前言
最近开始在复杂项目中使用TypeScript,然而在使用过程中遇到了一些状况和报错,决定开一个系列记录遇到的问题和我的解决方法。
遍历对象属性
在TypeScript里面,当需要遍历对象的时候,经常就会遇到下图所示的错误提示。
function test (foo: object) {
for (let key in foo) {
console.log(foo[key]); // typescript错误提示
// do something
}
}
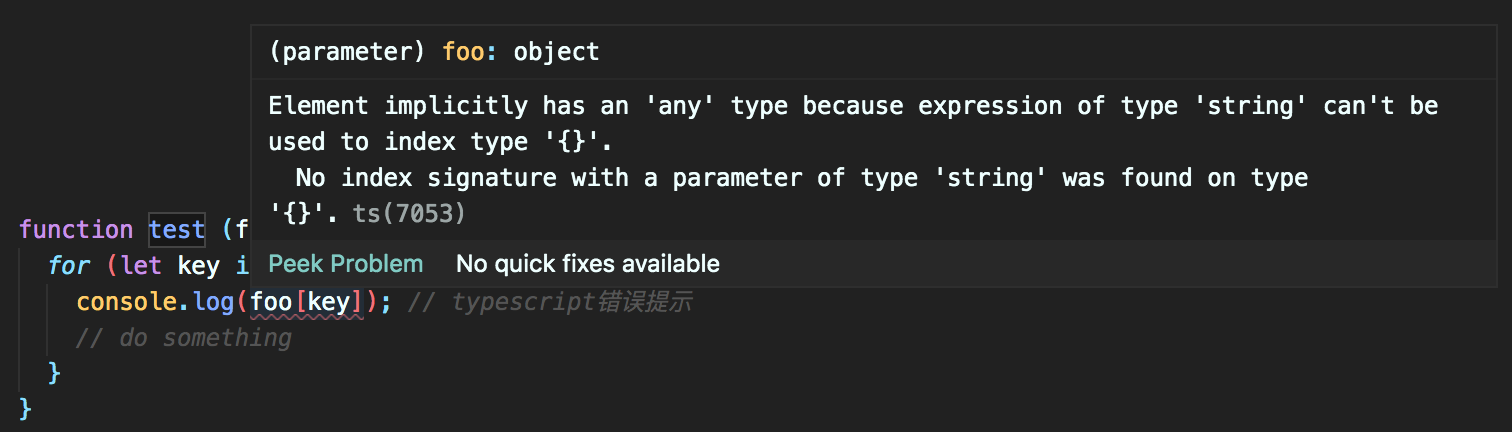
错误信息提示2个错误:
Element implicitly has an 'any' type because expression of type 'string' can't be used to index type '{}'.
因为foo作为object没有声明string类型可用,所以foo[key]将会是any类型。
No index signature with a parameter of type 'string' was found on type '{}'.
object没有找到string类型的
解决方法
那么,针对这种情况,我们有几种解决方法
1. 把对象声明as any
function test (foo: object) {
for (let key in foo) {
console.log((foo as any)[key]); // 报错消失
// do something
}
}
但是这个方法有点像是直接绕过了typescript的校验机制,失去了使用typescript的初心,这不是我们想要的。
2. 给对象声明一个接口
interface SimpleKeyValueObject {
[key: string]: any
}
function test (foo: SimpleKeyValueObject) {
for (let key in foo) {
console.log(foo[key]); // 报错消失
// do something
}
}
这个可以针对比较常见的对象类型,特别是一些工具方法。
但是这个不够骚,能不能再厉害点呢~
3. 使用泛型
function test<T extends object> (foo: T) {
for (let key in foo) {
console.log(foo[key]); // 报错消失
// do something
}
}
当我们需要对foo的key进行约束时,我们可以使用下面的第4种方法。
4. 使用keyof
interface Ifoo{
name: string;
age: number;
weight: number;
}
function test (opt: Ifoo) {
let key: (keyof Ifoo);
for (key in opt) {
console.log(opt[key]); // 报错消失
// do something
}
}