xml方式
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
id="WebApp_ID" version="3.0">
<display-name>CtrTimeOut</display-name>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
<context-param>
<param-name>contextConfigLocation</param-name>
# spring的配置
<param-value>classpath:config/spring/applicationContext.xml</param-value>
</context-param>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<servlet>
<servlet-name>controller</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
# springmvc的配置
<param-value>classpath:config/spring/spring-controller.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>controller</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app>
可以看到xml配置方式借助了了ContextLoaderListener来启动
看下ContextLoaderListener的类定义
public class ContextLoaderListener extends ContextLoader implements ServletContextListener
ContextLoaderListener实现了ServletContextListener
public interface ServletContextListener extends EventListener {
//容器初始化完成
public void contextInitialized(ServletContextEvent sce);
//容器停止
public void contextDestroyed(ServletContextEvent sce);
}
由servlet标准可知ServletContextListener是容器的生命周期方法,springmvc就借助其启动与停止
ContextLoadListener调用了initWebApplicationContext方法,创建WebApplicationContext作为spring的容器上下文
#org.springframework.web.context.ContextLoader
/**
* 根据xml配置创建applicationContext
*/
public WebApplicationContext initWebApplicationContext(ServletContext servletContext) {
...
try {
// Store context in local instance variable, to guarantee that
// it is available on ServletContext shutdown.
if (this.context == null) {//判空 (以注解方式配置时非空)
this.context = createWebApplicationContext(servletContext);
}
if (this.context instanceof ConfigurableWebApplicationContext) {
ConfigurableWebApplicationContext cwac = (ConfigurableWebApplicationContext) this.context;
if (!cwac.isActive()) {
...
//读取contextConfigLocation配置并refresh()
configureAndRefreshWebApplicationContext(cwac, servletContext);
}
}
//将applicationContext设置到servletContext中
servletContext.setAttribute(WebApplicationContext.ROOT_WEB_APPLICATION_CONTEXT_ATTRIBUTE, this.context);
...
return this.context;
}
}
而DispatcherServlet创建WebApplicationContext作为springmvc的上下文 并将ContextLoadListener创建的上下文设置为自身的parent
DispatcherServlet extends FrameworkServlet
#org.springframework.web.servlet.FrameworkServlet
@Override
protected final void initServletBean() throws ServletException {
...
try {
this.webApplicationContext = initWebApplicationContext();
initFrameworkServlet();
}
...
}
protected WebApplicationContext initWebApplicationContext() {
WebApplicationContext rootContext =
WebApplicationContextUtils.getWebApplicationContext(getServletContext());
WebApplicationContext wac = null;
...
if (wac == null) {
//创建applicationContext
wac = createWebApplicationContext(rootContext);
}
...
return wac;
}
protected WebApplicationContext createWebApplicationContext(ApplicationContext parent) {
//XmlWebApplicationContext
Class<?> contextClass = getContextClass();
...
