uni-app中使用地图显示当前的位置
我们现在的需求是,显示用户在地图上所处的位置。
有的小伙伴可能会说,这个是不是需要接入第三方的地图。
其实是不需要的,从目前这个需求来看。
我们只需要引入uni-app提供的内置组件 map 。
然后设置经纬度以及缩放大小就行
下面我们就来简单的演示一下
使用 map 组件显示某一个位置
<template>
<view>
<view class="page-body">
<view class="page-map-box">
<map style="width: 750rpx; height: 600rpx;"
:latitude="latitude"
:longitude="longitude"
:scale="scale"></map>
</view>
</view>
</view>
</template>
<script>
export default {
data(){
return {
msg:'12',
latitude: 30.572269,
longitude: 104.066541,
scale: 14,
}
}
}
</script>
<style scoped>
</style>
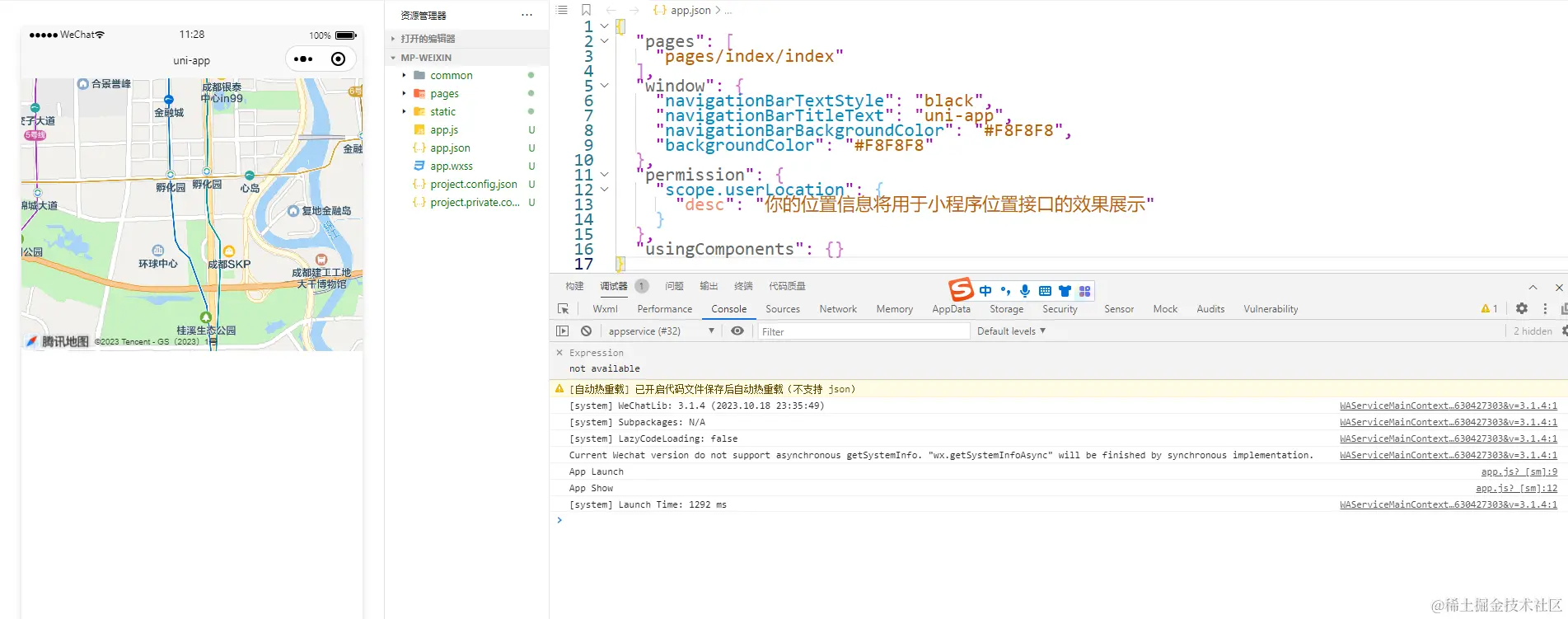
需要注意的点
第1点:<map> 组件的宽/高推荐写直接量,比如:750rpx,不要设置百分比值。
我尝试过写百分比也是可以正常显示的。
在写百分比的时候注意父盒子的宽高,如果父盒子没有设置宽高,会导致地图显示不出来。
既然官方说了不要写百分比咋们就不要写了。
第2点:谷歌地图使用 wgs84 坐标,其他地图使用 gcj02 坐标,用错坐标类型会显示偏移。
如何显示当前用户所在的位置
如果要显示用户当前所在位置。
我们需要借助一个api来获取。
uni.getLocation(options)获取当前的地理位置、速度
在获取之前,我们需要配置一下。
在HBuilder中的 uniapp项目下点击配置文件manifest.json。
选择微信小程序。填写【位置接口】描述。
或者在unpackage/dist/dev/mp-weixin/app.json中加入如下配置。
配置好后,在小程序的开发工具中看是否被正确编译了。
因为有些时候会出现丢死,此时需要再次重新写入
{
"pages": [
"pages/index/index"
],
"window": {
"navigationBarTextStyle": "black",
"navigationBarTitleText": "uni-app",
