准备工作之前得准备三个文件:一个是CSR请求文件(CertificateSigningRequest)、一个是aps_development.cer的SSL证书文件、还有一个是推送的PushDeveloper.p12秘钥文件。如下图所示:
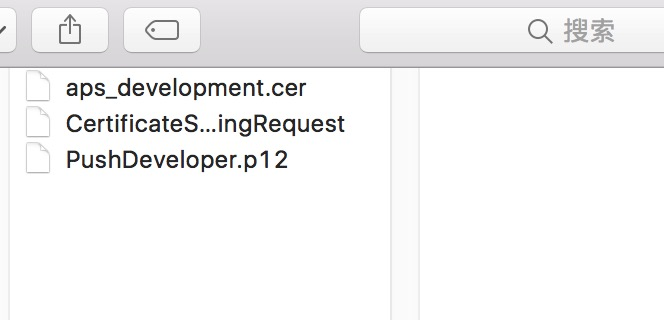
1.生成Certificate Signing Request步骤省略。
2.下载开发证书和发布证书
到苹果开发官网描述下载证书,以开发证书为案例。如下图所示:
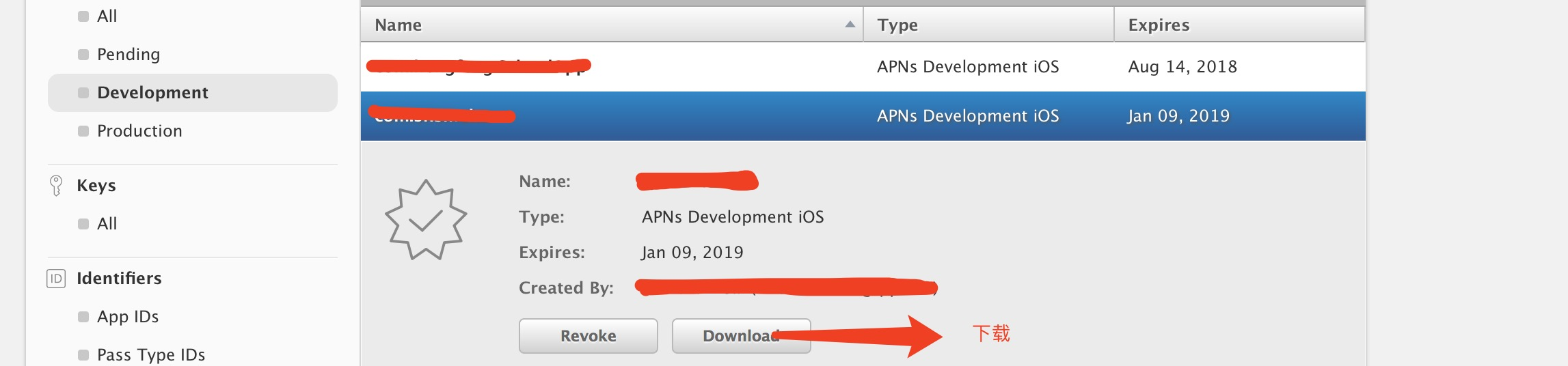
3.从钥匙串访问中导出密钥
打开钥匙串访问,找到我们的专用密钥(专用秘钥就是我们下载推送证书安装本地的私人密钥),如下图所示:
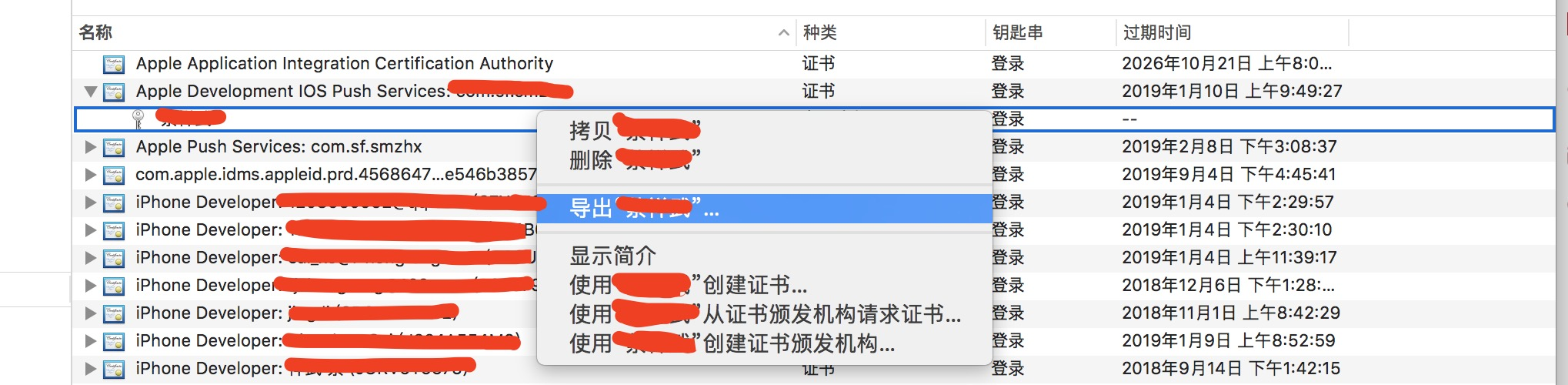
导出.p12文件的时候需要输入密码,我这里选择简单点为123456,这密码需要记住,后面有用到。
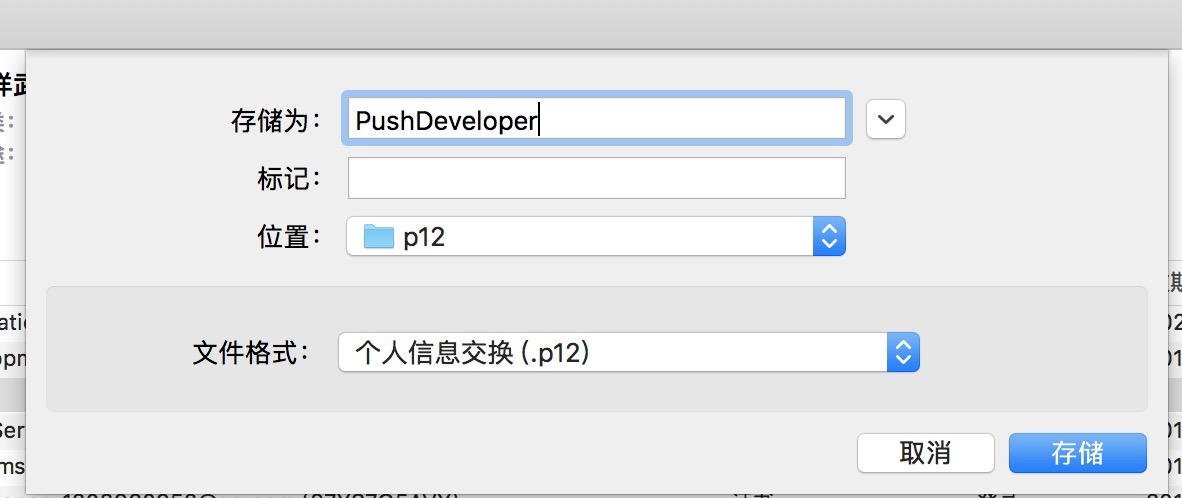
上面步骤完成后打开终端 cd到p12目录,就是放那三个文件所在的文件夹目录
1.把aps_development.cer的SSL证书转换为PushChatCert.pem文件,执行命令:
openssl x509 -in aps_development.cer -inform der -out PushChatCert.pem