//创建applicationContext
ConfigurableWebApplicationContext wac =
(ConfigurableWebApplicationContext) BeanUtils.instantiateClass(contextClass);
wac.setEnvironment(getEnvironment());
//设置parent(ContextLoadListener中创建的applicationContext)
wac.setParent(parent);
//读取contextConfigLocation配置
wac.setConfigLocation(getContextConfigLocation());
//refresh()
configureAndRefreshWebApplicationContext(wac);
return wac;
}
springmvc的applicationContext会去读取配置文件 我们来看一个最简单的配置文件
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.0.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd
http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.0.xsd"
default-autowire="byName">
#springmvc容器扫描路径
<context:component-scan base-package="com.iflytek.ossp.ctrtimeout.controller"></context:component-scan>
#spring4新增的标签 主要是添加了默认的HandleMappin,ViewResolver,HandleAdapter
<mvc:annotation-driven />
</beans>
springmvc标签解析
根据spring的自定义schema解析机制 我们找到 在下图位置
http\://www.springframework.org/schema/mvc=org.springframework.web.servlet.config.MvcNamespaceHandler
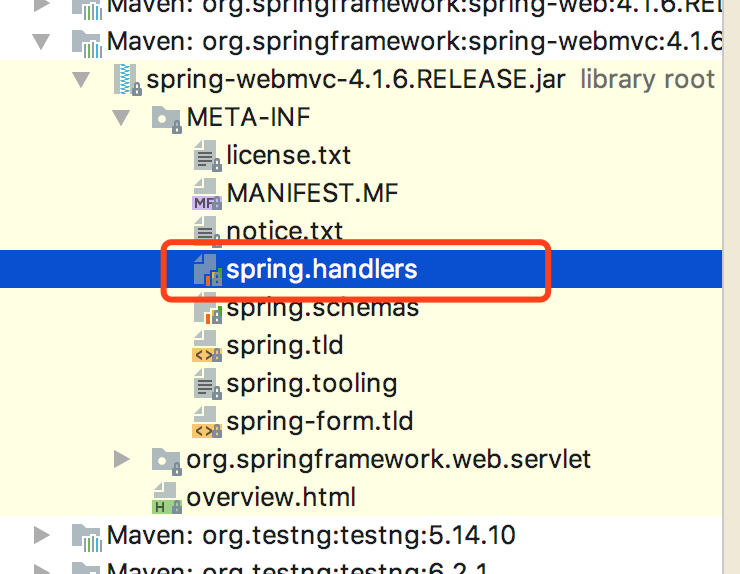
可以看到mvc所有的标签解析器都定义在此
public class MvcNamespaceHandler extends NamespaceHandlerSupport {
@Override
public void init() {
registerBeanDefinitionParser("annotation-driven", new AnnotationDrivenBeanDefinitionParser());
