目录
- 尝鲜Vue3之一:浏览器中如何断点调试Vue3 源码
- 尝鲜Vue3之二:最重要的变化CompositionApi
- 尝鲜Vue3之三:CompositionAPI小结和包装对象
- 尝鲜Vue3之四:如何运行Jest单元测试
- 尝鲜Vue3之五:源码结构
- 尝鲜Vue3之六:响应式原理的革新 - Vue2、Vue3实现对比
克隆Vue3源码
Vue源码位置
git clone git@github.com:vuejs/vue-next.git
安装依赖编译源码
进入代码目录并安装依赖
yarn
yarn dev

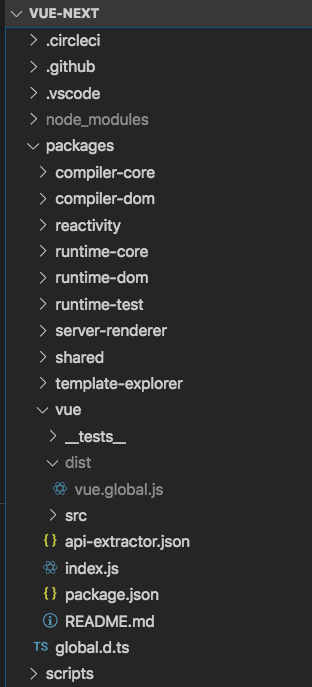
测试API
- 做一个简单的Helloworld测试 我们就先试试原有的vue2 的Api还可不可以使用,其实vue3中提倡使用composite-api也就是函数定义风格的api,原有vue偏向于配置配置风格我们把它统称为options风格 我们在根目录上创建文件夹
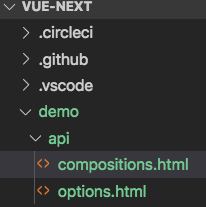
options.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<script src="../../packages/vue/dist/vue.global.js"></script>
</head>
<body>
<div id='app'></div>
<script>
const App = {
template: `
<button @click='click'>{{message}}</button>
`,
data() {
return {
message: 'Hello Vue 3!!'
}
},
methods: {
click() {
console.log('click ....', this.message)
this.message = this.message.split('').reverse().join('')
}
}
}
let vm = Vue.createApp().mount(App, '#app')
// console.log(vm)
</script>
</body>
</html>
其实这个代码就是vue2官网的代码 拿过来可以正常运行 我们再试验一下vue3的composition api
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<script src="../../packages/vue/dist/vue.global.js"></script>
</head>
<body>
<div id="app"></div>
<script>
const {
createApp,
reactive,
computed,
effect
} = Vue
const MyComponent = {
template: `
<button @click="click">
{{ state.message }}
</button>
`,
setup() {
const state = reactive({
message:'Hello Vue 3!!'
})
effect(() => {
console.log('state change ', state.message)
})
function click() {
state.message = state.message.split('').reverse().join('')
}
return {
state,
click
}
}
}
createApp().mount(MyComponent, '#app')
