部分成员函数
节点结构
struct Node {
char val;
Node* left;
Node* right;
Node(char ch) {
val = ch;
left = right = nullptr;
}
};
先序+中序创建二叉树
//根据先序遍历和中序遍历进行创建二叉树
void PreOrderCreate() {
string preorder;
string midorder;
// cout << "please input preorder and midorder:" << endl;
// cin >> preorder >> midorder;
// cout << preorder << ":" << midorder << endl;
preorder = "ABDEHJCFGK";
midorder = "DBHJEAFGKC";
int len = preorder.size();
if (len != midorder.size()){
cout << "error input." << endl;
return;
}
PreOrderCreateCore(root, &preorder[0], &midorder[0], len);
}
void PreOrderCreateCore(Node*& curNode, const char* pre, const char* mid, int len) {
int i = 0;
if (len < 1) return;
curNode = new Node(*pre);
int n = 0;
while (mid[n] != pre[0]) {
++n;
}
PreOrderCreateCore(curNode->left, pre + 1, mid, n);
PreOrderCreateCore(curNode->right, pre + n + 1, mid + n + 1, len - n - 1);
}
后序+中序创建二叉树
//根据后序遍历和中序遍历进行创建二叉树
void PostOrderCreate() {
cout << "please input postorder and midorder." << endl;
string postorder;
string midorder;
cin >> postorder >> midorder;
int len = midorder.length();
if (len != postorder.length()) {
cout << "error input";
return;
}
PostOrderCreateCore(root, &postorder[0], &midorder[0], len);
}
void PostOrderCreateCore(Node*& curNode, const char* post, const char* mid, int len) {
if (len < 1) return;
curNode = new Node(post[len - 1]);
int n = 0;
while (mid[n] != post[len - 1]) {
++n;
}
PostOrderCreateCore(curNode->left, post, mid, n);
PostOrderCreateCore(curNode->right, post + n, mid + n + 1, len - n - 1);
}
先序遍历(递归+非递归)
//先序遍历(递归遍历)
void PreOrderTraverseByRecursion(Node* root) {
if (!root) return;
cout << root->val << " ";
PreOrderTraverseByRecursion(root->left);
PreOrderTraverseByRecursion(root->right);
}
//先序遍历(使用栈遍历)
void PreOrderTraverseByStack(Node* root) {
stack<Node*> nodeStack;
Node* p = root;
while (1) {
while (p) {
cout << p->val << " ";
if (p->right) {
nodeStack.push(p->right);
}
p = p->left;
}
if (!nodeStack.empty()) {
p = nodeStack.top();
nodeStack.pop();
}
else {
break;
}
}
}
中序遍历(递归+非递归)
//中序遍历(递归遍历)
void MidOrderTraverseByRecursion(Node* root) {
if (!root) return;
MidOrderTraverseByRecursion(root->left);
cout << root->val << " ";
MidOrderTraverseByRecursion(root->right);
}
//中序遍历(使用栈遍历)
void MidOrderTraverseByStack(Node* root) {
if (!root) return;
stack<Node*> nodeStack;
Node* p = root;
while (p || !nodeStack.empty()) {
while (p) { //关键要确保这个循环的节点一定是第一次访问的,所以采取所有点都压入栈中
nodeStack.push(p);
p = p->left;
}
p = nodeStack.top(); //p只指向栈中点的右节点,避免再次访问该点的左节点;
nodeStack.pop();
cout << p->val <<" ";
p = p->right;
}
}
后序遍历(递归+非递归)
//后序遍历(递归遍历)
void PostOrderTraverseByRecursion(Node* root) {
if (!root) return;
PostOrderTraverseByRecursion(root->left);
PostOrderTraverseByRecursion(root->right);
cout << root->val << " ";
}
//后序遍历(使用栈遍历)
void PostOrderTraverseByStack(Node* root) {
if (!root) return;
stack<Node*> nodeStack;
nodeStack.push(root);
Node* lastPos = nullptr;
while (!nodeStack.empty()) {
while (nodeStack.top()->left) {
nodeStack.push(nodeStack.top()->left);
}
while (!nodeStack.empty()) {
if (nodeStack.top()->right == nullptr || nodeStack.top()->right == lastPos){
cout << nodeStack.top()->val << " ";
lastPos = nodeStack.top();
nodeStack.pop();
}else if (nodeStack.top()->right) {
nodeStack.push(nodeStack.top()->right);
break;
}
}
}
}
层次遍历
//层次遍历
void LevelOrder(Node* root) {
if (!root) return;
queue<Node*> nodeQueue;
nodeQueue.push(root);
Node* p = nullptr;
while(!nodeQueue.empty()) {
p = nodeQueue.front();
nodeQueue.pop();
cout << p->val << " ";
if (p->left) nodeQueue.push(p->left);
if (p->right) nodeQueue.push(p->right);
}
}
树的高度(递归+非递归)
//获取二叉树的高度(递归 + 队列)
int GetHeightByRecursion(Node* root){
if (!root) return 0;
return GetHeightByRecursion(root->left) > GetHeightByRecursion(root->right) ?
