浅解Vue2生命周期
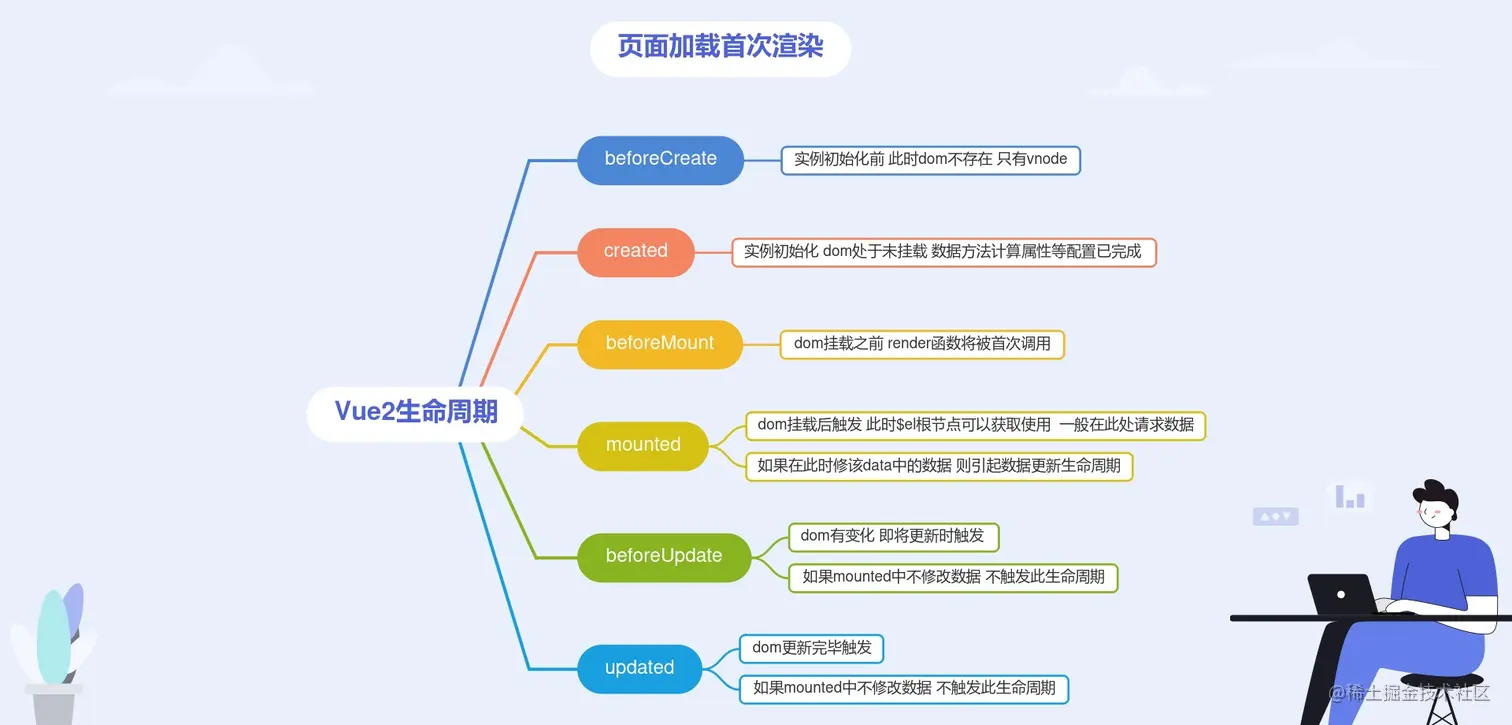
- 首页加载会触发哪几个生命周期
- beforeCreate
- created
- 实例初始化 dom处于未挂载 数据方法计算属性等配置已完成
- beforeMount
- mounted 此时$el 是根节点
- dom挂载后触发 此时$el根节点可以获取使用 一般在此处请求数据
- 怎么触发更新生命周期
- 在mounted中修改data里的数据 触发更新生命周期
- vue 全部的生命周期
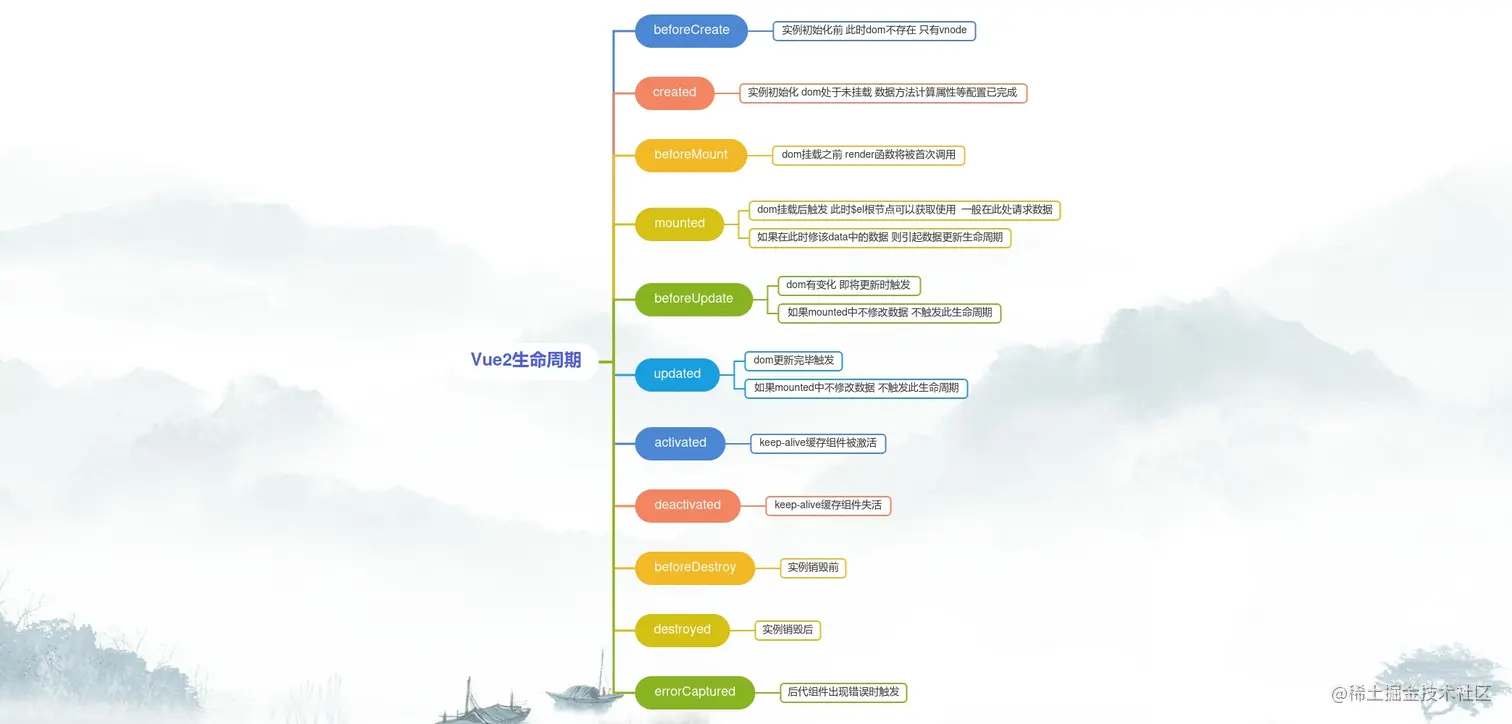
- 其他生命周期了解
- beforeUpdate
- updated
- activated
- deactivated
- beforeDestroy
- destroyed
- errorCaptured
- 生命周期小知识 ~
mounted() {
console.log('实例挂载后')
this.$nextTick(function () {
console.log('所有渲染结束')
})
},
updated() {
console.log('数据更新后')
this.$nextTick(function () {
console.log('重新渲染结束')
})
},
Vue2组件通信方式
props emit
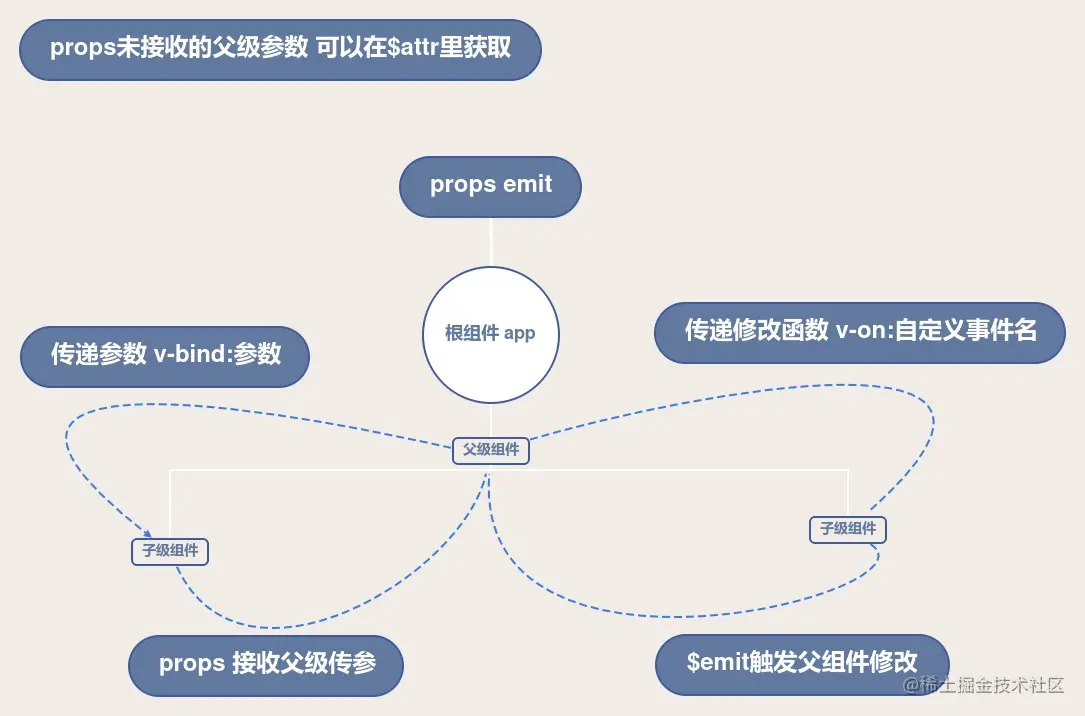
- 父组件通过v-bind指令向子级传递参数
