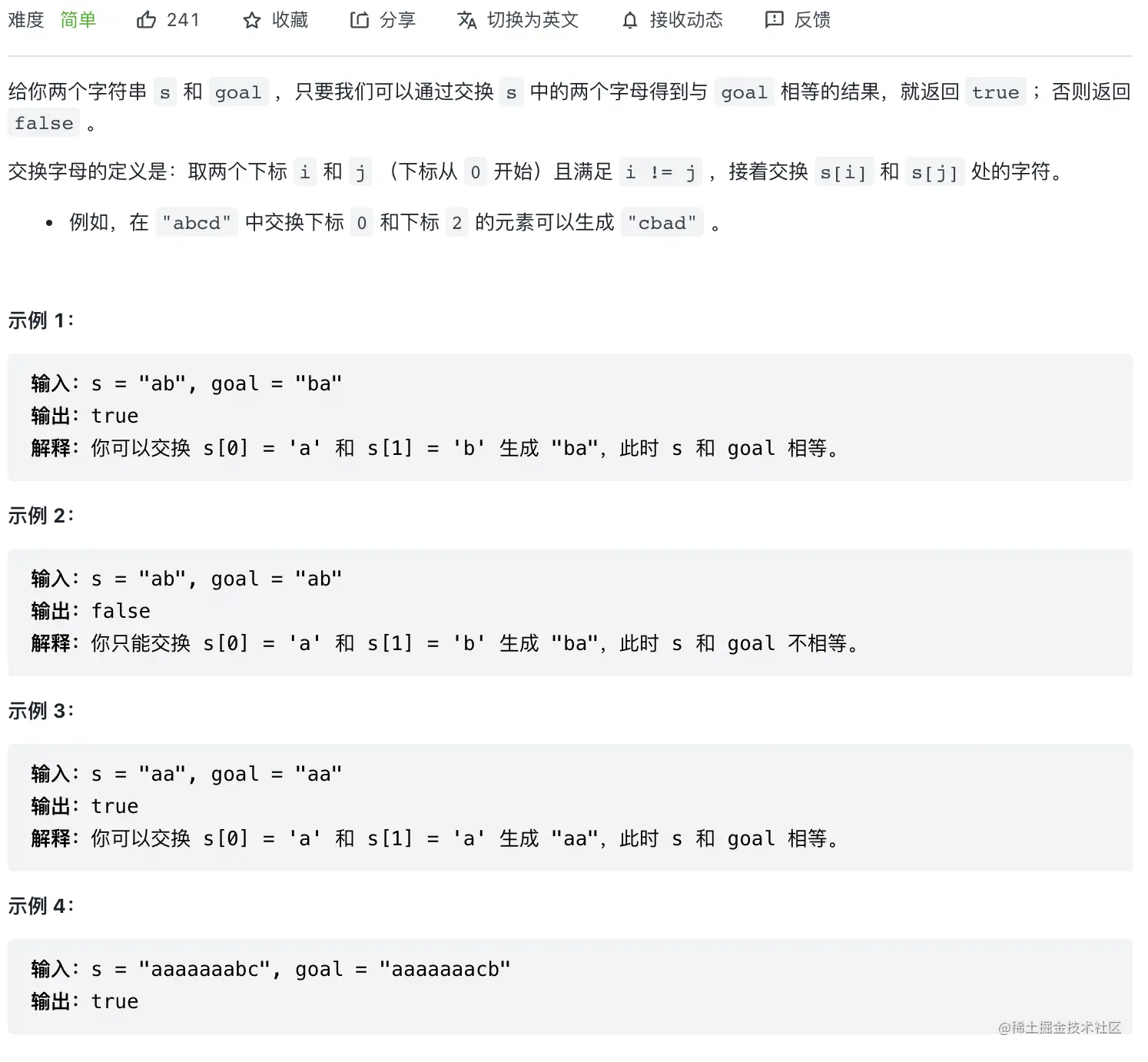
var buddyStrings = function(s, goal) {
let queue = [];
if(s?.length !== goal?.length) {
return false;
}
if(s === goal) {
return s.length > new Set(s).size;
}
for(let i=0; i < s.length; i++) {
if(s[i] !== goal[i] ) {
queue.push(s[i],goal[i]);
}
}
if(queue.length > 4) {
return false;
}
if(queue[0] === queue[3] && queue[1] === queue[2]) {
return true;
} else {
return false;
}
};
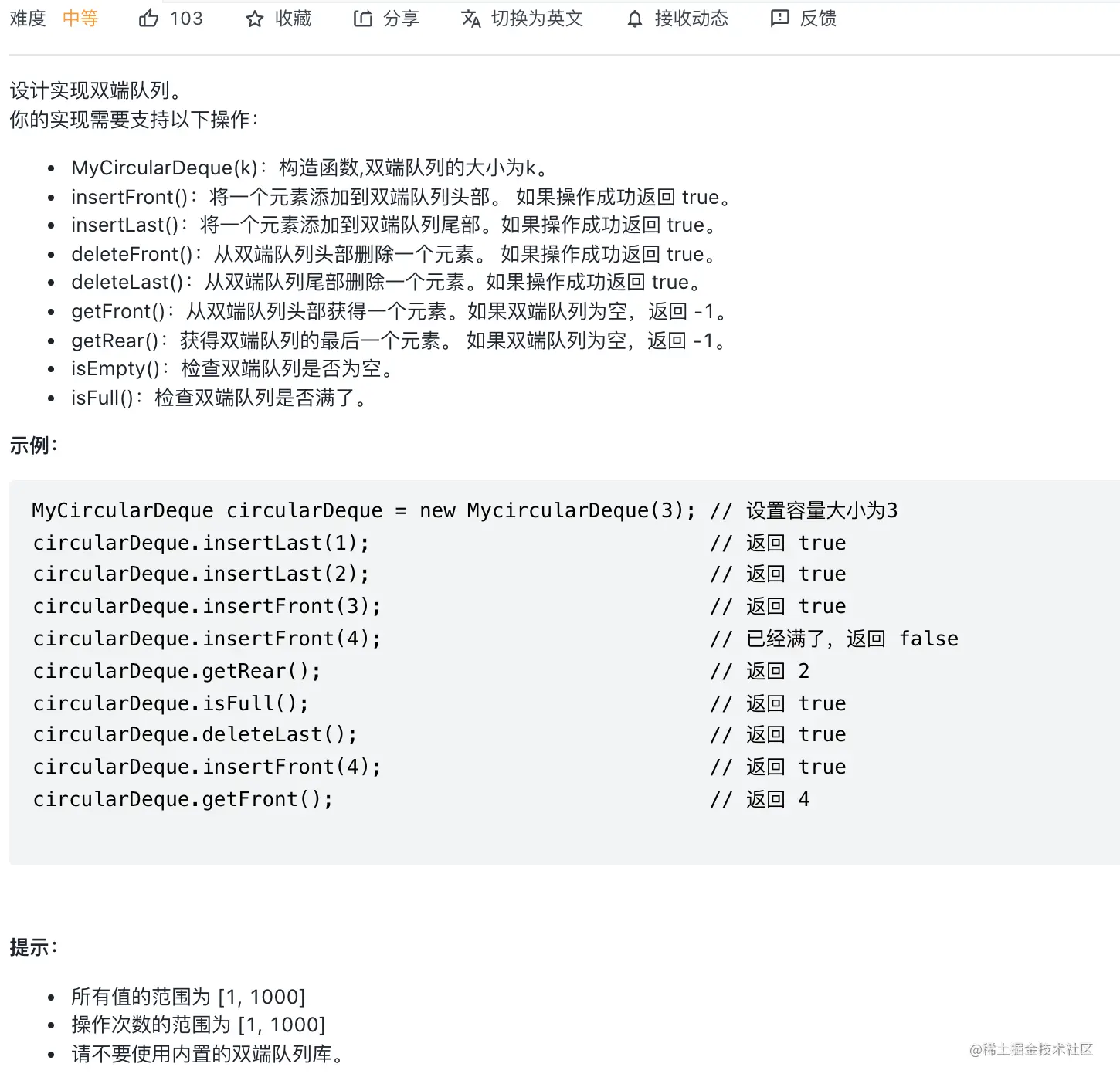
var MyCircularDeque = function(k) {
this.queue = new Array(k)
this.max = k;
this.count = 0;
this.head = 0;
this.tail = 1;
};
MyCircularDeque.prototype.insertFront = function(value) {
if(this.isFull()) {
return false;
}
this.queue[this.head] = value;
this.head = (this.head -1 +this.max)%this.max;
this.count++;
return true;
};
MyCircularDeque.prototype.insertLast = function(value) {
if(this.isFull()) {