GetHeightByRecursion(root->left) + 1 : GetHeightByRecursion(root->right) + 1;
}
int GetHeightByQueue(Node* root) {
if (!root) return 0;
int Height = 1;
queue<Node*> nodeQueue;
nodeQueue.push(root);
/* 记录最后一个节点的形式
Node* lastNodeInLayer = root;
Node* p = nullptr;
while (!nodeQueue.empty()) {
if (nodeQueue.front()->left) {
nodeQueue.push(nodeQueue.front()->left);
}
if (nodeQueue.front()->right) {
nodeQueue.push(nodeQueue.front()->right);
}
p = nodeQueue.front();
nodeQueue.pop();
if (p == lastNodeInLayer && !nodeQueue.empty()) { //pop出的点是上一层最后一个点且queue仍然有数据的话,则层数+1
++Height;
lastNodeInLayer = nodeQueue.back();
}
}
*/
//层次遍历的方式
Height = 0;
while (!nodeQueue.empty()){
Height++;
int width = nodeQueue.size();
for (int i = 0; i < width; i++) {
if (nodeQueue.front()->left) {
nodeQueue.push(nodeQueue.front()->left);
}
if (nodeQueue.front()->right) {
nodeQueue.push(nodeQueue.front()->right);
}
nodeQueue.pop();
}
}
return Height;
}
树的宽度
//获取二叉树的最大宽度
int GetWidth(Node* root) {
if (!root) return 0;
queue<Node*> nodeQueue;
nodeQueue.push(root);
int maxWidth = 1;
int curWidth = 0;
while (!nodeQueue.empty()) {
int curWidth = nodeQueue.size();
if (curWidth > maxWidth) {
maxWidth = curWidth;
}
for (int i = 0; i < curWidth; i++) {
Node* p = nodeQueue.front();
nodeQueue.pop();
if(p->left) nodeQueue.push(p->left);
if (p->right) nodeQueue.push(p->right);
}
}
return maxWidth;
}
树的节点数(递归+非递归)
//获取二叉树节点数量
//非递归,任选一种遍历方法即可获取
int GetNodesNumByStack(Node* root) {
stack<Node*> nodeStack;
Node* p = root;
int nodesNum = 0;
while (1) {
while (p) {
++nodesNum;
if (p->right) {
nodeStack.push(p->right);
}
p = p->left;
}
if (!nodeStack.empty()) {
p = nodeStack.top();
nodeStack.pop();
}
else {
break;
}
}
return nodesNum;
}
int GetNodesNumByRecursion(Node* root) {
if (!root) return 0;
return 1 + GetNodesNumByRecursion(root->left) + GetNodesNumByRecursion(root->right);
}
根据值获取某个点的指针
//使用先序遍历
Node* GetAimNodeByVal(Node* root, char ch) {
stack<Node*> nodeStack;
Node* p = root;
while (1) {
while (p) {
if (p->val == ch) return p;
if (p->right) {
nodeStack.push(p->right);
}
p = p->left;
}
if (!nodeStack.empty()) {
p = nodeStack.top();
nodeStack.pop();
}
else {
break;
}
}
return nullptr;
}
获取某个节点的父节点
//获取某个节点的父节点(递归)
Node* GetParentNodeByRecursion(Node* root, Node* sonNode) {
if (!root || !sonNode) return nullptr;
if (root->left == sonNode) return root;
if (root->right == sonNode) return root;
Node* left = GetParentNodeByRecursion(root->left, sonNode);
if (left) return left;
return GetParentNodeByRecursion(root->right, sonNode);
}
获取根节点到目标节点的完整路径,存入给定的栈中
//获取根节点到目标节点的完整路径
//这里使用了后续遍历,使得栈中保存的全是父节点
void GetFullPathToAimNode(Node* root, Node* aimNode, stack<Node*>& nodeStack) {
if (!root) return;
nodeStack.push(root);
if (root == aimNode) return;
Node* lastNode = nullptr;
while (!nodeStack.empty()) {
while (nodeStack.top()->left) {