registerBeanDefinitionParser("default-servlet-handler", new DefaultServletHandlerBeanDefinitionParser());
registerBeanDefinitionParser("interceptors", new InterceptorsBeanDefinitionParser());
registerBeanDefinitionParser("resources", new ResourcesBeanDefinitionParser());
registerBeanDefinitionParser("view-controller", new ViewControllerBeanDefinitionParser());
registerBeanDefinitionParser("redirect-view-controller", new ViewControllerBeanDefinitionParser());
registerBeanDefinitionParser("status-controller", new ViewControllerBeanDefinitionParser());
registerBeanDefinitionParser("view-resolvers", new ViewResolversBeanDefinitionParser());
registerBeanDefinitionParser("tiles-configurer", new TilesConfigurerBeanDefinitionParser());
registerBeanDefinitionParser("freemarker-configurer", new FreeMarkerConfigurerBeanDefinitionParser());
registerBeanDefinitionParser("velocity-configurer", new VelocityConfigurerBeanDefinitionParser());
registerBeanDefinitionParser("groovy-configurer", new GroovyMarkupConfigurerBeanDefinitionParser());
}
}
来看一下AnnotationDrivenBeanDefinitionParser解析器做了什么
解析过程较为复杂 通过注释我们可以得知以下对象将被装载
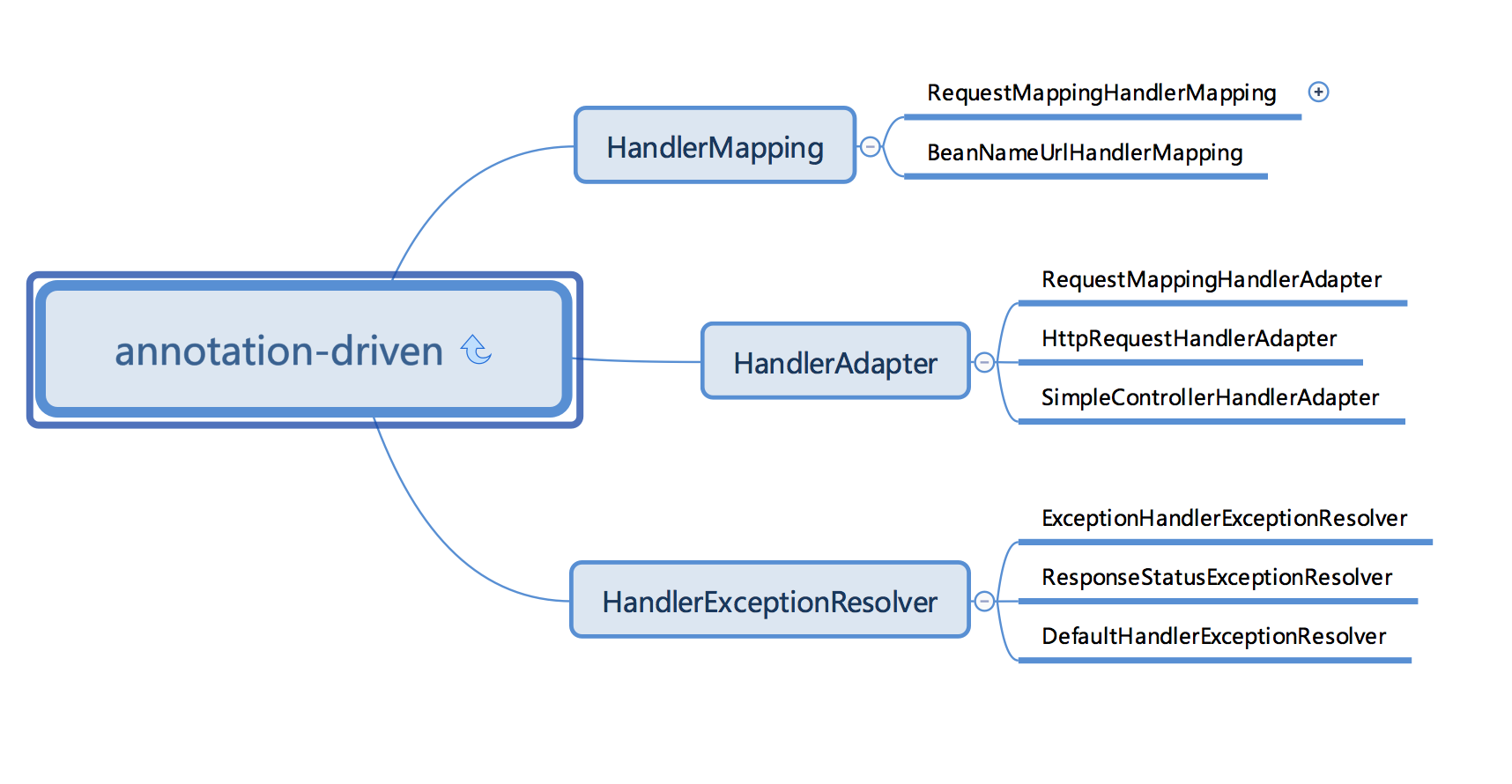
java方式
public class WebAppInitializer extends AbstractAnnotationConfigDispatcherServletInitializer{
//加载spring配置 创建spring上下文
