版本记录
版本号 | 时间 |
---|---|
V1.0 | 2018.11.23 星期五 |
前言
iOS中有关视图控件用户能看到的都在UIKit框架里面,用户交互也是通过UIKit进行的。感兴趣的参考上面几篇文章。
1. UIKit框架(一) —— UIKit动力学和移动效果(一)
2. UIKit框架(二) —— UIKit动力学和移动效果(二)
3. UIKit框架(三) —— UICollectionViewCell的扩张效果的实现(一)
4. UIKit框架(四) —— UICollectionViewCell的扩张效果的实现(二)
5. UIKit框架(五) —— 自定义控件:可重复使用的滑块(一)
6. UIKit框架(六) —— 自定义控件:可重复使用的滑块(二)
7. UIKit框架(七) —— 动态尺寸UITableViewCell的实现(一)
源码
1. Swift
首先看一下工程文件结构
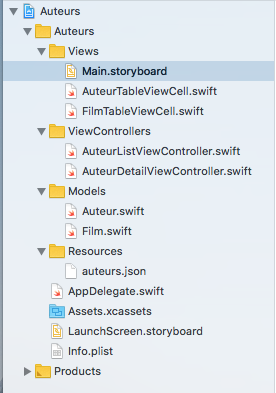
再看一下xib文件
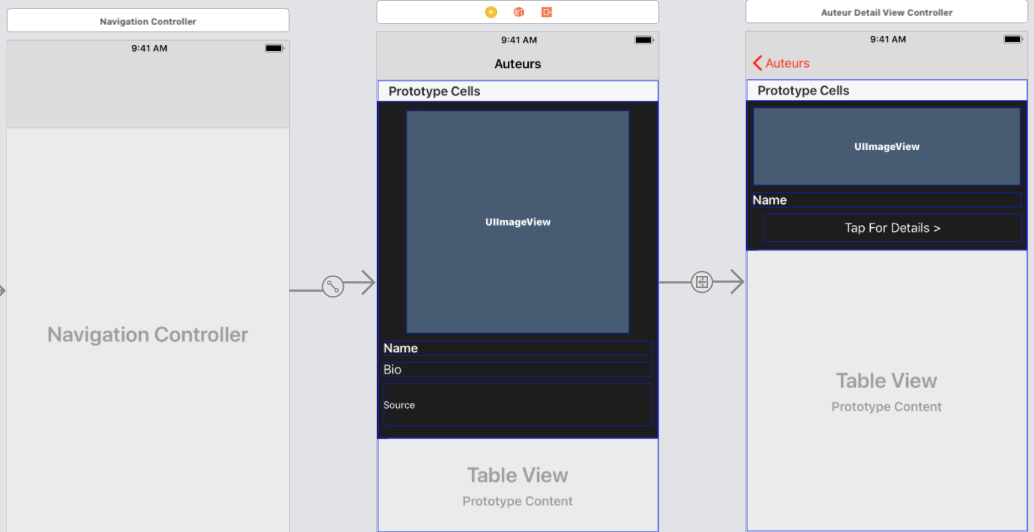
下面就是看一下源码了
1. AuteurListViewController.swift
import UIKit
class AuteurListViewController: UIViewController {
let auteurs = Auteur.auteursFromBundle()
@IBOutlet weak var tableView: UITableView!
override func viewDidLoad() {
super.viewDidLoad()
tableView.rowHeight = UITableView.automaticDimension
tableView.estimatedRowHeight = 600
navigationController?.navigationBar.largeTitleTextAttributes = [NSAttributedString.Key.font: UIFont.systemFont(ofSize: 34, weight: .bold) ]
navigationItem.largeTitleDisplayMode = .automatic
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if let destination = segue.destination as? AuteurDetailViewController,
let indexPath = tableView.indexPathForSelectedRow {
destination.selectedAuteur = auteurs[indexPath.row]
}
}
}
extension AuteurListViewController: UITableViewDataSource {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return auteurs.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath) as! AuteurTableViewCell
let auteur = auteurs[indexPath.row]
cell.bioLabel.text = auteur.bio
cell.auteurImageView.image = UIImage(named: auteur.image)
cell.nameLabel.text = auteur.name
cell.source.text = auteur.source
cell.auteurImageView.layer.cornerRadius = cell.auteurImageView.frame.size.width / 2
cell.nameLabel.textColor = .white
cell.bioLabel.textColor = UIColor(red:0.75, green:0.75, blue:0.75, alpha:1.0)
cell.source.textColor = UIColor(red:0.74, green:0.74, blue:0.74, alpha:1.0)
cell.source.font = UIFont.italicSystemFont(ofSize: cell.source.font.pointSize)
cell.nameLabel.textAlignment = .center
cell.selectionStyle = .none
return cell
}
}
2. AuteurDetailViewController.swift
import UIKit
class AuteurDetailViewController: UIViewController {
var selectedAuteur: Auteur!
let moreInfoText = "Tap For Details >"
@IBOutlet weak var tableView: UITableView!