- 子组件通过$emit触发父组件修改方法 接收两个参数 父组件修改方法名 修改后的值
<template name="component-name">
<div>
<p>我是父组件</p>
// 通过v-bind:names 向child组件传递参数
// 通过v-on:自定义事件传递修改方法
<child :names="message" @change="handleChange"></child>
</div>
</template>
<script>
import Child from './children.vue'
export default {
name:'Parent',
components: {
Child
},
data () {
return {
message:'这是父级传过来的'
}
},
methods: {
handleChange (e) {
this.message = e
}
}
}
</script>
<template name="component-name">
<div>
<p>我是子组件 {{names}}</p>
// 点击按钮 触发修改事件
<button @click="handleChange">按钮</button>
</div>
</template>
<script>
export default {
name:'Child',
props: {
names:{
type: String,
default:''
}
},
methods: {
handleChange () {
this.$emit('change','修改后的names')
}
}
}
</script>
event bus
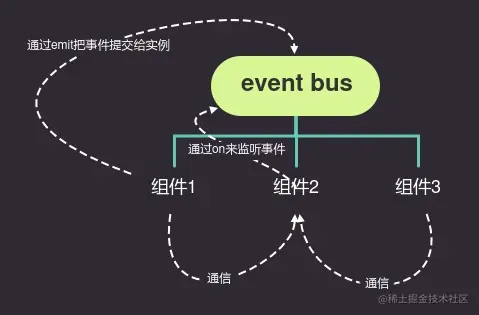
Vue.prototype.$bus = new Vue()
<template name="component-name">
<div>
<p>我是父组件 {{msg}}</p>
</div>
</template>
<script>
export default {
name:'Parent',
data () {
return {
msg: ''
}
},
mounted() {
this.$bus.$on('msg', (msg)=> {
this.msg = msg
})
}
}
</script>
<template name="component-name">
<div>
<p>这是第二个组件</p>
<button @click="handleClick">点击发送信息</button>
</div>
</template>
<script>
export default {
name:'childs',
methods: {
handleClick () {
this.$bus.$emit('msg','来自childs的消息')
}
}
}
</script>
$root
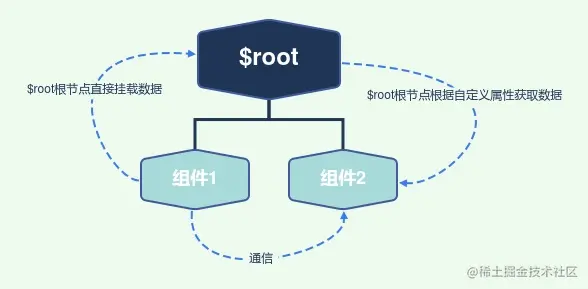
- $root传递数据本质上是在实例上挂载自定义的数据