@Override
protected Class<?>[] getRootConfigClasses() {
return new Class<?>[] {RootConfig.class};
}
//加载springMvc配置 创建springMVC上下文
@Override
protected Class<?>[] getServletConfigClasses() {
return new Class<?>[] {WebConfig.class}; // 指定配置类
}
//DiapatchServlet映射路径
@Override
protected String[] getServletMappings() {
return new String[] {"/"}; // 将dispatcherServlet映射到“/”
}
}
可以看到,同xml方式相同,java方式启动也有两个上下文
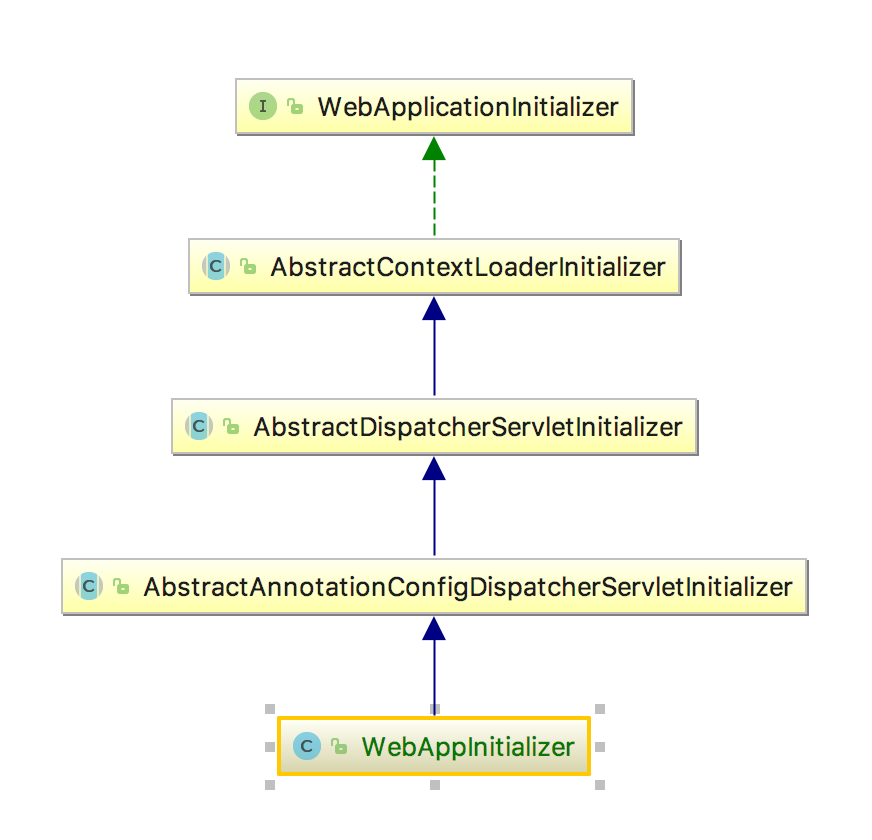
同xml方式类似,java配置启动方式也需要借助于servlet容器的生命周期方法,就是WebApplicationInitializer接口
public interface WebApplicationInitializer {
//容器启动时被调用
void onStartup(ServletContext servletContext) throws ServletException;
}
注: WebApplicationInitializer是spring定义的接口,它能够响应容器生命周期的原因是因为SpringServletContainerInitializer
@HandlesTypes(WebApplicationInitializer.class)
public class SpringServletContainerInitializer implements ServletContainerInitializer{
//得到所有WebApplicationInitializer实现 并调用其onStartup()方法
@Override
public void onStartup(Set<Class<?>> webAppInitializerClasses, ServletContext servletContext)
throws ServletException {
List<WebApplicationInitializer> initializers = new LinkedList<WebApplicationInitializer>();
...
for (Class<?> waiClass : webAppInitializerClasses) {
...
initializers.add((WebApplicationInitializer) waiClass.newInstance());
...
}
...
