目录
- 原理
- 原理验证一(表面验证)
- 原理验证二 (使用 runtime)
- 原理验证三(底层验证)
- 如何调用原类方法
一 原理
平常开发中,我们经常给某些类添加分类,新增新的方法。
原理
- Category编译之后的底层结构是
struct category_t
,里面存储着分类的对象方法、类方法、属性、协议信息 - 在程序运行的时候,runtime会将Category的数据,合并到类信息中(类对象、元类对象中)
Category的底层结构
struct _category_t {
const char *name;
struct _class_t *cls;
const struct _method_list_t *instance_methods; // 对象方法列表
const struct _method_list_t *class_methods; // 类方法列表
const struct _protocol_list_t *protocols; // 协议列表
const struct _prop_list_t *properties; // 属性列表
};
Category的加载处理过程
- 1.通过Runtime加载某个类的所有Category数据
- 2.把所有Category的方法、属性、协议数据,合并到一个大数组中,后面参与编译的Category数据,会在数组的前面
- 3.将合并后的分类数据(方法、属性、协议),插入到类原来数据的前面
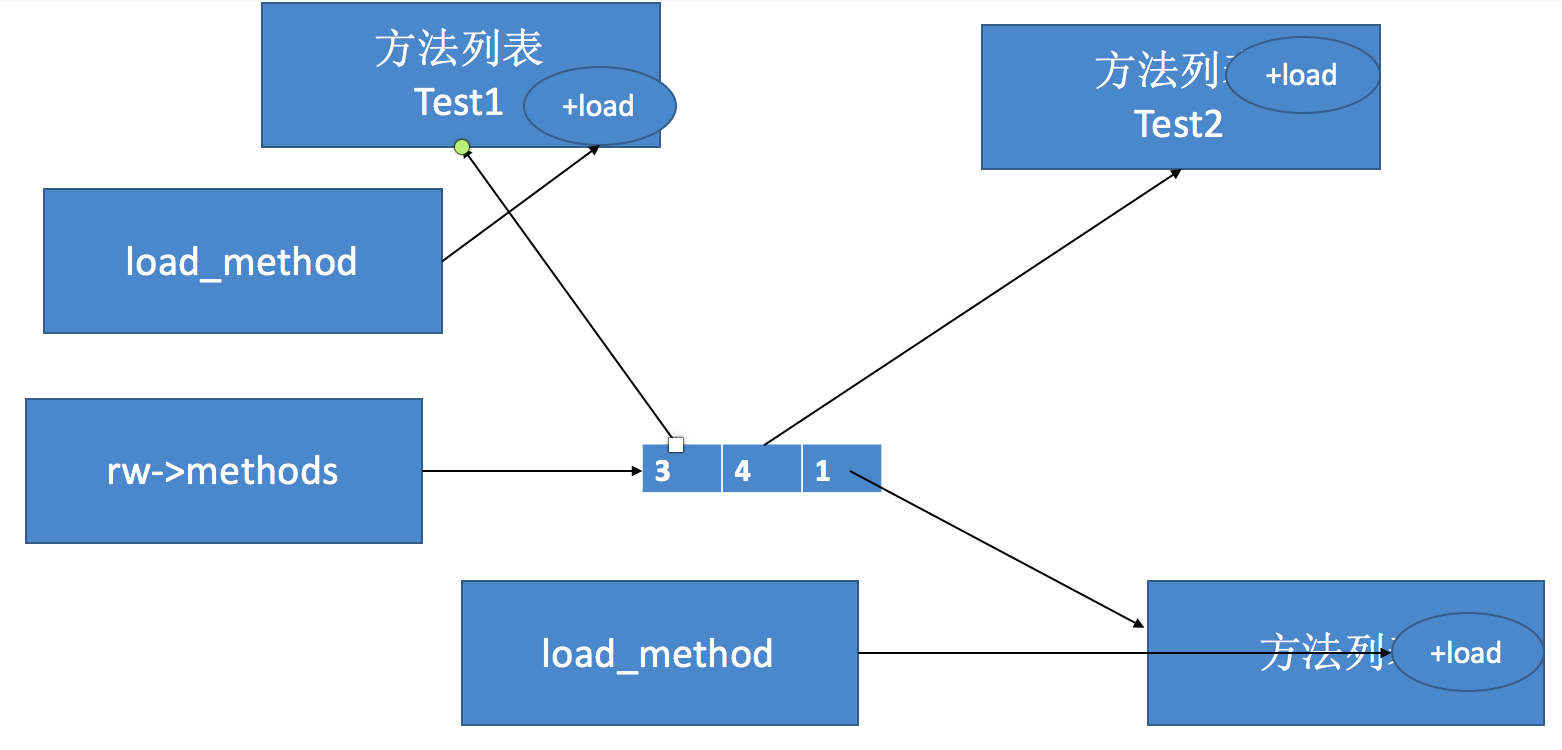
合并方法.png
二 代码佐证
2.1 从表层验证
- 代码如下
@interface CSPerson : NSObject {
- (void)run;
@end
@implementation CSPerson
- (void)run {
NSLog(@"%s",__FUNCTION__);
}
@end
#pragma mark - CSPerson (Extension)
@interface CSPerson (Extension)
- (void)run;
@end
@implementation CSPerson (Extension)
- (void)run {
NSLog(@"%s",__FUNCTION__);
}
@end
- 调用
CSStudent *stu = [[CSStudent alloc] init];
[stu run];
运行结果如下
-[CSPerson(Extension) run]
总结:当分类重写原类方法时,调用分类方法
2.2 借助 Runtime 验证
-
Student
类和Student (Extension)
类
@interface Student : NSObject
- (void)run;
@end
@implementation Student
- (void)run {
NSLog(@"%s",__FUNCTION__);
}
@end
@interface Student (Extension)
- (void)run;
@end
@implementation Student (Extension)
- (void)run {
NSLog(@"%s",__FUNCTION__);
}
@end
-
runtime
获取底层信息
- (void)getAllClassMethod {
u_int count;
Method *methods = class_copyMethodList([Student class], &count);
NSInteger index = 0;
for (int i = 0; i < count; i++) {
SEL name = method_getName(methods[i]);
IMP imp = method_getImplementation(methods[i]);
const char *encode = method_getTypeEncoding(methods[i]);
NSString *strName = [NSString stringWithCString:sel_getName(name) encoding:NSUTF8StringEncoding];
NSLog(@"%@",NSStringFromSelector(name));
NSLog(@"%s",encode);
NSLog(@"%@",strName);
NSLog(@"----------------------");
}
}
- 打印结果如下
2019-06-26 09:34:49.135221+0800 CallClassMethodNoCategory[50897:14264151] v16@0:8
2019-06-26 09:34:49.135759+0800 CallClassMethodNoCategory[50897:14264151] run
(lldb) po imp
(CallClassMethodNoCategory`-[Student(Extension) run] at Student+Extension.m:13)
2019-06-26 09:35:33.648576+0800 CallClassMethodNoCategory[50897:14264151] ----------------------