nodeStack.push(nodeStack.top()->left);
if (nodeStack.top() == aimNode) return;//每次判断push进来的点是不是aimNode;
}
while (!nodeStack.empty()) {
if (!nodeStack.top()->right || nodeStack.top()->right == lastNode) {
lastNode = nodeStack.top();
nodeStack.pop();
}
else {
nodeStack.push(nodeStack.top()->right);
if (nodeStack.top() == aimNode) return; //每次判断push进来的点是不是aimNode;
break;
}
}
}
}
销毁二叉树
void Destroy(Node* root) {
if (!root) return;
Destroy(root->left);
Destroy(root->right);
cout << root->val << " ";
delete root;
}
判断两颗二叉树是否相等
static bool Equal(Node* treeA, Node* treeB) {
if (treeA == NULL&& treeB == NULL)
return true;
if (treeA != NULL && treeB != NULL && (treeA->val == treeB->val) && Equal(treeA->left, treeB->left) && Equal(treeA->right, treeB->right))
return true;
else
return false;
}
x 和 y的最近公共节点
//最近x,y的公共节点(比较好的方法)
Node* GetLastCommonAncestor(Node* root, Node* node1, Node* node2) {
if (!root || !node1 || !node2) return nullptr;
if (root == node1 || root == node2) return root;
Node* left = GetLastCommonAncestor(root->left, node1, node2);
Node* right = GetLastCommonAncestor(root->right, node1, node2);
if (left && right) return root;
if (!left) {
return right;
}else {
return left;
}
}
左右子树交换
//交换所有左右子树
void SwapLeftRight(Node* root) {
if (!root) return;
Node* p = root->left;
root->left = root->right;
root->right = p;
SwapLeftRight(root->left);
SwapLeftRight(root->right);
}
完整代码
BinaryTree.hpp
#pragma once
#include<iostream>
#include<string>
#include<stack>
#include<queue>
using namespace std;
struct Node {
char val;
Node* left;
Node* right;
Node(char ch) {
val = ch;
left = right = nullptr;
}
};
class BinaryTree {
Node* root;
public:
BinaryTree() {
root = nullptr;
}
static bool Equal(Node* treeA, Node* treeB) {
if (treeA == NULL&& treeB == NULL)
return true;
if (treeA != NULL && treeB != NULL && (treeA->val == treeB->val) && Equal(treeA->left, treeB->left) && Equal(treeA->right, treeB->right))
return true;
else
return false;
}
Node* GetRoot() {
return root;
}
void Destroy(Node* root) {
if (!root) return;
Destroy(root->left);
Destroy(root->right);
cout << root->val << " ";
delete root;
}
//根据先序遍历和中序遍历进行创建二叉树
void PreOrderCreate() {
string preorder;
string midorder;
// cout << "please input preorder and midorder:" << endl;
// cin >> preorder >> midorder;
// cout << preorder << ":" << midorder << endl;
preorder = "ABDEHJCFGK";
midorder = "DBHJEAFGKC";
int len = preorder.size();
if (len != midorder.size()){
cout << "error input." << endl;
return;
}
PreOrderCreateCore(root, &preorder[0], &midorder[0], len);
}
void PreOrderCreateCore(Node*& curNode, const char* pre, const char* mid, int len) {
int i = 0;
if (len < 1) return;
curNode = new Node(*pre);
int n = 0;
while (mid[n] != pre[0]) {
++n;
}
PreOrderCreateCore(curNode->left, pre + 1, mid, n);
PreOrderCreateCore(curNode->right, pre + n + 1, mid + n + 1, len - n - 1);
