开启服务器
const http = require('http')
const app = http.createServer( (req,res)=>{
console.log( req.url )
console.log( decodeURI(req.url) )
} )
app.listen(3000,()=>{
console.log('服务器启动成功')
})
点击这里查看服务器
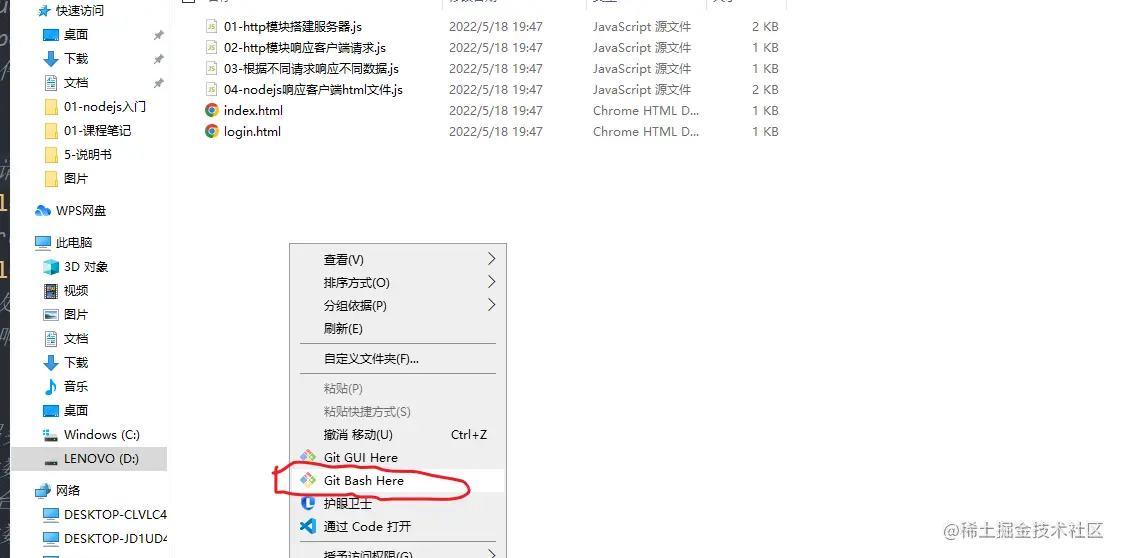
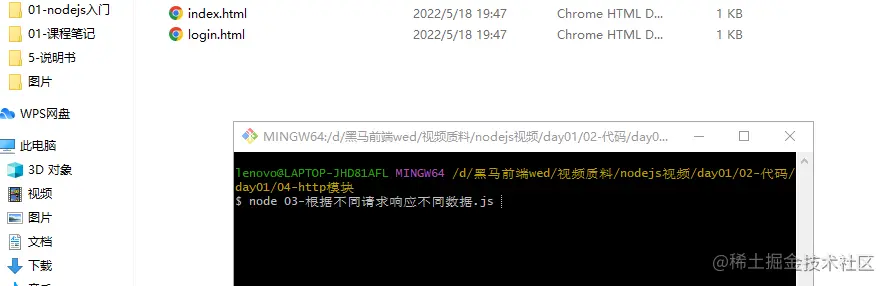
创建成功
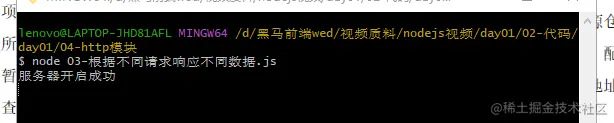
http模块响应客户端请求
const http = require('http')
const app = http.createServer((req, res) => {
console.log(decodeURI(req.url))
res.setHeader('Content-type', 'text/plain;charset=utf-8')
res.end('今天请看电影')
})
app.listen(3000, () => {
console.log('服务器开启成功');
})
必须先关闭之前的服务器然后按照上面的步骤进行开启服务器
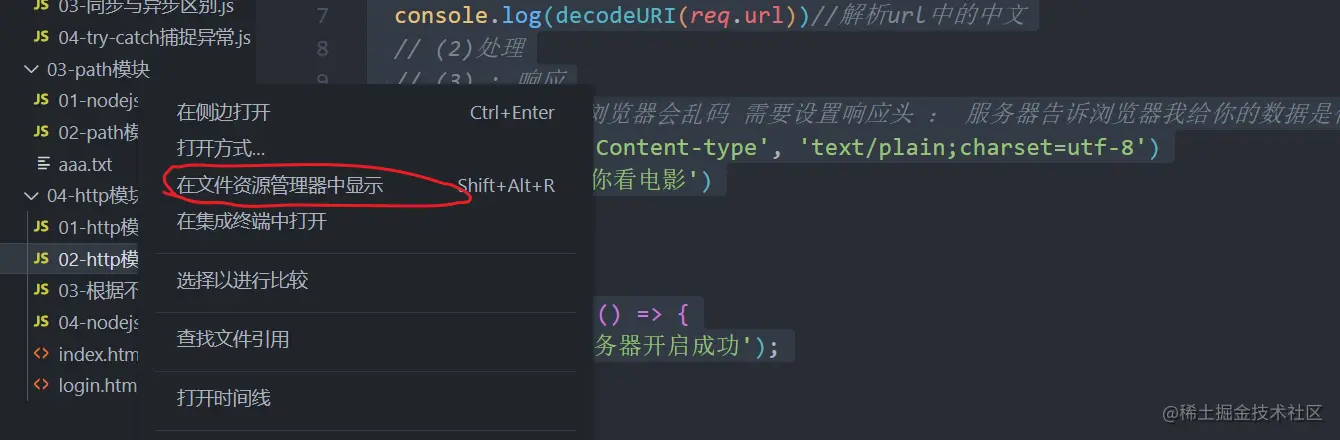
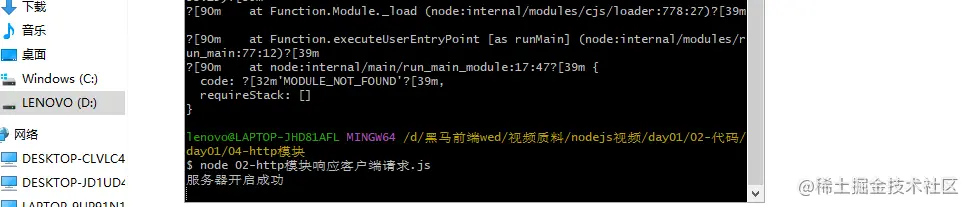
浏览器就可以访问了
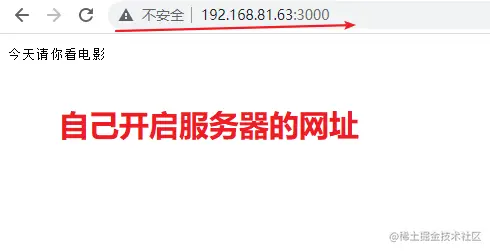
nodejs响应客户端html文件
准备两个跳转的html页面
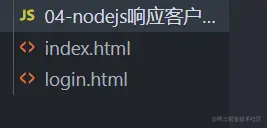
index页面
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
div{
height: 100px;
line-height: 100px;
font-size: 50px;
