题目
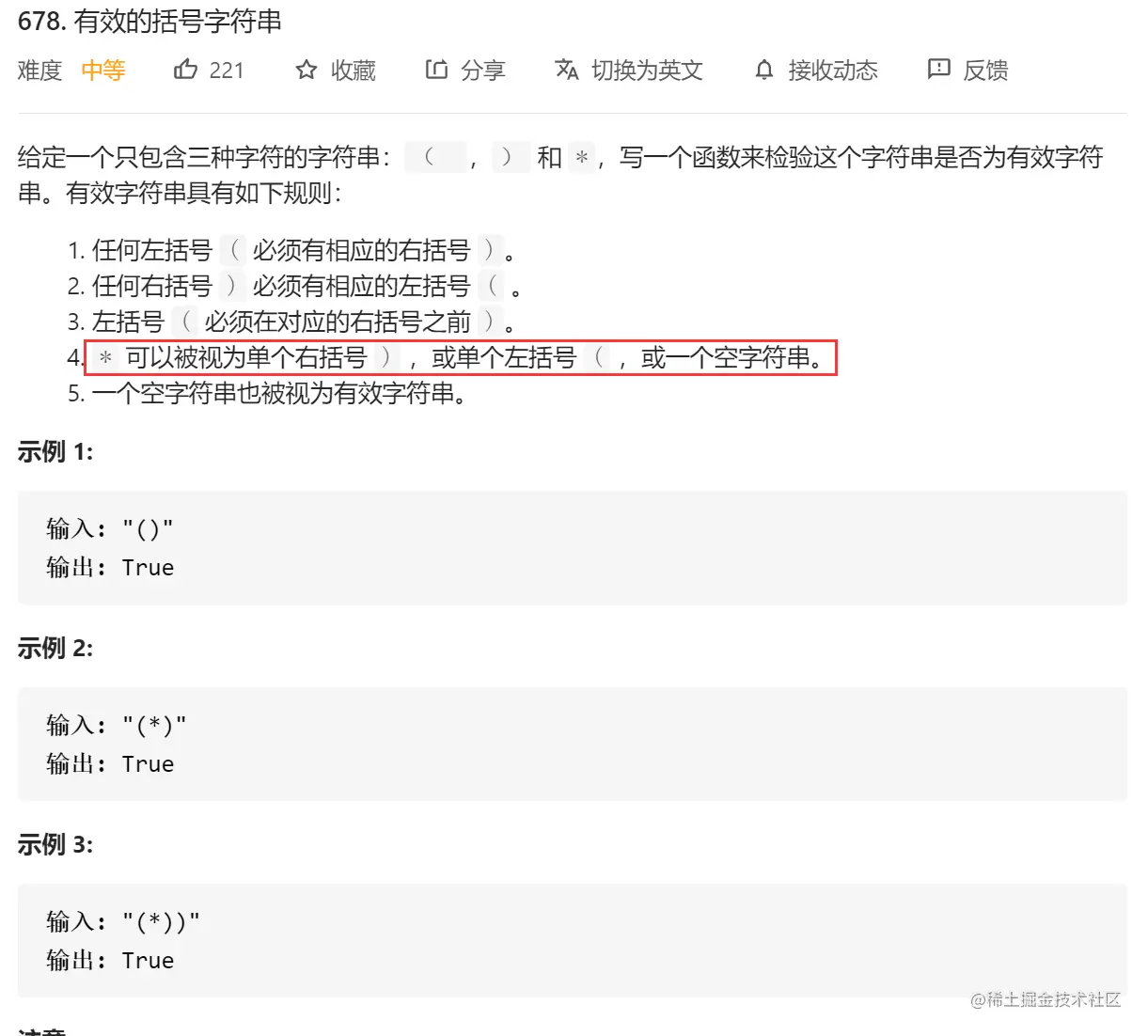
思路
- 两个栈分别将"("和"*"的序号压入栈中,
- 每次遇到右括号,首先检测左括号栈中是否为空,再检测star栈,不为空则弹出元素。
- 空的话直接false
- 最后考虑left和star栈可能存在元素,判断此时栈中元素的序号大小
- 如果left栈顶的序号大于star栈顶序号,则表明 存在 "*(" 此种情况,表明有(无法被抵消掉直接false
- 如果最后left栈还有剩余,说明还有(无法被抵消,false
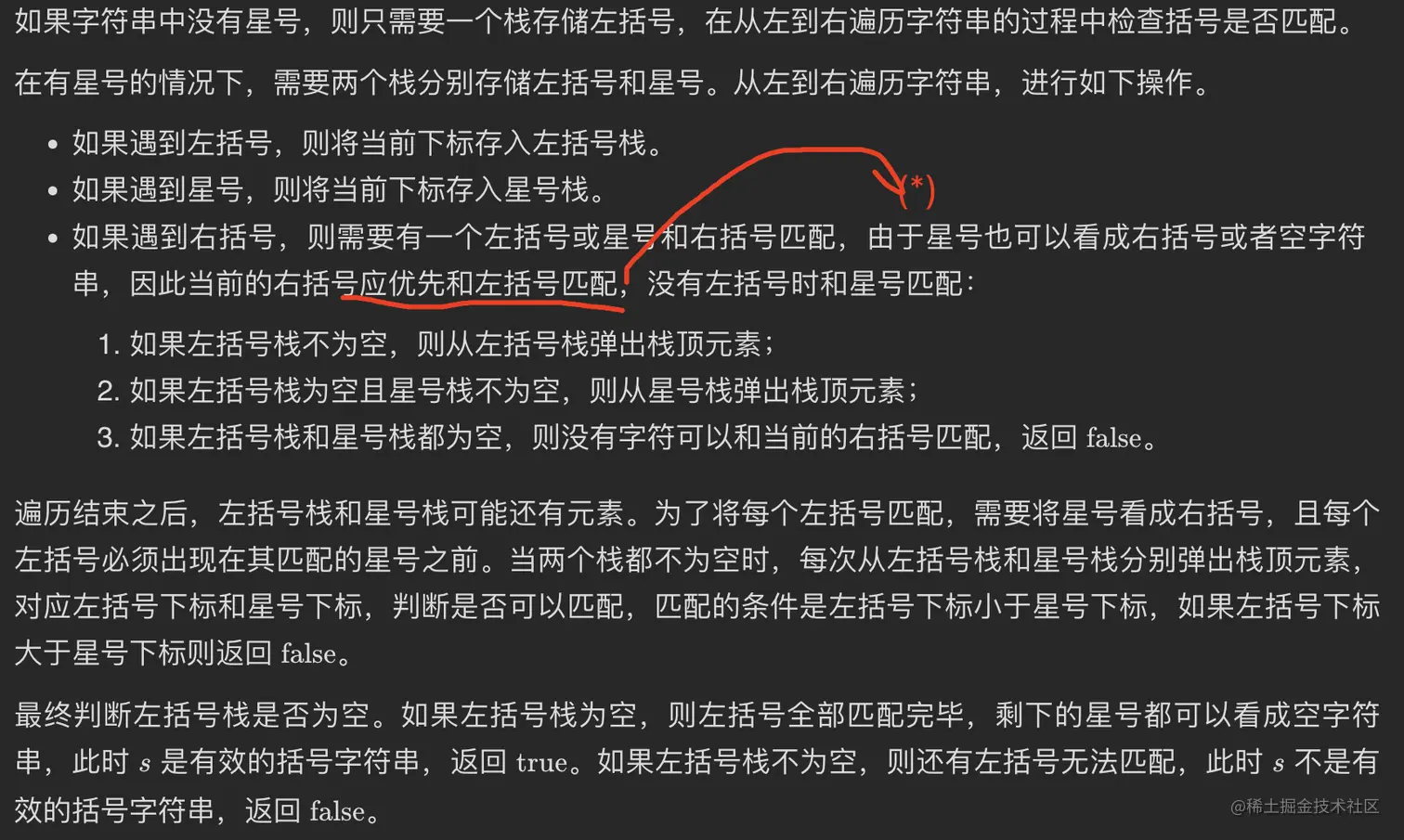
代码
class Solution {
public boolean checkValidString(String s) {
Stack<Integer> left = new Stack<>();
Stack<Integer> star = new Stack<>();
for (int i = 0; i < s.length(); i++) {
if (s.charAt(i) == '(') {
left.push(i);
} else if (s.charAt(i) == '*') {
star.push(i);
} else {
if (left.isEmpty() && star.isEmpty()) {
return false;
}
if (!left.isEmpty()) {
left.pop();
} else if (!star.isEmpty()) {
star.pop();
}
}
}
while (!left.isEmpty() && !star.isEmpty()) {
if (left.pop() > star.pop()) {
return false;
}
}
return left.isEmpty();
}
}