2019-06-26 09:35:33.648773+0800 CallClassMethodNoCategory[50897:14264151] v16@0:8
2019-06-26 09:35:33.648873+0800 CallClassMethodNoCategory[50897:14264151] run
(lldb) po imp
(CallClassMethodNoCategory`-[Student run] at Student.m:13)
通过
runtime
,我们可以知道,分类同名方法位于方法列表methods
的前面。
2.3 从代码底层验证
- 首先需要 MJ 写的一个类,用于窥探底层结构,
MJClassInfo.h
#import <Foundation/Foundation.h>
#ifndef MJClassInfo_h
#define MJClassInfo_h
# if __arm64__
# define ISA_MASK 0x0000000ffffffff8ULL
# elif __x86_64__
# define ISA_MASK 0x00007ffffffffff8ULL
# endif
#if __LP64__
typedef uint32_t mask_t;
#else
typedef uint16_t mask_t;
#endif
typedef uintptr_t cache_key_t;
struct bucket_t {
cache_key_t _key;
IMP _imp;
};
struct cache_t {
bucket_t *_buckets;
mask_t _mask;
mask_t _occupied;
};
struct entsize_list_tt {
uint32_t entsizeAndFlags;
uint32_t count;
};
struct method_t {
SEL name;
const char *types;
IMP imp;
};
struct method_list_t : entsize_list_tt {
method_t first;
method_t second;
method_t third;
method_t fourth;
method_t last;
};
struct ivar_t {
int32_t *offset;
const char *name;
const char *type;
uint32_t alignment_raw;
uint32_t size;
};
struct ivar_list_t : entsize_list_tt {
ivar_t first;
};
struct property_t {
const char *name;
const char *attributes;
};
struct property_list_t : entsize_list_tt {
property_t first;
};
struct chained_property_list {
chained_property_list *next;
uint32_t count;
property_t list[0];
};
typedef uintptr_t protocol_ref_t;
struct protocol_list_t {
uintptr_t count;
protocol_ref_t list[0];
};
struct class_ro_t {
uint32_t flags;
uint32_t instanceStart;
uint32_t instanceSize; // instance对象占用的内存空间
#ifdef __LP64__
uint32_t reserved;
#endif
const uint8_t * ivarLayout;
const char * name; // 类名
method_list_t * baseMethodList;
protocol_list_t * baseProtocols;
const ivar_list_t * ivars; // 成员变量列表
const uint8_t * weakIvarLayout;
property_list_t *baseProperties;
};
struct class_rw_t {
uint32_t flags;
uint32_t version;
const class_ro_t *ro;
method_list_t * methods; // 方法列表
property_list_t *properties; // 属性列表
const protocol_list_t * protocols; // 协议列表
Class firstSubclass;
Class nextSiblingClass;
char *demangledName;
};
#define FAST_DATA_MASK 0x00007ffffffffff8UL
struct class_data_bits_t {
uintptr_t bits;
public:
class_rw_t* data() {
return (class_rw_t *)(bits & FAST_DATA_MASK);
