读取json文件内容,转化为list,再放入map中
import 'package:flutter/services.dart' show rootBundle;
Future<Map<int, Map<String, dynamic>>> loadData(String key) async {
final list = jsonDecode(await rootBundle.loadString(key)) as List;
return Map.fromEntries(list.map((v) => MapEntry(v['id'], v)));
}
延迟加载
final response = await Future.delayed(
Duration(
milliseconds: 500 + _random.nextInt(500),
),
() {
_userId = 1;
return {
'user': _users[_userId],
};
},
);
界面模版
import 'package:flutter/material.dart';
class LoginPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('标题'),
),
body: _Body(),
);
}
}
class _Body extends StatefulWidget {
@override
State<StatefulWidget> createState() => _BodyState();
}
class _BodyState extends State<_Body> {
@override
Widget build(BuildContext context) {
return GestureDetector();
}
}
异步loading
Future<T> doWithLoading<T>(Future<T> Function() doSomething) async {
final cancelLoading = showLoading();
T result;
try {
result = await doSomething();
} on Exception catch (e) {
handleException(e);
} finally {
cancelLoading();
}
return result;
}
字体样式
TextStyle buttonStyle(BuildContext context) => Theme.of(context)
.primaryTextTheme
.button
.copyWith(fontSize: 16, letterSpacing: 32);
常用布局
return GestureDetector(
onTap: () => FocusScope.of(context).unfocus(),
child: ListView(
children: <Widget>[
//...
],
),
);
控件
GestureDetector
ListView
Card
Padding
EdgeInsets.all
Column
TextFormField
TextFormField
Container
Row
Text
FlatButton
Text
HomePage
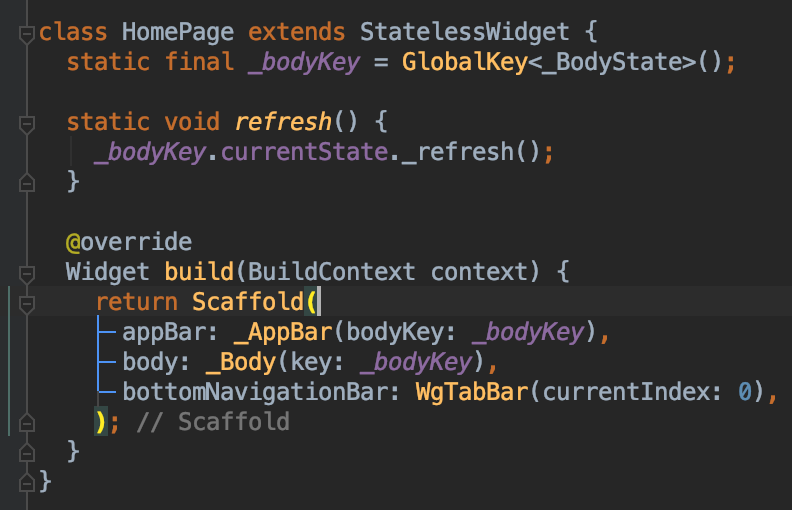
StoreConnector
distinct
页面跳转
注册路由 main.dart
MaterialApp(
routes:{
'xx_page': (context) => XXPage(),
...
}
)
点击跳转 xx.dart
FlatButton(
onPressed: () {
// 可通过arguments传递参数
Navigator.pushNamed(context, 'xx_page', arguments: {'data': data});
}
)
Row 循环
Row(children: strings.map((item) => Text(item)).toList());
热更新
图片字体资源
环境变量
export PUB_HOSTED_URL=https://pub.flutter-io.cn
export FLUTTER_STORAGE_BASE_URL=https://storage.flutter-io.cn
export PATH=`pwd`/flutter/bin:$PATH
export PATH=$HOME/flutter/bin:$PATH
echo $PATH
文件名
Android 是在 AndroidManifest.xml 中修改;iOS 则是在 Info.plist 中修改的;
Android8.0 适配解决页面跳转过程出现短暂黑屏的问题
windowBackground
在 Android8.0 中 overridePendingTransition 若设为进入和进出为 0 时会出现黑屏,解决方案是:将 0 换为固定的进入和退出的 anim 即可,如下:
// 原来
overridePendingTransition(R.anim.slide_right_in, 0);
// 替换为
overridePendingTransition(R.anim.slide_right_in, R.anim.slide_right_out);
// 原来
overridePendingTransition(0, R.anim.slide_right_out);
// 替换为
overridePendingTransition(R.anim.slide_right_in, R.anim.slide_right_out);