背景
在之前,从来都是后端返一个图片地址,然后前端直接使用。 那如果后端返了一个图片文件过来,那要怎么做?
参考:
正文
这次我接收到的直接是一个图片文件,然后乱码了。。 如下图
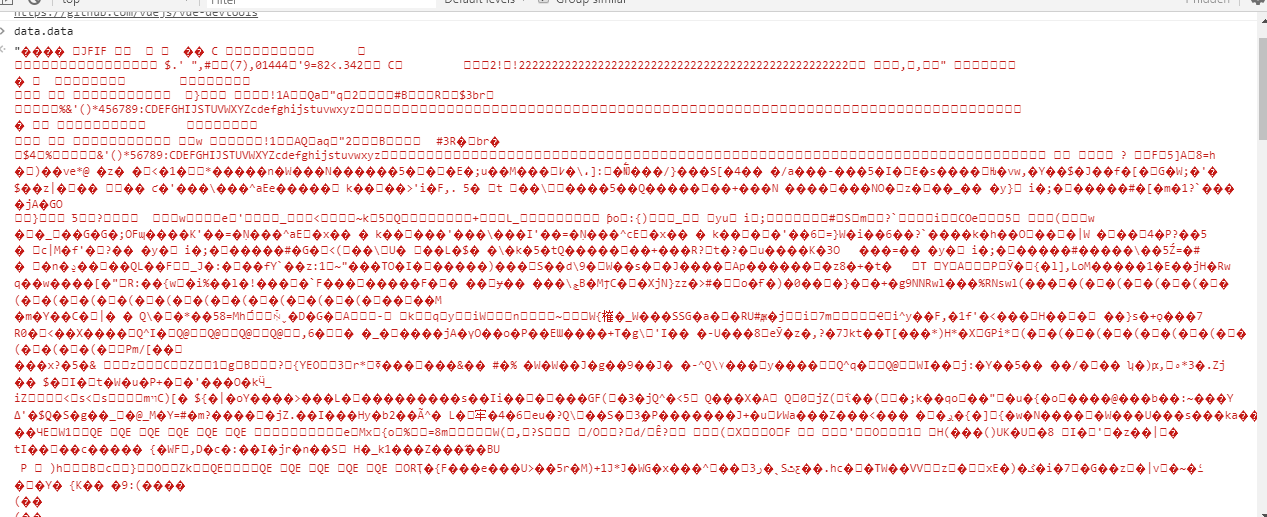
要怎么处理呢?
首先是第一步,请求处理,下面我分别列出了axios、fetch、jq、原生js的处理方式
1.修改请求
1.1. axios
重点在于添加option: responseType: 'arraybuffer'
let url = `imgurl`;
axios.get(url,{responseType: 'arraybuffer'}).then(function(data){
console.log(data);
}).catch(function(err){
error(err)
});
1.2. fetch
我们在使用fetch的时候,会有一个response的对象处理过程。
普遍我们都是使用的 response.json()
,但这次我们需要进行response.arrayBuffer()
处理
fetch(url)
.then(function(response) {
if(response.ok) {
return response.arrayBuffer(); // 这一行是关键
}
throw new Error('Network response was not ok.');
})
.then(function(data) {
console.log(data)
})
.catch(function(error) {
});
1.3. JQ-ajax
如果使用jq封装的ajax,那么抱歉,这个功能用不了。
jq封装的ajax是基于xhr第一版本的,而ArrayBuffer是基于xhr第二版本的
这种情况就推荐使用原生js来写
1.4. 原生js
let xhr = new XMLHttpRequest();
xhr.open('GET', url, true);
xhr.responseType = 'arraybuffer'; // 重点
let that = this; // 这个不是必须,只是用来保存this的指向
xhr.onload = function(e) {
if (this.status == 200) {
let result = this.response;
console.log(result)
}
};
xhr.send();
补充知识点
responseType设置的是啥? arraybuffer又是啥?
这些内容估计又可以开一篇文章来写了,详细的可以参考以下网址:
MDN-XMLHttpRequest.responseType
2.处理返回值
好了,我们现在已经获取到了我们需要的二进制数组(arrayBuffer) 下一步,我们要把图片显示处理,使用base64编码即可
let imgUrl = 'data:image/png;base64,' + btoa(new Uint8Array(data.data).reduce((data, byte) => data + String.fromCharCode(byte), ''))
// 这里如果不清楚 new Uint8Array(data.data) 中data的指代,就看看最上面的那个图
这样能将图片进行编码,然后做前端缓存(如果用localStorage缓存blob,存能成功,但是刷新后再取出来是看不见图片的)。
解释一下各自含义:
data: ----获取数据类型名称
image/png; -----指数据类型名称
base64 -----指编码模式
AAAAA ------指编码以后的结果。
3.使用
2种方法:
- 如果使用vue这类框架,可以直接style赋值
- 创建动态style,创建动态css
第一个这个使用就不说了,直接src=imgUrl
;
//vue
<img :src="imgUrl">
第二个我们要做的就是创建style节点,并添加在head种
if(document.all){ // document.createStyleSheet(url)
window.style=`.imga{background-img: url(${imgUrl});`;
document.createStyleSheet("javascript:style");
}else{ //document.createElement(style)
var style = document.createElement('style');
style.type = 'text/css';
style.innerHTML=`.imga{background-img: url(${imgUrl});`;
document.getElementsByTagName('HEAD').item(0).appendChild(style);
}
这样,就动态创建了相应的style样式。 然后给需要的地方添加上 .img 即可
...
<div class="imga">
...
</div
...