我们在开发中经常需要把时间戳转化成日期格式,但 JavaScript 本身自带的 Date 方法并不像 PHP date 的那么强大。因此,我们就需要自己动手写一个方法。
首先我们要先了解下需要用到的 JavaScript 自带的 Date 对象的方法:
Date 对象的方法 | 描述 |
---|---|
getDate | 获取一个中的某一天(1-31) |
getFullYear | 获取完整的年份,例:2019 |
getMonth | 获取月份(0-11)(ps:基数从0开始) |
getHours | 获取小时(0-23) |
getMinutes | 获取分钟(0-59) |
getSeconds | 获取秒数(0-59) |
通过上面的方法,我们就可以写出时间戳转化的方法,注意时间戳一定要精确到毫秒,因为很多后端返回的时间戳单位是秒,下面让我们看下具体写法:
/**
* 日期格式化
* @param Number time
*/
function dateFormat (time) {
const t = new Date(time)
// 日期格式
const format = 'Y-m-d h:i:s'
const year = t.getFullYear()
// 由于 getMonth 返回值会比正常月份小 1
const month = t.getMonth() + 1
const day = t.getDate()
const hours = t.getHours()
const minutes = t.getMinutes()
const seconds = t.getSeconds()
const hash = {
'Y': year,
'm': month,
'd': day,
'h': hours,
'i': minutes,
's': seconds
}
return format.replace(/\w/g, o => {
return hash[o]
})
}
通过上面的方法,dateFormat(1558259949034) 返回的结果是 2019-5-19 17:59:9,如果我们想要的结果是小于 10 的数字前面自动补 0: 2019-05-19 17:59:09,写法可以改进下:
/**
* 日期格式化
* @param Number time
*/
function dateFormat (time) {
const t = new Date(time)
// 日期格式
const format = 'Y-m-d h:i:s'
let year = t.getFullYear()
// 由于 getMonth 返回值会比正常月份小 1
let month = t.getMonth() + 1
let day = t.getDate()
let hours = t.getHours()
let minutes = t.getMinutes()
let seconds = t.getSeconds()
month = month > 9 ? month : `0${month}`
day = day > 9 ? day : `0${day}`
hours = hours > 9 ? hours : `0${hours}`
minutes = minutes > 9 ? minutes : `0${minutes}`
seconds = seconds > 9 ? seconds : `0${seconds}`
const hash = {
'Y': year,
'm': month,
'd': day,
'h': hours,
'i': minutes,
's': seconds
}
return format.replace(/\w/g, o => {
return hash[o]
})
}
但上面的方法还不够灵活,比如,我可能只想要日期,或者不显示年,或者小于 10 的数字前面不补上 0 等等,我们约定,字母大写小于 10 的数字就补上 0 :
/**
* 日期格式化
* @param Number time 时间戳
* @param String format 格式
*/
function dateFormat (time, format) {
const t = new Date(time)
// 日期格式
format = format || 'Y-m-d h:i:s'
let year = t.getFullYear()
// 由于 getMonth 返回值会比正常月份小 1
let month = t.getMonth() + 1
let day = t.getDate()
let hours = t.getHours()
let minutes = t.getMinutes()
let seconds = t.getSeconds()
const hash = {
'y': year,
'm': month,
'd': day,
'h': hours,
'i': minutes,
's': seconds
}
// 是否补 0
const isAddZero = (o) => {
return /M|D|H|I|S/.test(o)
}
return format.replace(/\w/g, o => {
let rt = hash[o.toLocaleLowerCase()]
return rt > 10 || !isAddZero(o) ? rt : `0${rt}`
})
}
dateFormat(1558259949034, 'Y-M-D H:I:S') 返回结果是 2019-05-19 17:59:09。
完结,撒花。如果你有更好的写法,欢迎留言交流。
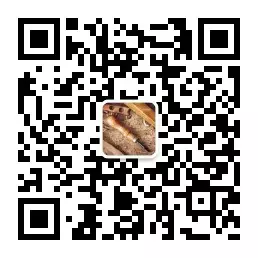