<template name="component-name">
<div>
<button @click="handleChange">按钮</button>
</div>
</template>
<script>
export default {
name:'Child',
methods: {
handleChange () {
this.$root._cb = '根组件上的数据'
}
}
}
</script>
<template name="component-name">
<div>
<p>我是父组件 {{msg}}</p>
</div>
</template>
<script>
export default {
name:'Parent',
mounted() {
console.log(this._cd)
},
}
</script>
$parent
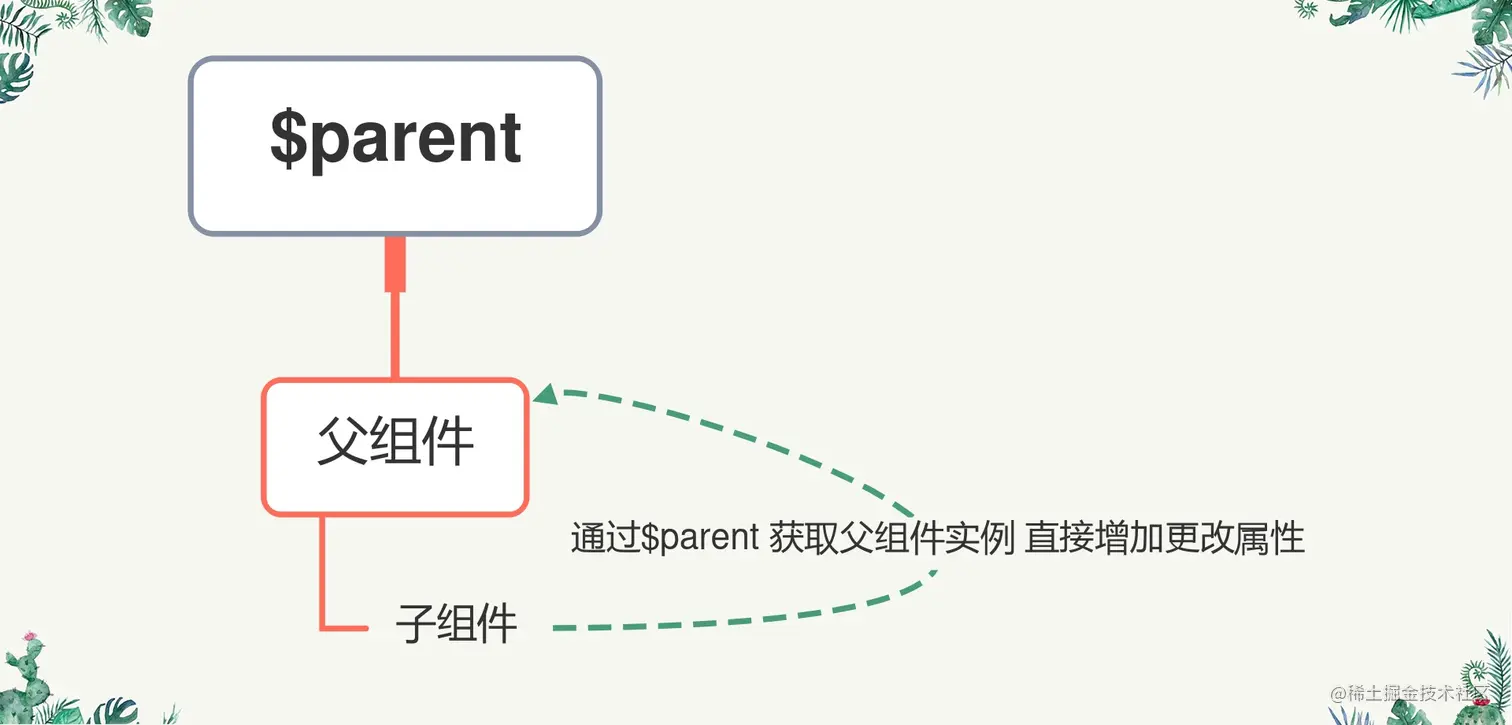
<template name="component-name">
<div>
<p>我是子组件 {{names}}</p>
<button @click="handleChange">按钮</button>
</div>
</template>
<script>
export default {
name:'Child',
methods: {
handleChange () {
this.$parent.$data.msg = 1323232
}
}
}
</script>