}
//根据后序遍历和中序遍历进行创建二叉树
void PostOrderCreate() {
cout << "please input postorder and midorder." << endl;
string postorder;
string midorder;
cin >> postorder >> midorder;
int len = midorder.length();
if (len != postorder.length()) {
cout << "error input";
return;
}
PostOrderCreateCore(root, &postorder[0], &midorder[0], len);
}
void PostOrderCreateCore(Node*& curNode, const char* post, const char* mid, int len) {
if (len < 1) return;
curNode = new Node(post[len - 1]);
int n = 0;
while (mid[n] != post[len - 1]) {
++n;
}
PostOrderCreateCore(curNode->left, post, mid, n);
PostOrderCreateCore(curNode->right, post + n, mid + n + 1, len - n - 1);
}
//先序遍历(递归遍历)
void PreOrderTraverseByRecursion(Node* root) {
if (!root) return;
cout << root->val << " ";
PreOrderTraverseByRecursion(root->left);
PreOrderTraverseByRecursion(root->right);
}
//先序遍历(使用栈遍历)
void PreOrderTraverseByStack(Node* root) {
stack<Node*> nodeStack;
Node* p = root;
while (1) {
while (p) {
cout << p->val << " ";
if (p->right) {
nodeStack.push(p->right);
}
p = p->left;
}
if (!nodeStack.empty()) {
p = nodeStack.top();
nodeStack.pop();
}
else {
break;
}
}
}
//中序遍历(递归遍历)
void MidOrderTraverseByRecursion(Node* root) {
if (!root) return;
MidOrderTraverseByRecursion(root->left);
cout << root->val << " ";
MidOrderTraverseByRecursion(root->right);
}
//中序遍历(使用栈遍历)
void MidOrderTraverseByStack(Node* root) {
if (!root) return;
stack<Node*> nodeStack;
Node* p = root;
while (p || !nodeStack.empty()) {
while (p) { //关键要确保这个循环的节点一定是第一次访问的,所以采取所有点都压入栈中
nodeStack.push(p);
p = p->left;
}
p = nodeStack.top(); //p只指向栈中点的右节点,避免再次访问该点的左节点;
nodeStack.pop();
cout << p->val <<" ";
p = p->right;
}
}
//后序遍历(递归遍历)
void PostOrderTraverseByRecursion(Node* root) {
if (!root) return;
PostOrderTraverseByRecursion(root->left);
PostOrderTraverseByRecursion(root->right);
cout << root->val << " ";
}
//后序遍历(使用栈遍历)
void PostOrderTraverseByStack(Node* root) {
if (!root) return;
stack<Node*> nodeStack;
nodeStack.push(root);
Node* lastPos = nullptr;
while (!nodeStack.empty()) {
while (nodeStack.top()->left) {
nodeStack.push(nodeStack.top()->left);
}
while (!nodeStack.empty()) {
if (nodeStack.top()->right == nullptr || nodeStack.top()->right == lastPos){
cout << nodeStack.top()->val << " ";
lastPos = nodeStack.top();
nodeStack.pop();
}else if (nodeStack.top()->right) {
nodeStack.push(nodeStack.top()->right);
break;
}
}
}
}
//层次遍历
void LevelOrder(Node* root) {
if (!root) return;
queue<Node*> nodeQueue;
nodeQueue.push(root);
Node* p = nullptr;
while(!nodeQueue.empty()) {
p = nodeQueue.front();
nodeQueue.pop();
cout << p->val << " ";
if (p->left) nodeQueue.push(p->left);
if (p->right) nodeQueue.push(p->right);
}
}
//获取二叉树的高度(递归 + 队列)
int GetHeightByRecursion(Node* root){
if (!root) return 0;
return GetHeightByRecursion(root->left) > GetHeightByRecursion(root->right) ?