在p12目录下会生成一个PushChatCert.pem文件
2.把PushDeveloper.p12格式的私钥转化成PushChatKey.pem,需要设置密码,密码为abc123
openssl pkcs12 -nocerts -out PushChatKey.pem -in PushDeveloper.p12
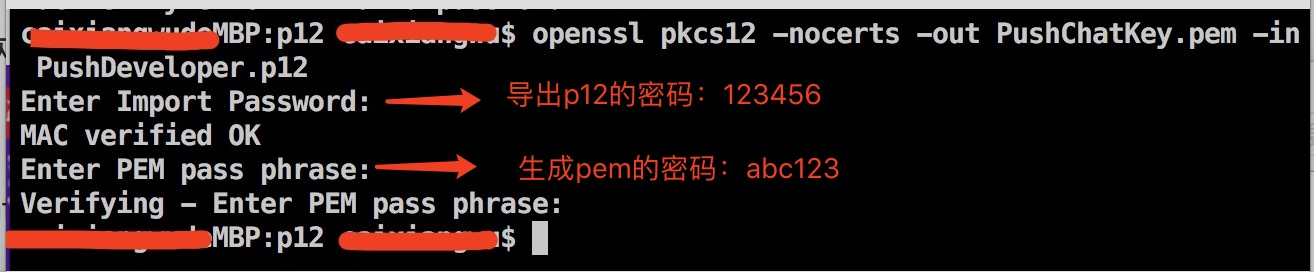
3.用certificate和PushChatKey.pem创建apns_developer_identity.p12文件(java后台配置用到的),需要输入密语:abc123
openssl pkcs12 -export -in PushChatCert.pem -inkey PushChatKey.pem -certfile CertificateSigningRequest.certSigningRequest -name "apns_developer_identity" -out apns_developer_identity.p12

ios工程测试
在AppDelegate里didFinishLaunchingWithOptions函数里写
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
// Override point for customization after application launch.