<template name="component-name">
<div>
<p>我是父组件 {{msg}}</p>
<child :names="message" @change="handleCHnage"></child>
</div>
</template>
<script>
import Child from './children.vue'
export default {
name:'Parent',
components: {
Child
},
data () {
return {
msg: ''
}
}
}
</script>
$children
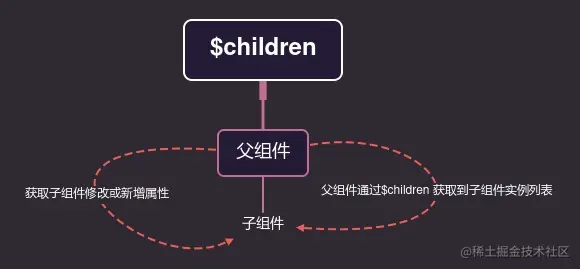
<template name="component-name">
<div>
<p>我是父组件</p>
<child></child>
</div>
</template>
<script>
import Child from './children.vue'
export default {
name:'Parent',
components: {
Child
},
mounted() {
this.$children[0].arg = 233232
}
}
</script>
<template name="component-name">
<div>
<p>我是子组件 {{arg}}</p>
<button @click="handleChange">按钮</button>
</div>
</template>
<script>
export default {
name:'Child',
data () {
return {
arg: ''
}
}
}
</script>
<style scoped>
</style>
porvide / inject
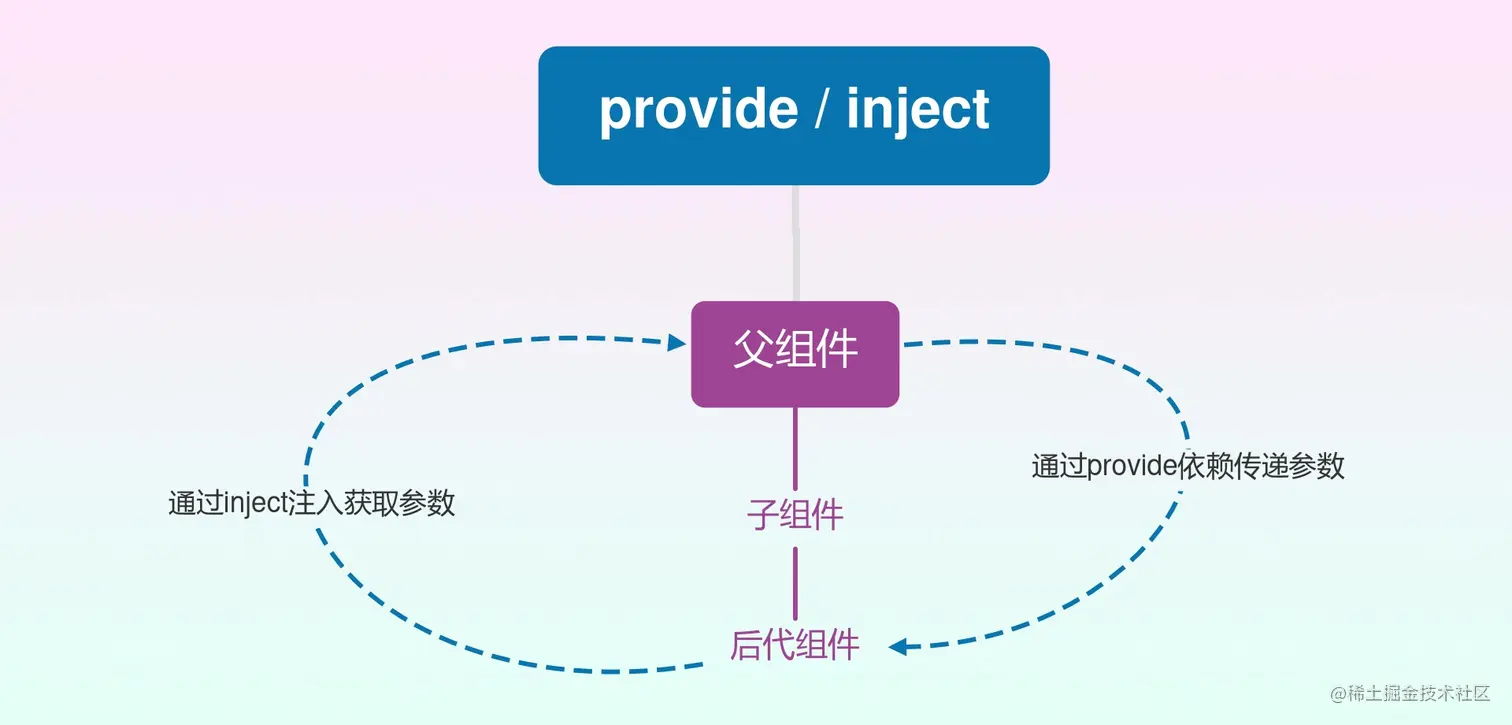
- provide依赖在父组件定义 传向后代组件
- 后代组件通过inject注入获取
- 父组件参数不可更改 如需更改可以向后代传递修改参数的函数
<template name="component-name">
<div>
<p>我是父组件 {{msg}}</p>
<child></child>
</div>
</template>
<script>
import Child from './children.vue'
export default {
name:'Parent',
components: {
Child
},
provide: {
query: 'provide 参数'
}
}
</script>
<template name="component-name">
<div>
<p>我是子组件{{query}}</p>
<button @click="handleChange">按钮</button>
</div>
</template>
<script>
export default {
name:'Child',
inject: ['query']
</script>
结语