GetHeightByRecursion(root->left) + 1 : GetHeightByRecursion(root->right) + 1;
}
int GetHeightByQueue(Node* root) {
if (!root) return 0;
int Height = 1;
queue<Node*> nodeQueue;
nodeQueue.push(root);
/* 记录最后一个节点的形式
Node* lastNodeInLayer = root;
Node* p = nullptr;
while (!nodeQueue.empty()) {
if (nodeQueue.front()->left) {
nodeQueue.push(nodeQueue.front()->left);
}
if (nodeQueue.front()->right) {
nodeQueue.push(nodeQueue.front()->right);
}
p = nodeQueue.front();
nodeQueue.pop();
if (p == lastNodeInLayer && !nodeQueue.empty()) { //pop出的点是上一层最后一个点且queue仍然有数据的话,则层数+1
++Height;
lastNodeInLayer = nodeQueue.back();
}
}
*/
//层次遍历的方式
Height = 0;
while (!nodeQueue.empty()){
Height++;
int width = nodeQueue.size();
for (int i = 0; i < width; i++) {
if (nodeQueue.front()->left) {
nodeQueue.push(nodeQueue.front()->left);
}
if (nodeQueue.front()->right) {
nodeQueue.push(nodeQueue.front()->right);
}
nodeQueue.pop();
}
}
return Height;
}
//获取二叉树的最大宽度
int GetWidth(Node* root) {
if (!root) return 0;
queue<Node*> nodeQueue;
nodeQueue.push(root);
int maxWidth = 1;
int curWidth = 0;
while (!nodeQueue.empty()) {
int curWidth = nodeQueue.size();
if (curWidth > maxWidth) {
maxWidth = curWidth;
}
for (int i = 0; i < curWidth; i++) {
Node* p = nodeQueue.front();
nodeQueue.pop();
if(p->left) nodeQueue.push(p->left);
if (p->right) nodeQueue.push(p->right);
}
}
return maxWidth;
}
//获取二叉树节点数量
//非递归,任选一种遍历方法即可获取
int GetNodesNumByStack(Node* root) {
stack<Node*> nodeStack;
Node* p = root;
int nodesNum = 0;
while (1) {
while (p) {
++nodesNum;
if (p->right) {
nodeStack.push(p->right);
}
p = p->left;
}
if (!nodeStack.empty()) {
p = nodeStack.top();
nodeStack.pop();
}
else {
break;
}
}
return nodesNum;
}
int GetNodesNumByRecursion(Node* root) {
if (!root) return 0;
return 1 + GetNodesNumByRecursion(root->left) + GetNodesNumByRecursion(root->right);
}
//根据值获取某个点的指针
//使用先序遍历
Node* GetAimNodeByVal(Node* root, char ch) {
stack<Node*> nodeStack;
Node* p = root;
while (1) {
while (p) {
if (p->val == ch) return p;
if (p->right) {
nodeStack.push(p->right);
}
p = p->left;
}
if (!nodeStack.empty()) {
p = nodeStack.top();
nodeStack.pop();
}
else {
break;
}
}
return nullptr;
}
//获取某个节点的父节点(递归)