if ([[[UIDevice currentDevice] systemVersion] floatValue] >= 8.0){
[[UIApplication sharedApplication] registerUserNotificationSettings:[UIUserNotificationSettings
settingsForTypes:(UIUserNotificationTypeSound | UIUserNotificationTypeAlert | UIUserNotificationTypeBadge)
categories:nil]];
[[UIApplication sharedApplication] registerForRemoteNotifications];
}else{
//这里还是原来的代码
[[UIApplication sharedApplication] registerForRemoteNotificationTypes:
(UIUserNotificationTypeBadge | UIUserNotificationTypeSound | UIUserNotificationTypeAlert)];
}
return YES;
}
- (void)application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken{
NSLog(@"regisger success:%@", [[[[deviceToken description] stringByReplacingOccurrencesOfString:@"<" withString:@""] stringByReplacingOccurrencesOfString:@">"withString:@""]stringByReplacingOccurrencesOfString:@" "withString:@""]);
}
//前台收到消息
-(void)application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo{
// 处理推送消息
NSLog(@"userinfo:%@",userInfo);
NSLog(@"收到推送消息:%@",[[userInfo objectForKey:@"aps"] objectForKey:@"alert"]);
}
- (void)application:(UIApplication *)application didFailToRegisterForRemoteNotificationsWithError:(NSError *)error {
NSLog(@"Registfail%@",error);
NSLog(@"DeviceToken获取失败,原因:%@",error);
}
java后台代码
public static void main(String[] args) throws Exception{
int badge = 1; // 图标小红圈的数值
String sound = "default"; // 铃音
String msgCertificatePassword = "123456";//导出证书时设置的密码
//90fb73e94659a1822caa51ca079734de6a7e60e44f260a1bfd1326bb4648d734
String deviceToken = "a1f52f971ca720d6ffc98ce7212c74edf347234ab2cc34fefcb541314e2e13e1"; //手机设备token号
String message = "test push message to ios device11111111111";
List<String> tokens = new ArrayList<String>();
tokens.add(deviceToken);
//java必须要用导出p12文件 php的话是pem文件
String certificatePath = "/Users/xxxx/Desktop/p12/apns_developer_identity.p12";
boolean sendCount = true;
PushNotificationPayload payload = new PushNotificationPayload();
payload.addAlert(message); // 消息内容
payload.addBadge(badge);
//payload.addCustomAlertBody(msgEX);
if (null == sound || "".equals(sound)) {
payload.addSound(sound);
}
PushNotificationManager pushManager = new PushNotificationManager();
// false:表示的是产品测试推送服务 true:表示的是产品发布推送服务
pushManager.initializeConnection(new AppleNotificationServerBasicImpl(
certificatePath, msgCertificatePassword, false));
List<PushedNotification> notifications = new ArrayList<PushedNotification>();
// 开始推送消息
if (sendCount) {
Device device = new BasicDevice();
device.setToken(deviceToken);
PushedNotification notification = pushManager.sendNotification(
device, payload, true);
notifications.add(notification);
} else {
List<Device> devices = new ArrayList<Device>();
for (String token : tokens) {
devices.add(new BasicDevice(token));
}
notifications = pushManager.sendNotifications(payload, devices);
}
List<PushedNotification> failedNotification = PushedNotification
.findFailedNotifications(notifications);
List<PushedNotification> successfulNotification = PushedNotification
.findSuccessfulNotifications(notifications);
int failed = failedNotification.size();
int successful = successfulNotification.size();
System.out.println("zsl==========成功数:" + successful);
System.out.println("zsl==========失败数:" + failed);
pushManager.stopConnection();
System.out.println("zsl==========消息推送完毕");
}
您的pom.xml添加以下依赖项:
<dependency>
<groupId>com.github.fernandospr</groupId>
<artifactId>javapns-jdk16</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>