}
};
/* OC对象 */
struct mj_objc_object {
void *isa;
};
/* 类对象 */
struct mj_objc_class : mj_objc_object {
Class superclass;
cache_t cache;
class_data_bits_t bits;
public:
class_rw_t* data() {
return bits.data();
}
mj_objc_class* metaClass() {
return (mj_objc_class *)((long long)isa & ISA_MASK);
}
};
#endif /* MJClassInfo_h */
其中需要特别注意的是,获取方法列表
struct method_list_t : entsize_list_tt {
method_t first;
method_t second;
method_t third;
method_t fourth;
method_t last;
};
- 测试代码
@interface CSPerson : NSObject {
@public
int _age;
}
@property(nonatomic, assign)int no;
- (void)personInstanceMethod;
+ (void)personClassMethod;
- (void)run;
@end
@implementation CSPerson
- (void)run {
NSLog(@"%s",__FUNCTION__);
}
- (void)personInstanceMethod {
}
+ (void)personClassMethod {
}
@end
#pragma mark - CSPerson (Extension)
@interface CSPerson (Extension)
- (void)run;
@end
@implementation CSPerson (Extension)
- (void)run {
NSLog(@"%s",__FUNCTION__);
}
@end
- 调用
mj_objc_class *personClass = (__bridge mj_objc_class *)([CSPerson class]);
class_rw_t *personClassData = personClass->data();
class_rw_t *personMetaClassData = personClass->metaClass()->data();
- 运行结果如下(
打断点
)
image.png
总结:通过打印底层方法列表结构,我们可以知道,分类同名方法位于原类方法后面,所以分类方法优先调用。
推荐参考文章 iOS-底层原理(14)isa-Class的结构详解
三 如何调用原类方法
-
Student
类和Student (Extension)
类
@interface CSPerson : NSObject {
- (void)run;
@end
@implementation CSPerson
- (void)run {
NSLog(@"%s",__FUNCTION__);
}
@end
#pragma mark - CSPerson (Extension)
@interface CSPerson (Extension)
- (void)run;
@end
@implementation CSPerson (Extension)
- (void)run {
NSLog(@"%s",__FUNCTION__);
}
@end
- 使用
runtime
调用
- (void)callClassMethod {
u_int count;
Method *methods = class_copyMethodList([Student class], &count);
NSInteger index = 0;
for (int i = 0; i < count; i++) {
SEL name = method_getName(methods[i]);
NSString *strName = [NSString stringWithCString:sel_getName(name) encoding:NSUTF8StringEncoding];
if ([strName isEqualToString:@"run"]) {
index = i; // 先获取原类方法在方法列表中的索引
}
}
// 调用方法
Student *stu = [[Student alloc] init];
SEL sel = method_getName(methods[index]);
IMP imp = method_getImplementation(methods[index]);
((void (*)(id, SEL))imp)(stu,sel);
}
- 运行结果
2019-06-26 09:39:20.744704+0800 CallClassMethodNoCategory[51029:14270433] -[Student run]
总结:通过遍历类
Student
的方法列表,或许run
方法在方法列表methods
的索引,然后调用即可。