"navigationBarBackgroundColor": "#F8F8F8",
"backgroundColor": "#F8F8F8"
},
"permission": {
"scope.userLocation": {
"desc": "你的位置信息将用于小程序位置接口的效果展示"
}
},
"requiredPrivateInfos": ["getLocation", "chooseLocation"],
"usingComponents": {}
}
uni.getLocation({})获取当前的地理位置、速度
<template>
<view>
<view class="page-body">
<view class="page-map-box">
<map style="width: 750rpx; height: 600rpx;"
:latitude="latitude"
:longitude="longitude"
:scale="scale"></map>
</view>
<view class="btn-position">
<button type="default" @tap="getLocationTap">获取定位</button>
</view>
</view>
</view>
</template>
<script>
export default {
data(){
return {
latitude:'' ,
longitude: '',
scale: 14,
}
},
methods:{
getLocationTap(){
let that=this;
uni.getLocation({
type: 'gcj02',
success: function (res) {
console.log('经度:' + res.longitude);
console.log('纬度:' + res.latitude);
that.latitude = res.latitude
that.longitude= res.longitude
},
fail: function (res) {
console.log('获取位置信息失败', res);
}
})
}
}
}
</script>
<style scoped>
.page-map-box{
width: 750rpx; height: 600rpx;
}
.btn-position{
margin-top: 10rpx;
}
</style>
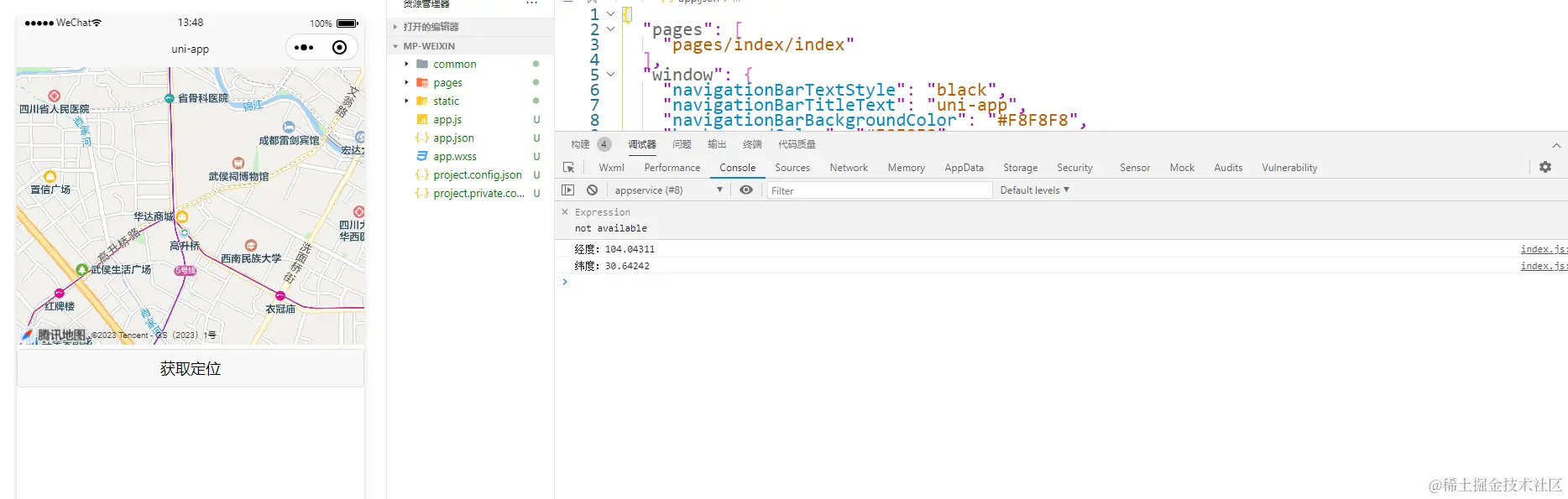
标记当前这个点
如果要标记当前的这个点,需要我们借助markers属性。
