阅读目录
深入跨域问题(4) - 利用 代理服务器 解决跨域(本篇)
在上面的文章中,我们依此了解到,CORS
,JSONP
两种方式实现跨域请求。
这两种方法,都需要 前后端进行配合 才能够正确地处理跨域问题。 今天介绍一种方法,不需要前后端配合,前端可独立完成。
众所周知,同源策略是浏览器需要遵循的标准,重点是 浏览器 ,如果,是服务器是不是就不用遵守了呢 ???
NODE 作为客户端
这是,我们的一个猜想,非浏览器之间,不用遵行同源策略,先来测试一下:
NODE 发送 POST 方法
客户端:
const http = require('http');
const options = {
hostname: '127.0.0.1', // 注意这里不要添加 http 前缀
port: 4000, // 服务器端口号
path: '/', // 访问路径
method: 'POST',
headers: {
'Content-Type': 'application/json;charset=utf-8',
}
};
const request = http.request(options, (serverResponse) => {
var body = '';
serverResponse.on('data', (chunk) => {
body += chunk;
});
serverResponse.on('end', () => {
console.log('The data is ' + body);
})
});
request.end();
服务器,发起一个POST请求
PS: 在浏览器中 POST 方法下 Content-Type
为 application/json;
,会触发 预请求 (请看我之前的文章)。
服务器:
const http = require('http');
const data = { name: 'BruceLee', password: '123456' };
const server = http.createServer((request, response) => {
if (request.url === '/') {
response.end( JSON.stringify(data) );
}
});
server.listen(4000, () => {
console.log('The server is running at http://localhost:4000');
});
注意:我们没有在服务器内部,加入任何与 CORS 跨域资源共享的首部字段 。
实验结果:
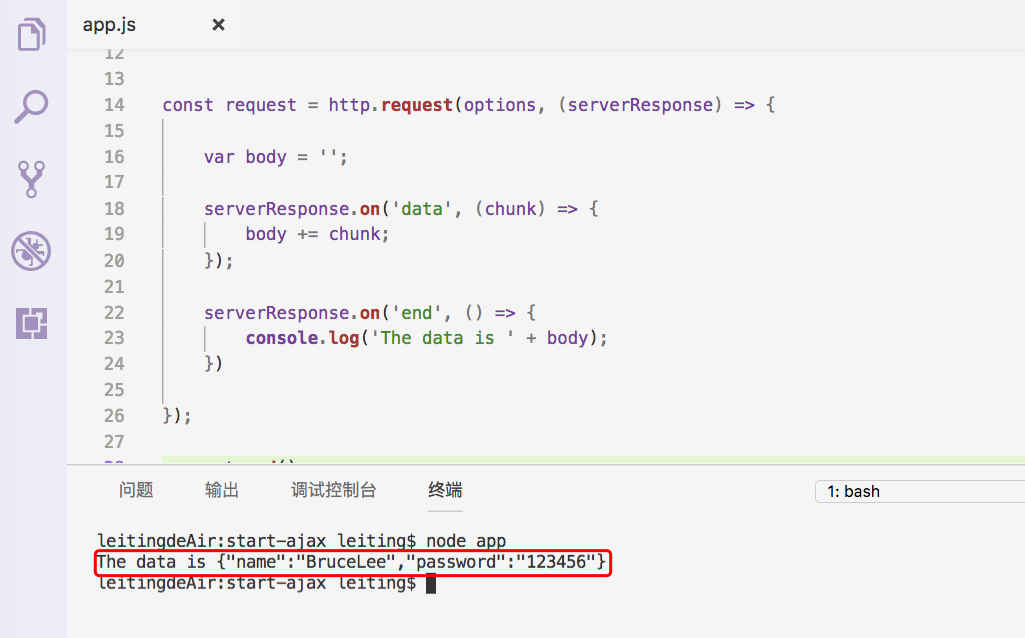
测试结果表明,通过 NODE
发起请求可以避免 浏览器 的同源策略。
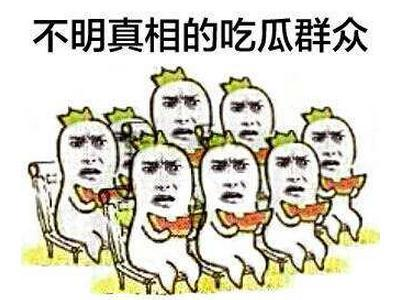
代理服务器
代理服务器,需要做以下几个步骤:
- 接受客户端 请求 。
- 将 请求 转发给服务器。
- 拿到服务器 响应 数据。
- 将 响应 转发给客户端。
这是简单的文字解析,下方为图解流程:
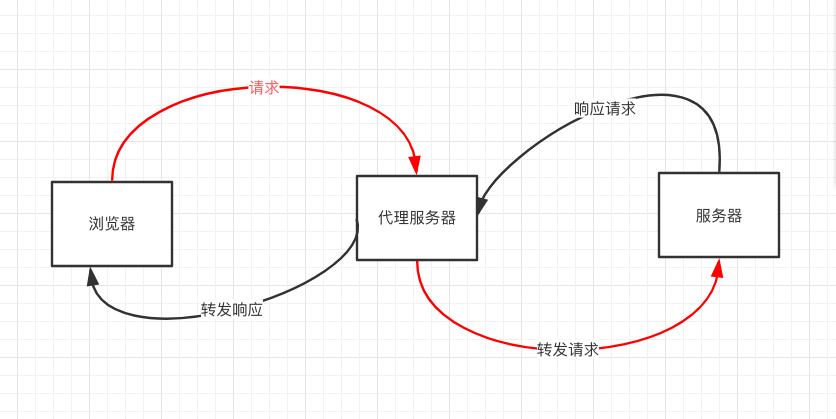
这就是,代理服务器的工作机制(PS:画的垃圾,哈哈哈)。
实现代理服务器
在了解,代理服务器的工作机制之后,下面我们将用 NODE 实现代理服务器。
客户端:
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Ajax测试</title>
</head>
<body>
<script src="./node_modules/jquery/dist/jquery.min.js"></script>
<script>
var data = { name: 'BruceLee', password: '123456' };
$.ajax({