AnnotationAwareOrderComparator.sort(initializers);
for (WebApplicationInitializer initializer : initializers) {
initializer.onStartup(servletContext);
}
}
}
//真正的容器生命周期方法
//会根据@HandlesTypes注解获取所有类实现,并作为onStartup()方法的第一个参数
public interface ServletContainerInitializer {
public void onStartup(Set<Class<?>> c, ServletContext ctx)
throws ServletException;
}
启动过程:
- AbstractContextLoaderInitializer调用createRootApplicationContext创建spring上下文
- AbstractDispatcherServletInitializer调用createServletApplicationContext创建springMVC上下文
经过以上两步spring容器就启动起来了
再看xml方式通过mvc标签来添加默认实现(HandlerMapping,HandlerAdapter等等)
java方式则通过@EnableWebMvc注解来添加默认实现
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
@Documented
@Import(DelegatingWebMvcConfiguration.class)
public @interface EnableWebMvc {
}
@Configuration
public class DelegatingWebMvcConfiguration extends WebMvcConfigurationSupport {
private final WebMvcConfigurerComposite configurers = new WebMvcConfigurerComposite();
@Autowired(required = false)
public void setConfigurers(List<WebMvcConfigurer> configurers) {
if (!CollectionUtils.isEmpty(configurers)) {
this.configurers.addWebMvcConfigurers(configurers);
}
}
@Override
protected void configurePathMatch(PathMatchConfigurer configurer) {
this.configurers.configurePathMatch(configurer);
}
@Override
protected void configureContentNegotiation(ContentNegotiationConfigurer configurer) {
this.configurers.configureContentNegotiation(configurer);
}
@Override
protected void configureAsyncSupport(AsyncSupportConfigurer configurer) {
this.configurers.configureAsyncSupport(configurer);
}
@Override
protected void configureDefaultServletHandling(DefaultServletHandlerConfigurer configurer) {
this.configurers.configureDefaultServletHandling(configurer);
}
@Override
protected void addFormatters(FormatterRegistry registry) {
this.configurers.addFormatters(registry);
}
@Override
protected void addInterceptors(InterceptorRegistry registry) {
this.configurers.addInterceptors(registry);
}
@Override
protected void addResourceHandlers(ResourceHandlerRegistry registry) {
this.configurers.addResourceHandlers(registry);
}
@Override
protected void addCorsMappings(CorsRegistry registry) {
this.configurers.addCorsMappings(registry);
}
@Override
protected void addViewControllers(ViewControllerRegistry registry) {
this.configurers.addViewControllers(registry);
}
@Override
protected void configureViewResolvers(ViewResolverRegistry registry) {
this.configurers.configureViewResolvers(registry);
}
@Override
protected void addArgumentResolvers(List<HandlerMethodArgumentResolver> argumentResolvers) {
this.configurers.addArgumentResolvers(argumentResolvers);
}
@Override
protected void addReturnValueHandlers(List<HandlerMethodReturnValueHandler> returnValueHandlers) {
this.configurers.addReturnValueHandlers(returnValueHandlers);
}
@Override
protected void configureMessageConverters(List<HttpMessageConverter<?>> converters) {
this.configurers.configureMessageConverters(converters);
}
@Override
protected void extendMessageConverters(List<HttpMessageConverter<?>> converters) {
this.configurers.extendMessageConverters(converters);
}
@Override
protected void configureHandlerExceptionResolvers(List<HandlerExceptionResolver> exceptionResolvers) {
this.configurers.configureHandlerExceptionResolvers(exceptionResolvers);
}
@Override
protected void extendHandlerExceptionResolvers(List<HandlerExceptionResolver> exceptionResolvers) {
this.configurers.extendHandlerExceptionResolvers(exceptionResolvers);
}
@Override
protected Validator getValidator() {
return this.configurers.getValidator();
}
@Override
protected MessageCodesResolver getMessageCodesResolver() {
return this.configurers.getMessageCodesResolver();
}
}
@IMPORT是spring4的注解 用于关联@Configuration类
DelegatingWebMvcConfiguration:像它的名字一样只是个delegate,起作用的主要是其父类WebMvcConfigurationSupport.
WebMvcConfigurationSupport:其中注册了大量的默认实现(如同AnnotationDrivenBeanDefinitionParser一样),同时它的持有一个WebMvcConfigurerComposite对象.
WebMvcConfigurerComposite:内部聚合了WebMvcConfigurerAdapter的集合.
WebMvcConfigurerAdapter:用于添加自定义HandlerAdapter,HandlerMapping等
springMVC的启动过程就是这样了..