它接受一个数组,这个数组就是要标记的点。
其中id需要传递,否者会有提示信息。
iconPath是标记的图标地址。可以去阿里矢量图标库中下载一个图片。
width,height是这个图标的大小。
latitude,longitude是经纬度
<template>
<view>
<view class="page-body">
<view class="page-map-box">
<map style="width: 750rpx; height: 600rpx;"
:latitude="latitude"
:longitude="longitude"
:scale="scale"
:markers="markers"
></map>
</view>
<view class="btn-position">
<button type="default" @tap="getLocationTap">获取定位</button>
</view>
</view>
</view>
</template>
<script>
export default {
data(){
return {
latitude: '',
longitude: '',
scale: 14,
markers: [
{
id:1001,
width:50,
height:50,
latitude: this.latitude*1,
longitude: this.longitude*1,
iconPath: '../../static/weizhi.png',
},
],
}
},
methods:{
getLocationTap(){
let that=this;
uni.getLocation({
type: 'gcj02',
success: function (res) {
console.log('经度:' + res.longitude);
console.log('纬度:' + res.latitude);
that.latitude = res.latitude
that.longitude= res.longitude
},
fail: function (res) {
console.log('获取位置信息失败', res);
}
})
}
}
}
</script>
<style scoped>
.page-map-box{
width: 750rpx; height: 600rpx;
}
.btn-position{
margin-top: 10rpx;
}
</style>
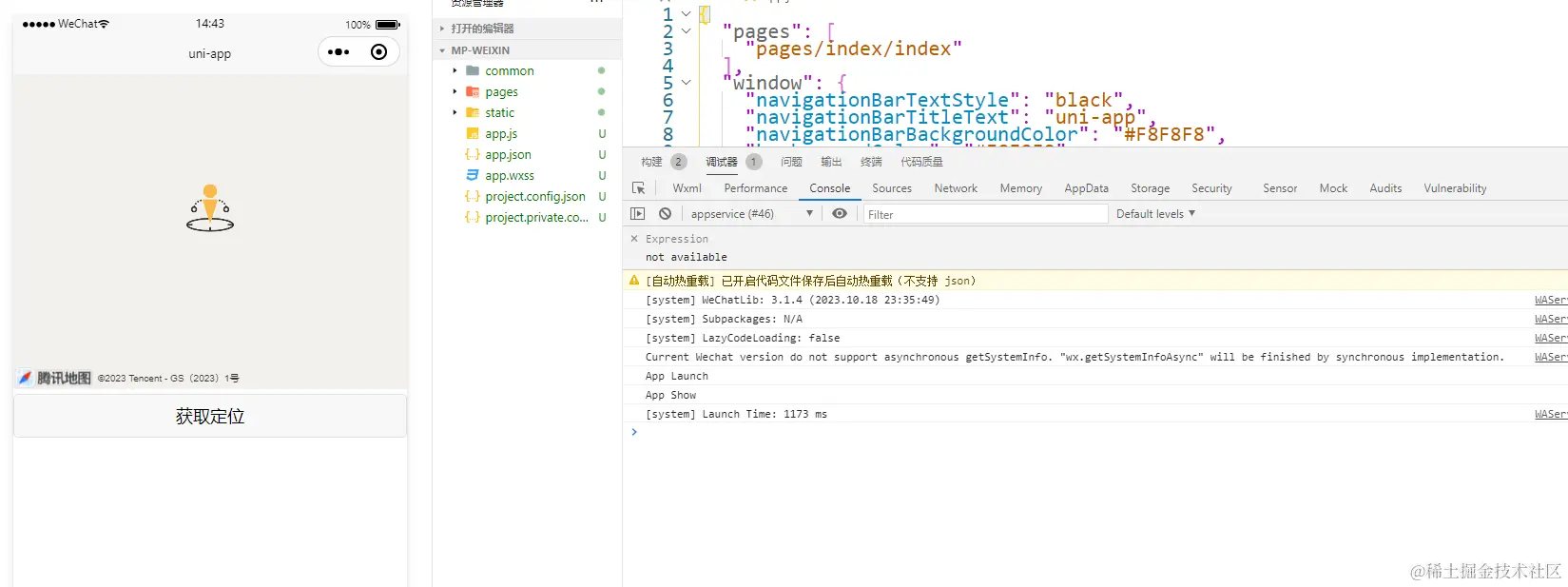