color: #fff;
text-align: center;
background: linear-gradient(to right,red,orange,yellow,green,cyan,blue,purple);
}
</style>
</head>
<body>
<div>这是首页</div>
</body>
</html>
login页面
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
这是登录页
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
<li>4</li>
<li>5</li>
<li>6</li>
</ul>
</body>
</html>
const http = require('http')
const fs = require('fs')
const path = require('path')
const app = http.createServer((req, res) => {
console.log(decodeURI(req.url))
if (req.url == '/') {
fs.readFile(path.join(__dirname, 'index.html'), (err, data) => {
if (err) {
throw err
} else {
res.end(data)
}
})
} else if (req.url == '/login') {
fs.readFile(path.join(__dirname, 'login.html'), (err, data) => {
if (err) {
throw err
} else {
res.end(data)
}
})
} else {
res.end('404 not found')
}
})
app.listen(3000, () => {
console.log('服务器开启成功');
})
进入首页效果

login页面
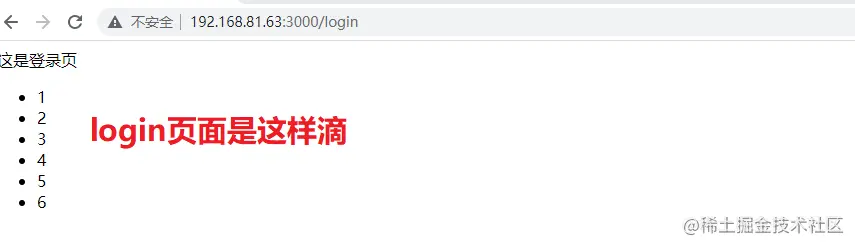