</script>
</body>
</html>
直接通过浏览器就可以打开本地文件
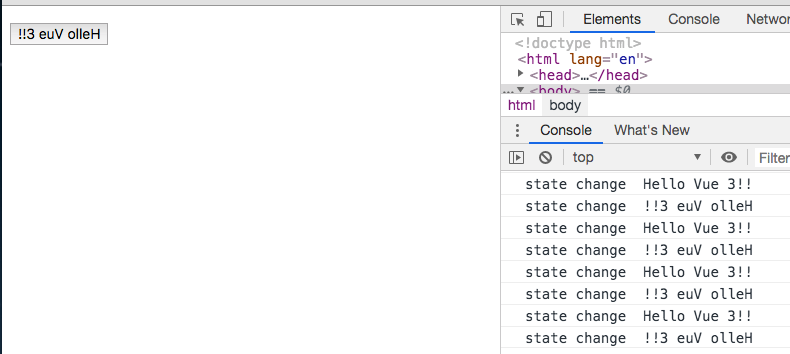
可以试一下点击的效果 接下来如果你要debug一下源码的时候你会发现代码是经过打包的无法直接在源码上打断点调试
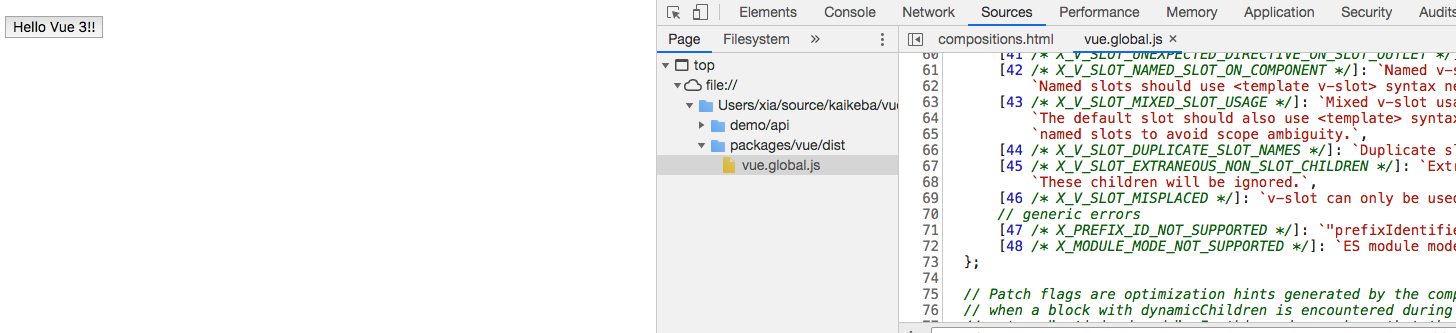
添加SourceMap文件
为了能在浏览器中看到源码 并能够断点调试 需要再打包后代码中添加sourcemap
- rollup.config.js
// rollup.config.js line:104左右
output.sourcemap = true
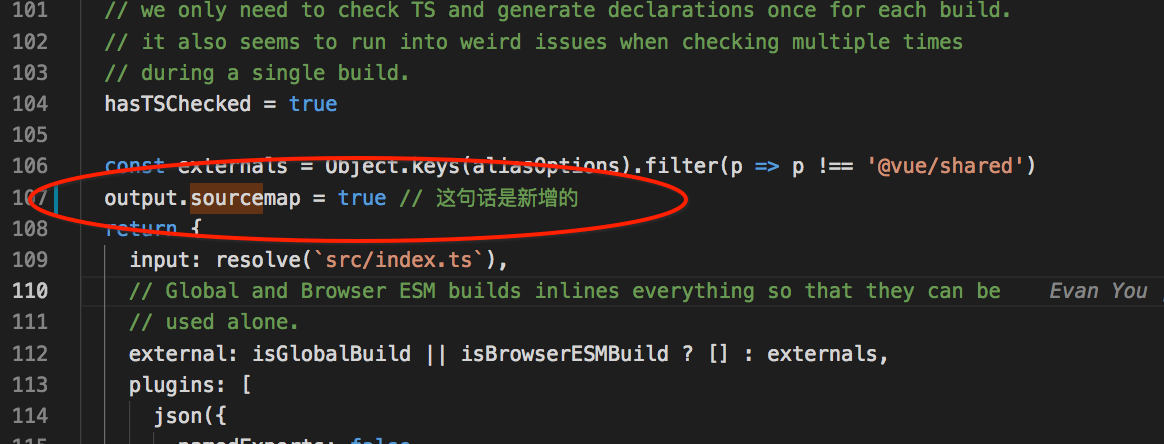
- 在tsconfig.json中配置sourcemap输出
// tsconfig.json
{
"compilerOptions": {
"baseUrl": ".",
"outDir": "dist",
"sourceMap": true, // ----------这里这里---------
"target": "esnext",
"module": "esnext",
"moduleResolution": "node",
"allowJs": false,
"noUnusedLocals": true,
"strictNullChecks": true,
"noImplicitAny": true,
"noImplicitThis": true,
"experimentalDecorators": true,
"resolveJsonModule": true,
"esModuleInterop": true,
"removeComments": false,
"jsx": "react",
"lib": ["esnext", "dom"],
"types": ["jest", "node"],
"rootDir": ".",
"paths": {
"@vue/shared": ["packages/shared/src"],
"@vue/runtime-core": ["packages/runtime-core/src"],
"@vue/runtime-dom": ["packages/runtime-dom/src"],
"@vue/runtime-test": ["packages/runtime-test/src"],
"@vue/reactivity": ["packages/reactivity/src"],
"@vue/compiler-core": ["packages/compiler-core/src"],
"@vue/compiler-dom": ["packages/compiler-dom/src"],
"@vue/server-renderer": ["packages/server-renderer/src"],
"@vue/template-explorer": ["packages/template-explorer/src"]
}
},
"include": [
"packages/global.d.ts",
"packages/runtime-dom/jsx.d.ts",
"packages/*/src",
"packages/*/__tests__"
]
}
再次运行
yarn dev
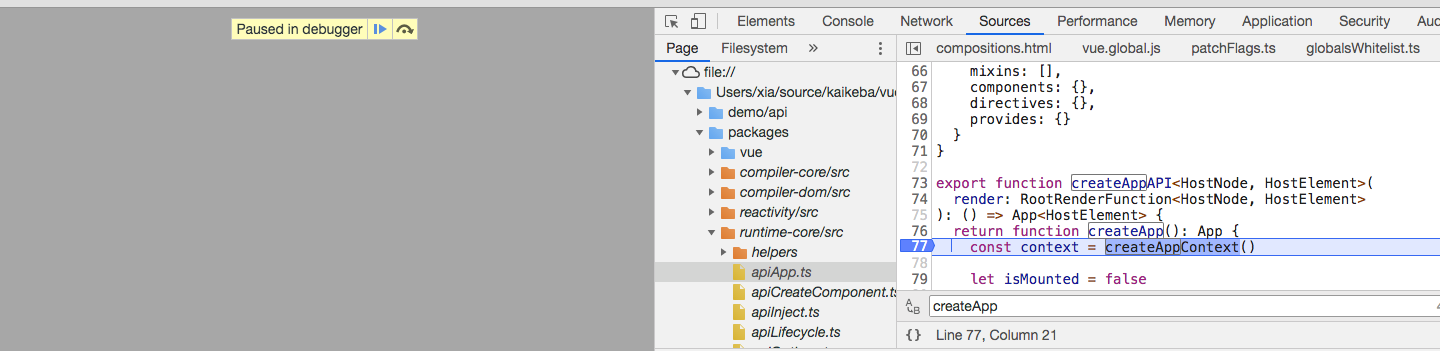
好了 同学们可以快乐的玩耍了。
不过要想真正了解源码 需要很多基础知识的储备譬如 ES6的proxy reflect、monorepo风格代码组织方式 后续文章中会一一解析3