override func viewDidLoad() {
super.viewDidLoad()
tableView.rowHeight = UITableView.automaticDimension
tableView.estimatedRowHeight = 300
title = selectedAuteur.name
self.tableView.contentInsetAdjustmentBehavior = .never
}
}
extension AuteurDetailViewController: UITableViewDataSource {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return selectedAuteur.films.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath) as! FilmTableViewCell
let film = selectedAuteur.films[indexPath.row]
cell.filmTitleLabel.text = film.title
cell.filmImageView.image = UIImage(named: film.poster)
cell.filmTitleLabel.textColor = .white
cell.filmTitleLabel.textAlignment = .center
cell.moreInfoTextView.textColor = .red
cell.selectionStyle = .none
cell.moreInfoTextView.text = film.isExpanded ? film.plot : moreInfoText
cell.moreInfoTextView.textAlignment = film.isExpanded ? .left : .center
cell.moreInfoTextView.textColor = film.isExpanded ? UIColor(red:0.75, green:0.75, blue:0.75, alpha:1.0) : .red
return cell
}
}
extension AuteurDetailViewController: UITableViewDelegate {
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
guard let cell = tableView.cellForRow(at: indexPath) as? FilmTableViewCell else { return }
var film = selectedAuteur.films[indexPath.row]
film.isExpanded = !film.isExpanded
selectedAuteur.films[indexPath.row] = film
cell.moreInfoTextView.text = film.isExpanded ? film.plot : moreInfoText
cell.moreInfoTextView.textAlignment = film.isExpanded ? .left : .center
cell.moreInfoTextView.textColor = film.isExpanded ? UIColor(red:0.75, green:0.75, blue:0.75, alpha:1.0) : .red
tableView.beginUpdates()
tableView.endUpdates()
tableView.scrollToRow(at: indexPath, at: .top, animated: true)
}
}
3. AuteurTableViewCell.swift
import UIKit
class AuteurTableViewCell: UITableViewCell {
@IBOutlet weak var bioLabel: UILabel!
@IBOutlet weak var nameLabel: UILabel!
@IBOutlet weak var source: UILabel!
@IBOutlet weak var auteurImageView: UIImageView!
}
4. FilmTableViewCell.swift
import UIKit
class FilmTableViewCell: UITableViewCell {
@IBOutlet weak var filmImageView: UIImageView!
@IBOutlet weak var filmTitleLabel: UILabel!
@IBOutlet weak var moreInfoTextView: UITextView!
}
5. Auteur.swift
import UIKit
struct Auteurs : Codable {
let auteurs : [Auteur]
}
struct Auteur: Codable {
let name: String
let bio: String
let source: String
let image: String
var films: [Film]
// Decode JSON
static func auteursFromBundle() -> [Auteur] {
var auteurs: [Auteur] = []
let url = Bundle.main.url(forResource: "auteurs", withExtension: "json")!
do {
let data = try Data(contentsOf: url)
let json = try JSONDecoder().decode(Auteurs.self, from: data)
auteurs = json.auteurs
}
catch {
print("Error occured during Parsing", error)
}
return auteurs
}
}
6. Film.swift
import UIKit
struct Film: Codable {
let title: String
let year: String
let poster: String
let plot: String
var isExpanded: Bool
// Custom init to set isExpanded bool to false
init(from decoder: Decoder) throws {
let container = try decoder.container(keyedBy: CodingKeys.self)
title = try container.decode(String.self, forKey: .title)
year = try container.decode(String.self, forKey: .year)
poster = try container.decode(String.self, forKey: .poster)
plot = try container.decode(String.self, forKey: .plot)
isExpanded = false
}
}
下面看下效果
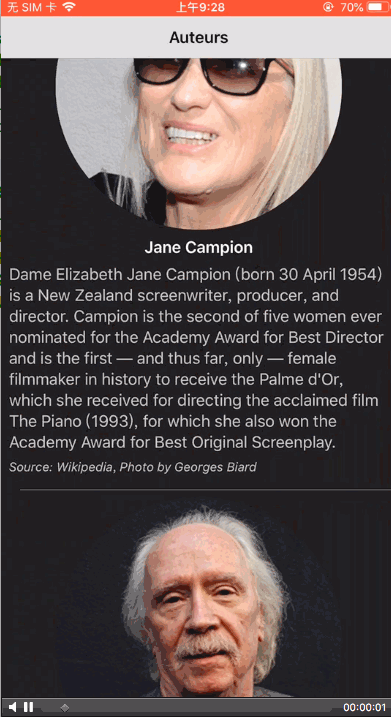
后记
本篇主要讲述了动态尺寸UITableViewCell的实现源码,感兴趣的给个赞或者关注~~~
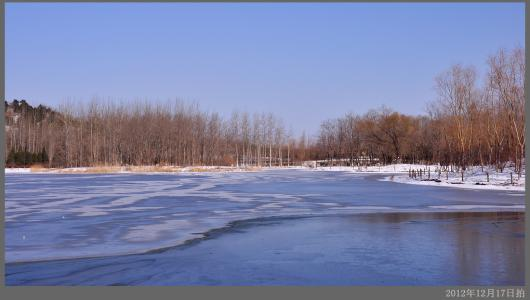