本文是对 Swift Algorithm Club 翻译的一篇文章。
Swift Algorithm Club是 raywenderlich.com网站出品的用Swift实现算法和数据结构的开源项目,目前在GitHub上有18000+⭐️,我初略统计了一下,大概有一百左右个的算法和数据结构,基本上常见的都包含了,是iOSer学习算法和数据结构不错的资源。
🐙andyRon/swift-algorithm-club-cn是我对Swift Algorithm Club,边学习边翻译的项目。由于能力有限,如发现错误或翻译不妥,请指正,欢迎pull request。也欢迎有兴趣、有时间的小伙伴一起参与翻译和学习🤓。当然也欢迎加⭐️,🤩🤩🤩🤨🤪。
本文的翻译原文和代码可以查看🐙swift-algorithm-club-cn/Shortest Path(Unweighted)
最短路径算法(Shortest Path(Unweighted Graph))
目标:找到图中从一个节点到另一个节点的最短路径。
假设我们以下图为例:
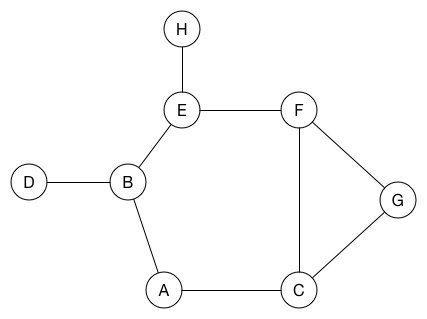
我们可能想知道从节点A
到节点F
的最短路径是什么。
如果图是未加权的,那么找到最短路径很容易:我们可以使用广度优先搜索算法。 对于加权图,我们可以使用Dijkstra算法。
未加权图:广度优先搜索
广度优先搜索是遍历树或图数据结构的方法。 它从源节点开始,在移动到下一级邻居之前首先探索直接邻居节点。 方便的副作用是,它会自动计算源节点与树或图中其他每个节点之间的最短路径。
广度优先搜索的结果可以用树表示:
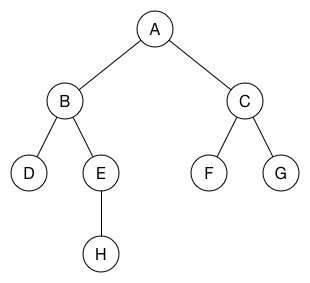
树的根节点是广度优先搜索开始的节点。 为了找到从节点A
到任何其他节点的距离,我们只计算树中边的数目。 所以我们发现A
和F
之间的最短路径是2.树不仅告诉你路径有多长,而且还告诉你如何实际从A
到F
(或者任何一个其他节点)。
让我们将广度优先搜索付诸实践,并计算从A
到所有其他节点的最短路径。 我们从源节点A
开始,并将其添加到队列中,距离为0
。
queue.enqueue(element: A)
A.distance = 0
队列现在是[A]
。 我们将A
出列并将其两个直接邻居节点B
和C
入列,并设置距离1
。
queue.dequeue() // A
queue.enqueue(element: B)
B.distance = A.distance + 1 // result: 1
queue.enqueue(element: C)
C.distance = A.distance + 1 // result: 1
队列现在是[B, C]
。 将B
出列,并将B
的邻居节点D
和E
入列,距离为2
。
queue.dequeue() // B
queue.enqueue(element: D)
D.distance = B.distance + 1 // result: 2
queue.enqueue(element: E)
E.distance = B.distance + 1 // result: 2
队列现在是[C, D, E]
。 将C
出列并将C
的邻居节点F
和G
入队,距离为2
。
queue.dequeue() // C
queue.enqueue(element: F)
F.distance = C.distance + 1 // result: 2
queue.enqueue(element: G)
G.distance = C.distance + 1 // result: 2
这么一直持续到队列为空,同时我们访问了所有节点。 每次我们发现一个新节点时,它会获得其父节点的distance
加1.正如您所看到的,这正是广度优先搜索算法的作用, 除此之外,我们现在还知道距离寻找的路径。
这是代码:
func breadthFirstSearchShortestPath(graph: Graph, source: Node) -> Graph {
let shortestPathGraph = graph.duplicate()
var queue = Queue<Node>()
let sourceInShortestPathsGraph = shortestPathGraph.findNodeWithLabel(label: source.label)
queue.enqueue(element: sourceInShortestPathsGraph)
sourceInShortestPathsGraph.distance = 0
while let current = queue.dequeue() {
for edge in current.neighbors {
let neighborNode = edge.neighbor
if !neighborNode.hasDistance {
queue.enqueue(element: neighborNode)
neighborNode.distance = current.distance! + 1
}
}
}
return shortestPathGraph
}
在playground中进行测试:
let shortestPathGraph = breadthFirstSearchShortestPath(graph: graph, source: nodeA)
print(shortestPathGraph.nodes)
输出结果:
Node(label: a, distance: 0), Node(label: b, distance: 1), Node(label: c, distance: 1),
Node(label: d, distance: 2), Node(label: e, distance: 2), Node(label: f, distance: 2),
Node(label: g, distance: 2), Node(label: h, distance: 3)
**注意:**这个版本的
breadthFirstSearchShortestPath()
实际上并不生成树,它只计算距离。 有关如何通过去除边缘将图转换为树,请参见最小生成树。
作者:Chris Pilcher,Matthijs Hollemans
翻译:Andy Ron
校对:Andy Ron