url: "http://localhost:3000",
type: "post",
data: JSON.stringify(data),
contentType: 'application/json;charset=utf-8',
success: function (result) {
console.log(result);
},
error: function (msg) {
console.log(msg);
}
})
</script>
</body>
</html>
同样,你可以换成,PUT,DELETE 等方法,进行测试。
代理服务器:3000 端口
const http = require('http');
// 第一步:接受客户端请求
const server = http.createServer((request, response) => {
// 代理服务器,直接和浏览器直接交互,也需要设置:CORS 的首部字段
response.writeHead(200, {
'Access-Control-Allow-Origin': '*', // 设置 optins 方法允许所有服务器访问
'Access-Control-Allow-Methods': '*',
'Access-Control-Allow-Headers': 'Content-Type',
});
// 第二步:将请求转发给服务器
const proxyRequest = http.request({
host: '127.0.0.1',
port: 4000,
url: '/',
method: request.method,
headers: request.headers
}, (serverResponse) => {
// 第三步:收到服务器的响应
var body = '';
serverResponse.on('data', (chunk) => {
body += chunk;
});
serverResponse.on('end', () => {
console.log('The data is ' + body );
// 第四步:将响应结果转发给浏览器
response.end(body);
})
}).end();
});
server.listen(3000, () => {
console.log('The proxyServer is running at http://localhost:3000');
});
需要我们注意的是:代理服务器,直接与浏览器相连接,也必须需要遵守 浏览器的同源策略,
服务器:4000 端口
const http = require('http');
const data = { name: 'BruceLee', password: '123456' };
const server = http.createServer((request, response) => {
if (request.url === '/') {
response.end( JSON.stringify(data) );
}
});
server.listen(4000, () => {
console.log('The server is running at http://localhost:4000');
});
为了篇幅仍然较小,我采用了较为简洁的代码,你可以自己多试试 比如:PUT,DELETE 方法。
实验结果:
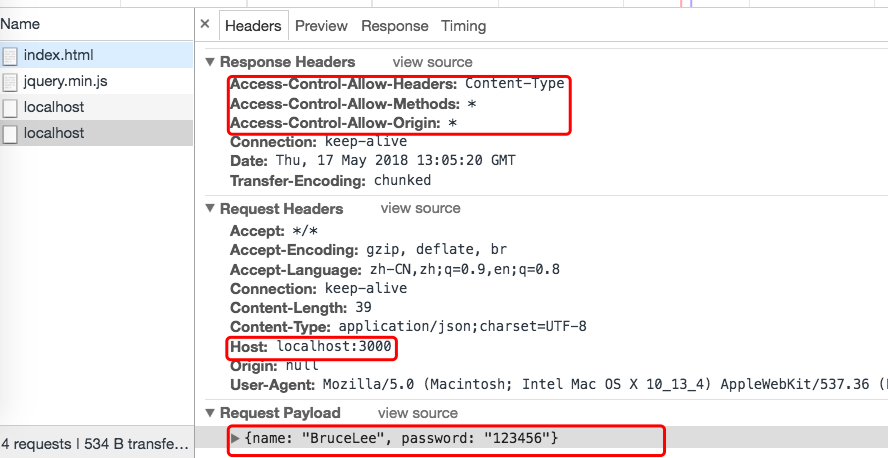
成功避免了跨域请求。
现在,我们已经完成了,代理服务器解决跨域问题了,并且不需要后台做任何设置。
参考与鸣谢
- NODE 官方文档
- https://blog.csdn.net/real_bird/article/details/52714535
代理服务器是现代化的前端常用的手段,另外还有反向代理,等等众多方式实现跨域请求。