定时任务一般会在很多项目中都会用到,我们往往会间隔性的的去完成某些特定任务来减少服务器和数据库的压力。比较常见的就是金融服务系统推送回调,一般支付系统订单在没有收到成功的回调返回内容时会持续性的回调,这种回调一般都是定时任务来完成的。还有就是报表的生成,我们一般会在客户访问量过小的时候来完成这个操作,那往往都是在凌晨。这时我们也可以采用定时任务来完成逻辑。SpringBoot为我们内置了定时任务,我们只需要一个注解@Scheduled就可以开启定时任务了。
下面我们来通过SpringBoot项目熟悉一下具体实现
一,在pom.xml文件中加入依赖
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.6.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
二,创建启动类上添加注解@SpringBootApplication
和@EnableScheduling
@SpringBootApplication
@EntityScan(basePackages= {"cn.sh.sttri.app.messagelistener.*.entity"})
@EnableCaching
@EnableScheduling
public class MessagelistenerApplication {
public static void main(String[] args) {
SpringApplication.run(MessagelistenerApplication.class, args);
}
}
三,创建类ScheduledTask
用来演示定时任务
使用@scheduled注解来演示定时执行任务的方式 我们通过@scheduled注解用来配置到方法上来完成对应的定时任务的配置,如执行时间,间隔时间,延迟时间等等,下面我们就来详细的看下对应的属性配置。 @Component这个注解可以在程序启动时自动将该类加载进来
fixedDelay属性
该属性是程序启动后每3000ms执行一次
/**
* Created by zhangshuai on 2018/11/14 .
*/
@Component
public class ScheduledTask {
@Autowired
public QuartzJobService quartzJobService;
private static int count1=1;
private static final SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
@Scheduled(fixedDelay = 3000)
public void fixedDelay() {
System.out.println(String.format("1第%s次执行,当前时间为:%s", count1++, dateFormat.format(new Date(System.currentTimeMillis()))));
}
}
执行结果如下
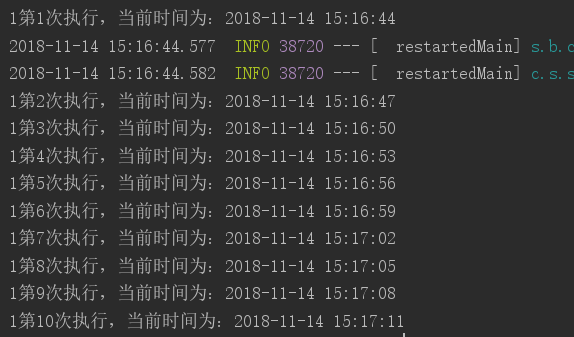
/**
* 每天中午十二点触发
*/
@Scheduled(cron="0 0 12 * * ?")
public void cron() {
System.out.println(String.format("1第%s次执行,当前时间为:%s", count1++, dateFormat.format(new Date(System.currentTimeMillis()))));
}
cron属性
这是一个时间表达式,可以通过简单的配置就能完成各种时间的配置,我们通过CRON表达式几乎可以完成任意的时间搭配,它包含了六或七个域: Seconds : 可出现", - * /"四个字符,有效范围为0-59的整数 Minutes : 可出现", - * /"四个字符,有效范围为0-59的整数 Hours : 可出现", - * /"四个字符,有效范围为0-23的整数 DayofMonth : 可出现", - * / ? L W C"八个字符,有效范围为0-31的整数 Month : 可出现", - * /"四个字符,有效范围为1-12的整数或JAN-DEc DayofWeek : 可出现", - * / ? L C #"四个字符,有效范围为1-7的整数或SUN-SAT两个范围。1表示星期天,2表示星期一, 依次类推 Year : 可出现", - * /"四个字符,有效范围为1970-2099年
下面简单举几个例子:
"0 0 12 * * ?" 每天中午十二点触发 "0 15 10 ? * *" 每天早上10:15触发 "0 15 10 * * ?" 每天早上10:15触发 "0 15 10 * * ? *" 每天早上10:15触发 "0 15 10 * * ? 2005" 2005年的每天早上10:15触发 "0 * 14 * * ?" 每天从下午2点开始到2点59分每分钟一次触发 "0 0/5 14 * * ?" 每天从下午2点开始到2:55分结束每5分钟一次触发 "0 0/5 14,18 * * ?" 每天的下午2点至2:55和6点至6点55分两个时间段内每5分钟一次触发 "0 0-5 14 * * ?" 每天14:00至14:05每分钟一次触发 "0 10,44 14 ? 3 WED" 三月的每周三的14:10和14:44触发 "0 15 10 ? * MON-FRI" 每个周一、周二、周三、周四、周五的10:15触发
fixedRate属性
该属性的含义是上一个调用开始后再次调用的延时(不用等待上一次调用完成),这样就会存在重复执行的问题,所以不是建议使用,但数据量如果不大时在配置的间隔时间内可以执行完也是可以使用的。
@Scheduled(fixedRate = 1000)
public void fixedRate() throws InterruptedException {
Thread.sleep(2000);
System.out.println(String.format("1第%s次执行,当前时间为:%s", count1++, dateFormat.format(new Date(System.currentTimeMillis()))));
}
initialDelay属性
该属性的作用是第一次执行延迟时间
@Scheduled(initialDelay=1000,fixedDelay = 3000)
public void initialDelay() {
System.out.println(String.format("1第%s次执行,当前时间为:%s", count1++, dateFormat.format(new Date(System.currentTimeMillis()))));
}
**注意:**上面所有属性的配置时间单位都是毫秒