Python简明语法
0.前言
本篇文章参考了python3-in-one-pic。
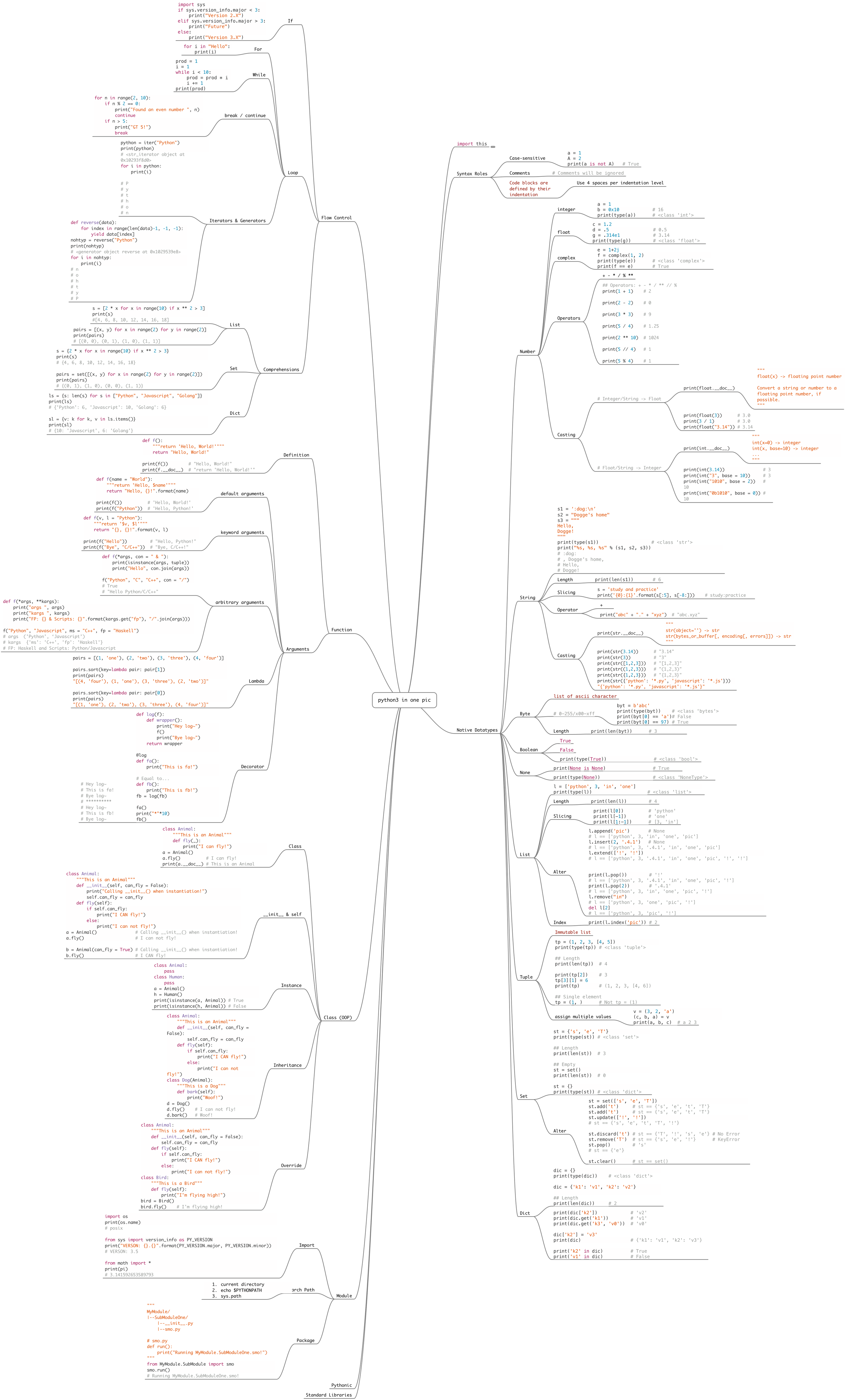
1.语法规则(Syntax Roles)
1.1 区分大小写(Case-sensitive)
a = 1
A = 2
print(a is not A) # True
1.2 注释(Comments)
# 注释会被忽略(Comments will be ignored)
1.3 代码块由首行缩进界定(Code blocks are defined by their indentation)
每一个首行缩进使用4个空格(Use 4 spaces per indentation)
2.基本数据类型(Native Datatypes)
2.1 数字(Number)
2.1.1 整型(integer)
a = 1
b = 0x10 # 16
print(type(a)) # <class 'int'>
2.1.2 浮点型(float)
C = 1.2
d = .5 # 0.5
g = .314e1 # 3.14
print(type(g)) # <class 'float'>
2.1.3 复数(complex)
e = 1 + 2j
f = complex(1, 2)
print(type(e)) # <class 'complex'>
print(f == e) # True
2.1.4 操作符(Operators)
## Operators:+-*/**//%
print(1 + 1) # 2
print(2 - 2) # 0
print(3 * 3) # 9
print(5 / 4) # 1.25
print(2 ** 10) # 1024
print(5 // 4) # 1
print(5 % 4) # 1
2.1.5 类型转换(Casting)
# Integer/String -> Float
print(float.__doc__)
"""
float(x)->floating point number
Convert a string or number to a floating point number, if possible.
