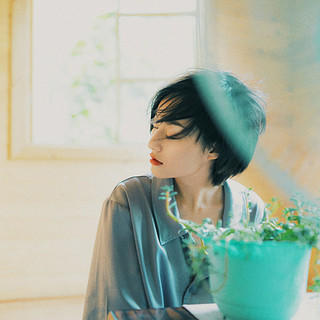
用惯了MVC模式,是不是觉得ViewContro'l'le'r层的东西太多了,太乱了,随随便便就几百行代码,现在给大家带来一个在Android软件开发中最常使用的MVP面向协议编程模式,我也是觉得这种模式好用,才将这种思想转移过来的,设计模式不是指单纯的应用在某一种语言当中,他可以试用任何一种开发语言,所以好用的东西就要分享给大家,为了便于大家理解,Demo只是最基本的完成了MVP的架构模式,感兴趣的可以下来看一看。
MVP 模式
Model-View-Presenter(MVP)是(MVC)体系结构模式的一种变体,并主要用于构建用户界面。在iOS,这个模式是使用一个协议实现的,协议定义了接口实现的委托。
Presenter
在MVP模式中,协议是假定中间人的功能,所有表示逻辑被推到中间人中。
Controller v/s Presenter
-
V层
UIView和UIViewController以及子类
-
P层
中介(关联M和V)
-
M层
数据层(数据:数据库,网络,文件等等)
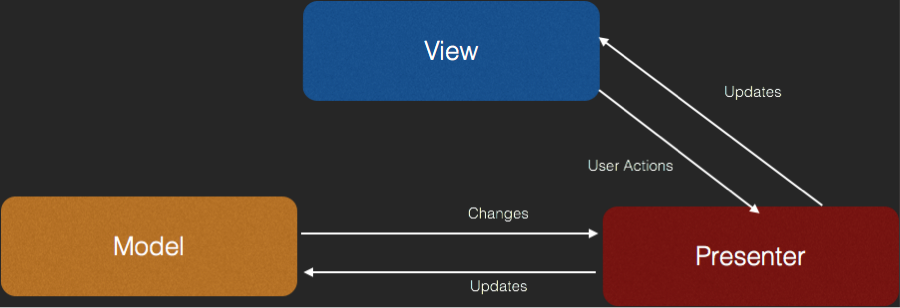
mvp.png
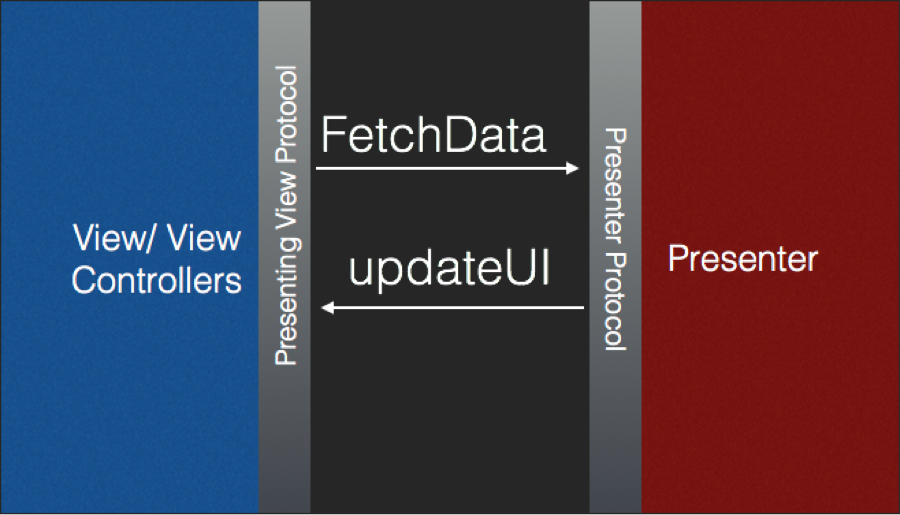
mvp-delegate.png
第一步:实现M层
#import "LoginModel.h"
//M层
@implementation LoginModel
- (void)loginWithName:(NSString*)name pwd:(NSString*)pwd callback:(Callback)callback{
//实现功能
//例如:访问网络?访问数据库?
//数据曾划分了模块()
[HttpUtils postWithName:name pwd:pwd callback:^(NSString *result) {
//解析json ,xml数据
//然后保存数据库
//中间省略100行代码
callback(result);//返回数据回调
}];
}
@end
第二步:实现V层
#import <Foundation/Foundation.h>
//V层
@protocol LoginView <NSObject>
- (void)onLoginResult:(NSString*)result;
@end
第三步:实现P层
// P层
#import <Foundation/Foundation.h>
#import "LoginView.h"
#import "LoginModel.h"
//中介(用于关联M层和V层)
@interface LoginPresenter : NSObject
//提供一个业务方法
- (void)loginWithName:(NSString*)name pwd:(NSString*)pwd;
- (void)attachView:(id<LoginView>)loginView;
- (void)detachView;
@end
#import "LoginPresenter.h"
//P是中介(职责是用于关联M和V)
//P层需要:持有M层的引用和V层的引用(OOP)思想
@interface LoginPresenter ()
@property (nonatomic,strong) LoginModel *loginModel;
@property (nonatomic,strong) id<LoginView> loginView;
@end
@implementation LoginPresenter
- (instancetype)init{
self = [super init];
if (self) {
//持有M层的引用
_loginModel = [[LoginModel alloc]init];
}
return self;
}
//提供绑定V层方法
//绑定
- (void)attachView:(id<LoginView>)loginView{
_loginView = loginView;
}
//解除绑定
- (void)detachView{
_loginView = nil;
}
//实现业务方法
- (void)loginWithName:(NSString*)name pwd:(NSString*)pwd{
[_loginModel loginWithName:name pwd:pwd callback:^(NSString *result) {
if (_loginView != nil) {
[_loginView onLoginResult:result];
}
}];
}
@end
第四步:在VIewController中使用
#import "ViewController.h"
#import "LoginView.h"
#import "LoginPresenter.h"
@interface ViewController ()<LoginView>
@property (nonatomic,strong) LoginPresenter* presenter;
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
_presenter = [[LoginPresenter alloc ]init];
[_presenter attachView:self];
//程序一旦运行立马执行请求(测试)(按钮或者事件)
[_presenter loginWithName:@"188*****8*8" pwd:@"123456"];
}
- (void)onLoginResult:(NSString *)result{
NSLog(@"返回结果%@",result);
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
[_presenter detachView];
}
@end
这样一个简单的MVP模式的逻辑就完成了,这里有宝藏Demo,如果觉得有帮助,不要忘记点个star哦!