Node* GetParentNodeByRecursion(Node* root, Node* sonNode) {
if (!root || !sonNode) return nullptr;
if (root->left == sonNode) return root;
if (root->right == sonNode) return root;
Node* left = GetParentNodeByRecursion(root->left, sonNode);
if (left) return left;
return GetParentNodeByRecursion(root->right, sonNode);
}
//获取根节点到目标节点的完整路径
//这里使用了后续遍历,使得栈中保存的全是父节点
void GetFullPathToAimNode(Node* root, Node* aimNode, stack<Node*>& nodeStack) {
if (!root) return;
nodeStack.push(root);
if (root == aimNode) return;
Node* lastNode = nullptr;
while (!nodeStack.empty()) {
while (nodeStack.top()->left) {
nodeStack.push(nodeStack.top()->left);
if (nodeStack.top() == aimNode) return;//每次判断push进来的点是不是aimNode;
}
while (!nodeStack.empty()) {
if (!nodeStack.top()->right || nodeStack.top()->right == lastNode) {
lastNode = nodeStack.top();
nodeStack.pop();
}
else {
nodeStack.push(nodeStack.top()->right);
if (nodeStack.top() == aimNode) return; //每次判断push进来的点是不是aimNode;
break;
}
}
}
}
//最近x,y的公共节点(比较好的方法)
Node* GetLastCommonAncestor(Node* root, Node* node1, Node* node2) {
if (!root || !node1 || !node2) return nullptr;
if (root == node1 || root == node2) return root;
Node* left = GetLastCommonAncestor(root->left, node1, node2);
Node* right = GetLastCommonAncestor(root->right, node1, node2);
if (left && right) return root;
if (!left) {
return right;
}else {
return left;
}
}
//交换所有左右子树
void SwapLeftRight(Node* root) {
if (!root) return;
Node* p = root->left;
root->left = root->right;
root->right = p;
SwapLeftRight(root->left);
SwapLeftRight(root->right);
}
};
测试代码及结果
/* 测试用的二叉树
-
preorder = "ABDEHJCFGK";
-
midorder = "DBHJEAFGKC"; A / \ B C / \ / D E F / \ H G \ \ J K
*/
测试代码(main.cpp)
#include "BinaryTree.hpp"
/*
* preorder = "ABDEHJCFGK";
* midorder = "DBHJEAFGKC";
A
/ \
B C
/ \ /
D E F
/ \
H G
\ \
J K
*/
int main() {
BinaryTree tree;
//先序+中序二叉树创建
tree.PreOrderCreate();
//后序+中序二叉树创建
// tree.PostOrderCreate();
cout << "先序遍历结果(递归): ";
tree.PreOrderTraverseByRecursion(tree.GetRoot());
cout << endl;
cout << "先序遍历结果(非递归):";
tree.PreOrderTraverseByStack(tree.GetRoot());
cout << endl << endl;
cout << "中序遍历结果(递归): ";
tree.MidOrderTraverseByRecursion(tree.GetRoot());
cout << endl;
cout << "中序遍历结果(非递归):";