"""
print(float(3)) # 3.0
print(3 / 1) # 3.0
print(float("3.14")) # 3.14
# Float/String -> Integer
print(int.__doc__)
"""
int(x=0)->integer
int(x,base=10)->integer
...
"""
print(int(3.14)) # 3
print(int("3",base = 10)) # 3
print(int("1010",base = 2)) # 10
print(int("0b1010",base = 8)) # 10
2.2 字符串(String)
s1 = ':dog:\n'
s2 = "Dogge's home"
s3 = """
Hel1o,
Dogge!
"""
print(type(s1)) # <class 'str'>
print("%s,%s,%s"%(s1,s2,s3))
# :dog:
# , Dogge's home,
# Hello,
# Dogge!
2.2.1 长度(Length)
print(len(s1)) # 6
2.2.2 分片(Slicing)
s = 'study and practice'
print('{0}:{1}'.format(s[:5], s[-8:])) # study:practice
2.2.3 操作符(Opetator)
print("abc" + "." + "xyz") # "abc.xyz"
2.2.4 类型转换(Casting)
print(str.__doc__)
"""
strCobject='') -> str
str(bytes_or_buffer[, encoding[, errors]) -> str
"""
print(str(3.14)) # "3.14"
print(str(3)) # "3"
print(str([1,2,3])) # "[1,2,3]"
print(str((1,2,3))) # "(1,2,3)"
print(str({1,2,3})) # "{1,2,3}"
print(str({'python': '*.py', 'javascript': '*.js'}))
"{'python': '*.py', 'javascript': '*.js'}"
2.3 字节(Byte)
2.3.1 list of ascii character
2.3.2 0-255/x00-xff
byt = b'abc'
print(type(byt)) # <class 'bytes'>
print(byt[0] == 'a') # False
print(byt[0] == 97) # True
2.3.3 Length
print(len(byt)) # 3
2.4 布尔值(Boolean)
True
False
print(type(True)) # <class 'bool'>
2.5 空类型(None)
print(None is None) # True
print(type(None)) # <class 'NoneType'>
2.6 列表(List)
l = ['python', 3, 'in', 'one']
print(type(l)) # <class 'list'>
2.6.1 长度(Length)
print(len(l)) # 4
2.6.2 分片(Slicing)
print(1[0]) # 'python'
print(1[-1]) # 'one'
print(1[1:-1]) # [3, 'in']
2.6.3 更改(Alter)
l.append(' pic') # None
# l == ['python', 3, 'in', 'one', 'pic']
l.insert(2,'.4.1') # None
# l == ['python', 3, '.4.1', 'in', 'one', 'pic']
l.extend([!',!'])
# l == ['python', 3, '.4.1', 'in', 'one', 'pic', '!', '!']
print(1. pop()) # '!'
