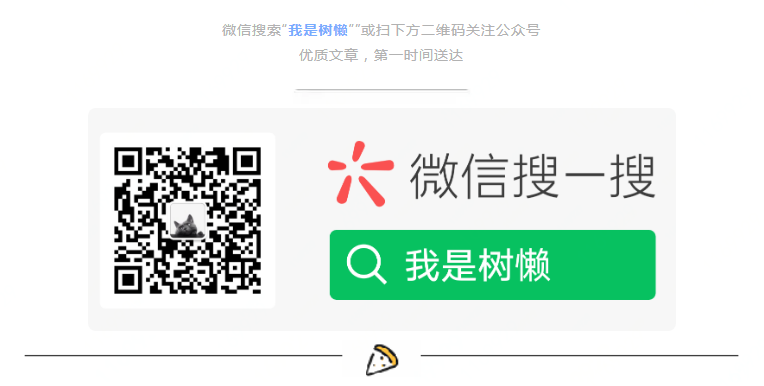
命令模式
命令模式属于行为型模式
定义:将一个请求封装成一个对象,从而让你使用不同的请求把客户端参数化,对请求排队或者记录请求日志,可以提供命令的撤销和恢复功能
模式类图
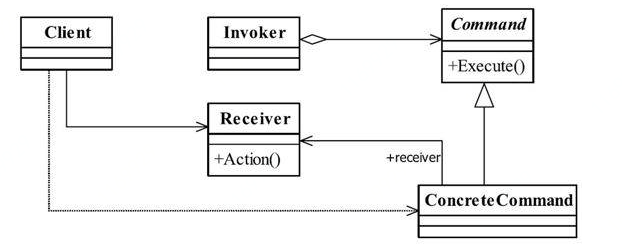
角色:
- 接受者:
Receiver
最后的执行者,具体的业务逻辑 - 命令接口:
Command
需要执行的所有命令都在这里声明 - 具体命令:
ConcreteCommand
实现Command
接口 - 调用者:
Invoker
接收到命令,并执行命令,命令中调用接受者
优点
- 降低类之间解耦,调用者与接受者之间没有任何依赖关系
- 扩展性高,非常容易地扩展
Command
类的子类
缺点
如果命令比较多,Command
类的子类就会变得非常的多
模式代码实现
源码地址:https://github.com/mingyuHub/design-patterns
接受者有两个方法,打游戏和学习,通过实现命令接口写了两个具体的命令实现类,分别代表玩游戏命令和学习命令,通过调用者去调用不同的命令
接受者
/**
* @author: chenmingyu
* @date: 2019/3/25 10:48
* @description: 接受者角色
*/
public class Receive {
public void play(){
System.out.println("打游戏吧");
}
public void study(){
System.out.println("要学习了");
}
}
命令接口
/**
* @author: chenmingyu
* @date: 2019/3/25 10:51
* @description: 命令接口
*/
public interface Commandable {
/**
* 命令执行接口
*/
void execute();
}
玩游戏命令
持有receive
实例
/**
* @author: chenmingyu
* @date: 2019/3/25 10:52
* @description: 玩游戏命令
*/
public class GameCommand implements Commandable{
private Receive receive;
public GameCommand(Receive receive) {
this.receive = receive;
}
@Override
public void execute() {
this.receive.play();
}
}
学习命令
持有receive
实例
/**
* @author: chenmingyu
* @date: 2019/3/25 10:54
* @description: 学习命令
*/
public class StudyCommand implements Commandable {
private Receive receive;
public StudyCommand(Receive receive) {
this.receive = receive;
}
@Override
public void execute() {
this.receive.study();
}
}
调用者
持有Commandable
的实现类的实例
/**
* @author: chenmingyu
* @date: 2019/3/25 10:51
* @description: 调用者
*/
@Data
public class Invoker {
/**
* 持有Commandable的实现类的实例
*/
private Commandable commandable;
/**
* 执行命令
*/
public void action(){
this.commandable.execute();
}
}
验证
public static void main(String[] args) {
Invoker invoker = new Invoker();
Receive receive = new Receive();
Commandable gameCommand = new GameCommand(receive);
invoker.setCommandable(gameCommand);
invoker.action();
Commandable studyCommand = new StudyCommand(receive);
invoker.setCommandable(studyCommand);
invoker.action();
}
输出
打游戏吧
要学习了