return false;
}
this.queue[this.tail] = value;
this.tail = (this.tail + 1) % this.max;
this.count++;
return true;
};
MyCircularDeque.prototype.deleteFront = function() {
if(this.isEmpty()) {
return false;
}
this.head = (this.head + 1) % this.max;
this.count--;
return true;
};
MyCircularDeque.prototype.deleteLast = function() {
if(this.isEmpty()) {
return false;
}
this.tail = (this.tail -1 + this.max)% this.max;
this.count--;
return true;
};
MyCircularDeque.prototype.getFront = function() {
if(this.isEmpty()) {
return -1;
}
return this.queue[(this.head + 1) % this.max];
};
MyCircularDeque.prototype.getRear = function() {
if(this.isEmpty()) {
return -1;
}
return this.queue[(this.tail -1 + this.max)% this.max];
};
MyCircularDeque.prototype.isEmpty = function() {
return this.count === 0;
};
MyCircularDeque.prototype.isFull = function() {
return this.count === this.max;
};
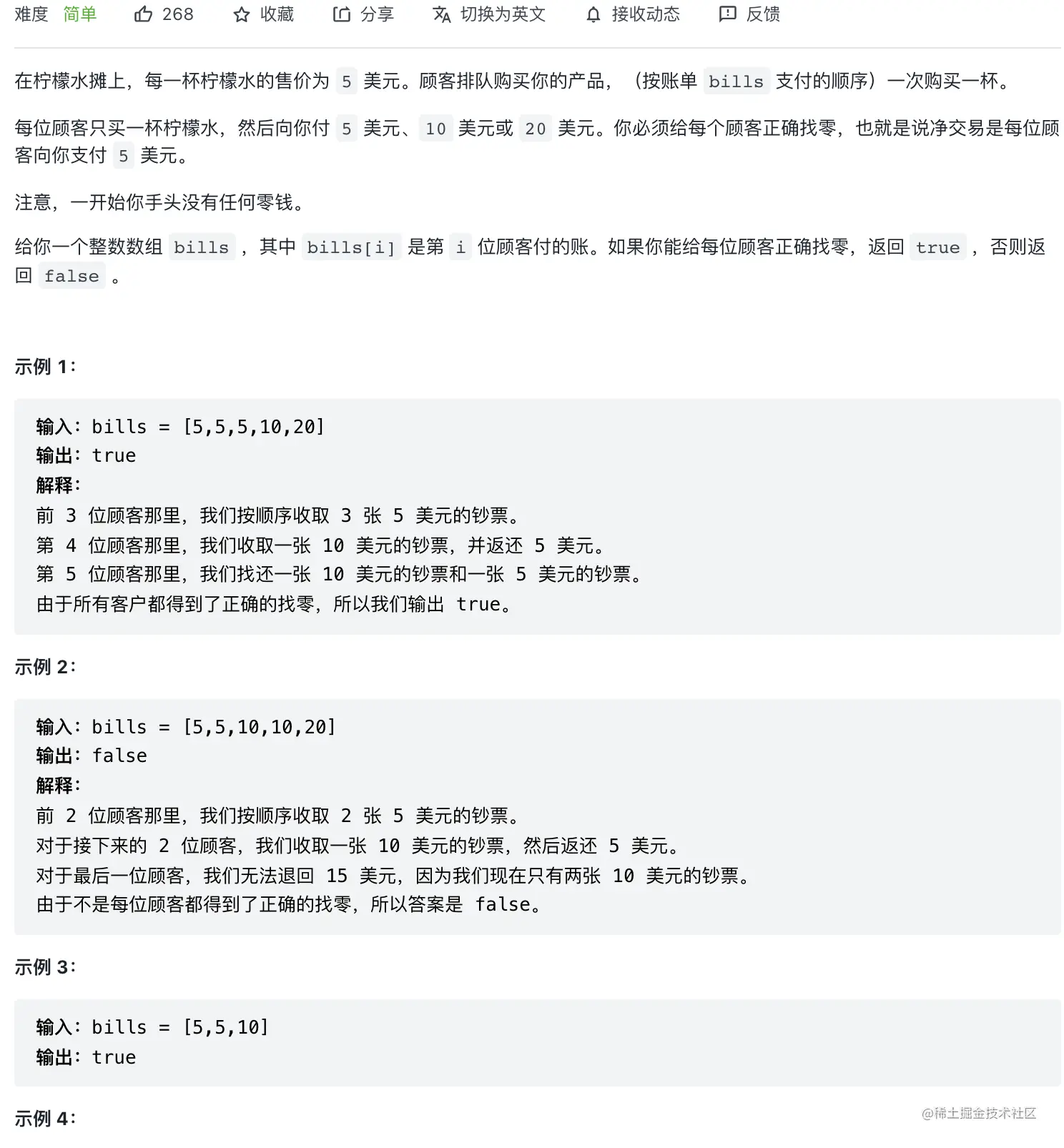
var lemonadeChange = function(bills) {
let result = true;
const billMap = new Map();
billMap.set(5,0)
billMap.set(10,0)
billMap.set(20,0)
const handleTrade = (bill) => {
let num = billMap.get(bill) || 0;
switch(bill) {
case 5:
billMap.set(5, num + 1 );
break;
case 10:
if(billMap.get(5) <= 0) {
return false
}
billMap.set(10, num + 1);
billMap.set(5, billMap.get(5) - 1);
break;
case 20:
if(billMap.get(10) >= 1) {
if(billMap.get(5) < 1) {
return false;
}
billMap.set(10,billMap.get(10) - 1)
billMap.set(5, billMap.get(5) - 1)
billMap.set(20, num + 1);
} else {
if(billMap.get(5) < 3){
return false;
}
billMap.set(5, billMap.get(5) - 3);
billMap.set(20, num + 1);
}
break;
}
}
for(let i = 0;i < bills.length; i++) {
const bill = bills[i];
if(handleTrade(bill) === false) {
return false;
}
}
return result;
};