# l == ['python', 3, '.4.1', 'in', 'one', 'pic', '!']
print(1. pop(2)) # '.4.1'
# l == ['python', 3, 'in', 'one', 'pic', '!']
l.remove("in")
# l == ['python', 3, 'one', 'pic', '!']
del l[2]
# l == ['python', 3, 'pic', '!']
2.6.4 索引(Index)
print(l.index('pic')) # 2
2.7 元组(Tuple)
不可更改的列表(Immutable list)
tp=(1,2,3,[4,5])
print(type(tp)) # <class 'tuple'>
## Length
print(len(tp)) # 4
print(tp[2]) # 3
tp[3][1]=6
print(tp) # (1, 2, 3, [4, 6])
## Single element
tp=(1,) # Not tp = (1)
分配多个变量值(assign multiple values)
v = (3, 2, 'a')
(c, b, a) = v
print(a, b, c) # a 2 3
2.8 集合(Set)
st = {'s','e','T'}
print(type(st)) # <class 'set'>
##Length
print(ien(st)) # 3
##Empty
st = set()
print(len(st)) # 0
st = {}
print(type(st)) # <class 'dict'>
Alter
st = set(['s', 'e', 'T'])
st.add('t') # st == {'s', 'e', 't', 'T'}
st.add('t") # st == {'s', 'e', 't', 'T'}
st.update(['!',!'])
# st == {'s', 'e', 't', 'T', '!'}
st.discard('t') # st == {'T',!",'s','e'} # No Error
st.remove('T') # st == {'s', 'e', '!'} # KeyError
st.pop() # 's'
# st == {'e'}
st.clear() # st == set()
2.9 字典(Dict)
dic = {}
print(type(dic)) # <class 'dict'>
dic = {'k1': 'v1', 'k2': 'v2'}
## Length
print(len(dic)) # 2
print(dic['k2']) # 'v2'
print(dic.get('k1')) # 'v1'
print(dic.get('k3','v0')) # 'vo'
dic['k2'] = 'v3'
print(dic) # {'k1': 'v1', 'k2': 'v3'}
print('k2' in dic) # True
print('v1' in dic) # False
3.流程控制(Flow Control)
3.1 如果(If)
import sys
if sys.version_info. major < 3:
print("Version 2.X")
elif sys.version_info. major > 3:
print("Future")
else:
print("Version 3.X")
3.2 循环(Loop)
3.2.1 For
for i in "Hello":
print(i)
3.2.2 While
prod = 1
i = 1
while i < 10:
prod = prod * i
i += 1
print(prod)
3.2.3 break / continue
for n in range(2, 10):
if n % 2 == 0:
print("Found an even number", n)
continue
if n > 5:
print("GT 5!")
break
3.2.4 迭代器 & 生成器(Iterators & Generators)
python = iter("Python")
print(python)
# <str_iterator object at 0x10293f8d0>
for i in python:
print(i)
# P
# y
# t
# h
# o
# n
def reverse(data):
for index in range(len(data)-1, -1, -1):
yield data[index]
nohtyp = reverse("Python")
print(nohtyp)
# <generator object reverse at 9x1829539e8>
for i in nohtyp:
print(i)
# n
# o
# h
# t
# y
# P
3.3 解析式(Comprehensions)
3.3.1 列表(List)
s = [2 * x for x in range(10) if x ** 2 > 3]
print(s)
# [4, 6, 8, 10, 12, 14, 16, 18]
pairs = [(x, y)for x in range(2) for y in range(2)]
print(pairs)
# [(0, 0), (0, 1), (1, 0), (1, 1)]
3.3.2 集合(Set)
s = {2 * x for x in range(10) if x ** 2 > 3}
print(s)
# 4, 6, 8, 10, 12, 14, 16, 18}
pairs = set([(x, y)for x in range(2) for y in range(2)])
print(pairs)
# {(0, 1), (1, 0), (e, 0), (1, 1)}
3.3.3 字典(Dict)
ls = {s: len(s) for s in ["Python", "Javascript", "Golang"]}
print(ls)
# {"Python': 6, ' Javascript': 10, ' Golang': 6}
s1 = {v: k for k, v in ls.items)}
print(sl)
# {10: 'Javascript', 6: 'Golang'}
4.函数(Function)
4.1 定义(Definition)
def f():
"""return 'Hello, World!'"""
return "Hello, World!"
print(f()) # "Hello, World!"
print(f.__doc__) # "return 'Hello, World!'"
4.2 参数(Arguments)
4.2.1 默认参数(default arguments)
def f(name = "World"):
"""return 'Hello, $name'"""
return "Hello,{}!".format(name)
print(f()) # 'Hello, World!'
print(f("Python")) # 'Hello, Python!'