tree.MidOrderTraverseByStack(tree.GetRoot());
cout << endl << endl;
//后序遍历
cout << "后序遍历结果(递归): ";
tree.PostOrderTraverseByRecursion(tree.GetRoot());
cout << endl;
cout << "后序遍历结果(非递归):";
tree.PostOrderTraverseByStack(tree.GetRoot());
cout << endl << endl;
cout << "层次遍历结果: ";
tree.LevelOrder(tree.GetRoot());
cout << endl << endl;
cout << "树的高度:" << endl;
cout << "递归获取: " << tree.GetHeightByRecursion(tree.GetRoot()) << endl;
cout << "非递归获取:" << tree.GetHeightByQueue(tree.GetRoot()) << endl << endl;
cout << "树的宽度:";
cout << tree.GetWidth(tree.GetRoot()) << endl << endl;
cout << "树的节点数:" <<endl;
cout << "递归获取: " << tree.GetNodesNumByRecursion(tree.GetRoot()) << endl;
cout << "非递归获取:" << tree.GetNodesNumByStack(tree.GetRoot()) << endl << endl;
//获取某个节点的父节点
char ch = 'K';
cout << "获取" << ch << "的父节点为:";
Node* p = tree.GetParentNodeByRecursion(tree.GetRoot(), tree.GetAimNodeByVal(tree.GetRoot(), ch));
if (p) cout << p->val << endl;
else cout << "p = nullptr." << endl;
cout << endl;
//获取根节点到目标节点的完整路径,存入给定的栈中
ch = 'K';
stack<Node*> nodeStack;
tree.GetFullPathToAimNode(tree.GetRoot(), tree.GetAimNodeByVal(tree.GetRoot(), ch), nodeStack);
cout << "root:" << tree.GetRoot()->val <<"->" << ch << "的完整路径:";
while (!nodeStack.empty()) {
cout << nodeStack.top()->val << " ";
nodeStack.pop();
}
cout << endl << endl;
//销毁二叉树
BinaryTree* deleteTree = new BinaryTree();
deleteTree->PreOrderCreate();
cout << "两棵树是否相等:" << BinaryTree::Equal(deleteTree->GetRoot(), tree.GetRoot()) << endl;
cout << "节点删除顺序为:";
deleteTree->Destroy(deleteTree->GetRoot());
deleteTree = nullptr;
cout << endl << endl;
//x 和 y的最近公共节点
char x = 'K';
char y = 'J';
Node* node1 = tree.GetAimNodeByVal(tree.GetRoot(), x);
Node* node2 = tree.GetAimNodeByVal(tree.GetRoot(), y);
cout << x << " 和 " << y << "的最近公共祖先节点为:" << tree.GetLastCommonAncestor(tree.GetRoot(), node1, node2)->val << endl << endl;
cout << "左右子树交换" << endl;
cout << "交换前(中序遍历):";
tree.MidOrderTraverseByStack(tree.GetRoot());
cout << endl << "交换后(中序遍历):";
tree.SwapLeftRight(tree.GetRoot());
tree.MidOrderTraverseByStack(tree.GetRoot());
tree.SwapLeftRight(tree.GetRoot());
cout << endl;
return 0;
}
测试结果
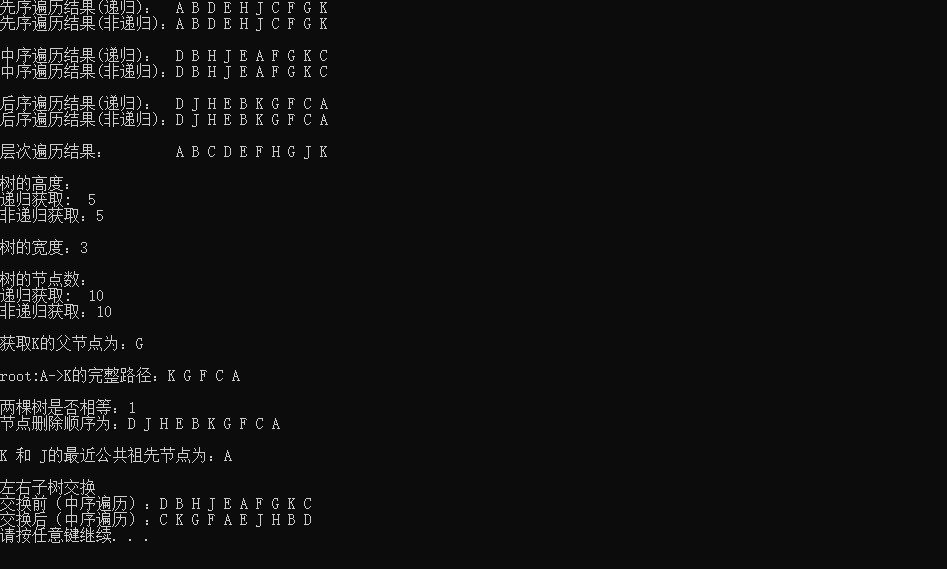