4.2.2 关键词参数(keyword arguments)
def f(v,l = "Python"):
"""return '$v, $l'"""
return "{}, {}!".format(v, l)
print(f("Hello")) # "Hello, Python!"
print(f("Bye", "C/C++")) # "Bye, C/C++!"
4.2.3 任意参数(arbitrary arguments)
def f(*args, con = "&"):
print(isinstance(args, tuple))
print("Hello", con.join(args))
f("Python", "C", "C++", con = "/")
# True
# "Hello Python/C/C++"
def f(*args, **kargs):
print("args", args)
print("kargs", kargs)
print("FP: {} & Scripts: {}".format(kargs.get("fp"), "/".join(args)))
f("Python", "Javascript", ms = "C++", fp = "Haskell")
# args ('Python', 'Javascript')
# kargs {'ms': 'C++', 'fp': 'Haskell'}
# FP: Haskell and Scripts: Python/Javascript
4.2.4 lambda表达式(Lambda)
pairs = [(1, 'one'), (2, 'two'), (3, 'three'), (4, 'four')]
pairs.sort(key = lambda pair: pair[1])
print(pairs)
"[(4, 'four'), (1, 'one'), (3, 'three'), (2, 'two')]"
pairs.sort(key = lambda pair: pair[0])
print(pairs)
"[(1, 'one'), (2, 'two'), (3, 'three'), (4, 'four')]"
4.2.5 装饰器(Decorator)
def log(f):
def wrapper():
print("Hey log~")
f()
print("Bye log~")
return wrapper
@Log
def fa():
print("This is fa!")
# Equal to...
def fb():
print("This is fb!")
fb = Log(fb)
fa()
print("*"*10)
fb()
# Hey log~
# This is fa!
# Bye log~
# **********
# Hey log~
# This is fb!
# Bye log~
5.类(面向对象)(Class(OOP))
5.1 类(Class)
class Animal:
"""This is an Animal."""
def fly():
print("I can fly!")
a = Animal()
a.fly() # I can fly!
print(a.__doc__) # This is an Animal
5.2 构造函数(init & self)
class Animal:
"""This is an Animal"""
def __init__(self, can_fly = False):
print("Calling__init__() when instantiation!")
self.can_fly = can_fly
def fly(self):
if self.can_fly:
print("I CAN fly!")
else:
print("I can not fly!")
a = Animal() # Calling __init__() when instantiation!
a.fly() # I can not fly!
b = Animal(can_fly = True) # Calling __init__() when instantiation!
b.fly() # I CAN fly!
5.3 实例(Instance)
class Animal:
pass
class Human:
pass
a = Animal()
h = Human()
print(isinstance(a,Animal)) # True
print(isinstance(h,Animal)) # False
5.4 继承(Inheritance)
class Animal:
"""This is an Animal"""
def __init__(self, can_fly = False):
self.can_fly = can_fly
def fly(self):
if self.can_fly:
print("I CAN fly!")
else:
print("I can not fly!")
class Dog(Animal):
"""This is a Dog"""
def bark(self):
print("Woof!")
d = Dog()
d.fly() # I can not fly!
d.bark() # Woof!
5.5 重载(Override)
class Animal:
"""This is an Animal"""
def __init__(self, can_fly = False):
self.can_fly = can_fly
def fly(self):
if self.can_fly:
print("I CAN fly!")
else:
print("I can not fly!")
class Bird:
"""This is a Bird"""
def fly(self):
printC"I'm flying high!")
bird = Bird()
bird.fly() # I'm flying high!
6.模块(Module)
6.1 导入(Import)
import os
print(os.name)
# posix
from sys import version_info as PY_VERSION
print("VERSON:{}.{}".format(PY_VERSION.major, PY_VERSION. minor))
# VERSON:3.5
from math import *
print(pi)
# 3.141592653589793
6.2 搜索路径(Search Path)
1.current directory
2.echo $PYTHONPATH
3.sys.path
6.3 打包(Package)
""
MyModule/
|--SubModuleOne/
|--__init__.py
|--smo.py
# smo.py
def run):
print("Running MyModule.SubModuleOne. smo!")
"""
from MyModule.SubModule import smo
smo.run()
# Running MyModule.SubModuleOne.smo!